This article explains MongoDB's advanced query operators, enabling complex data filtering beyond simple equality checks. It details operators like $eq, $ne, $gt, $in, $regex, and their combinations for efficient data retrieval, aggregation, and vali
How to Use MongoDB's Query Operators for Advanced Filtering?
MongoDB offers a rich set of query operators that go beyond simple equality checks, enabling powerful and flexible data filtering. These operators allow you to specify complex criteria for selecting documents from your collections. Here's a breakdown of how to use them:
1. Understanding the Basic Syntax: MongoDB queries use a JSON-like structure. The core element is a query document that contains key-value pairs. The keys represent the fields you want to filter, and the values specify the conditions.
2. Essential Operators:
-
$eq
(Equality): Matches documents where the field value is equal to the specified value. e.g.,{"age": {"$eq": 30}}
-
$ne
(Not Equal): Matches documents where the field value is not equal to the specified value. e.g.,{"city": {"$ne": "New York"}}
-
$gt
,$gte
,$lt
,$lte
(Comparison Operators): Greater than, greater than or equal to, less than, less than or equal to. e.g.,{"price": {"$gt": 100}}
-
$in
,$nin
(Inclusion/Exclusion): Matches documents where the field value is in (or not in) an array of values. e.g.,{"status": {"$in": ["active", "pending"]}}
-
$regex
(Regular Expressions): Matches documents where the field value matches a regular expression pattern. e.g.,{"name": {"$regex": /^John/}}
(matches names starting with "John") -
$exists
: Checks if a field exists in a document. e.g.,{"address": {"$exists": true}}
-
$type
: Matches documents based on the BSON type of a field. Useful for data validation.
3. Combining Operators: You can combine multiple operators within a single query document to create complex filtering logic. MongoDB will apply these conditions conjunctively (using AND). For OR conditions, use the $or
operator:
db.collection.find( { $or: [ { age: { $gt: 30 } }, { city: "London" } ] } )
4. Using the MongoDB Shell or Driver: These operators are used within the find()
method of your chosen MongoDB driver (e.g., pymongo for Python, the MongoDB shell).
What Are Some Common Use Cases for MongoDB's Advanced Query Operators?
MongoDB's advanced query operators are crucial for a wide variety of data filtering and manipulation tasks. Here are some common use cases:
- Targeted Data Retrieval: Quickly find specific documents based on complex criteria, such as finding all users with an age between 25 and 35 who live in a particular city and have a specific subscription status. This avoids retrieving and processing the entire dataset.
-
Data Aggregation and Analysis: Advanced operators are essential for building aggregation pipelines. For instance, you can use
$match
(to filter data) with$group
(to group documents) and$sum
(to perform calculations) to analyze sales data by region or product. -
Implementing Business Rules: Enforce business logic within your queries. For example, you might use
$regex
to validate email addresses or$type
to ensure data integrity. - Real-time Filtering and Search: In applications with dynamic filtering needs, like e-commerce sites or search engines, advanced operators provide the flexibility to refine search results based on user input.
-
Data Validation and Cleaning: Identify and correct inconsistent or erroneous data within your collection. For example, you can use
$exists
to find documents missing critical fields.
Can I Use MongoDB's Query Operators to Filter Data Based on Nested Documents?
Yes, MongoDB's query operators work seamlessly with nested documents. To filter based on fields within nested documents, you use dot notation to specify the path to the nested field.
For example, consider documents with the structure:
{ "user": { "name": "Alice", "address": { "city": "New York", "zip": "10001" } } }
To find all documents where the city is "New York," you would use:
db.collection.find( { "user.address.city": "New York" } )
For more complex nested filtering, you can combine dot notation with other operators:
db.collection.find( { "user.address.zip": { $regex: /^100/ } } ) // Find documents where zip code starts with "100"
You can also use the $elemMatch
operator to filter arrays of embedded documents. This allows you to specify conditions that must be met by at least one element within the array.
How Do I Optimize My MongoDB Queries Using Advanced Operators for Better Performance?
Optimizing MongoDB queries using advanced operators involves several strategies:
-
Indexing: Proper indexing is paramount. Create indexes on fields frequently used in
$match
stages of aggregations orfind()
queries. Compound indexes can speed up queries involving multiple fields. -
Selective Field Retrieval: Use the
projection
parameter in yourfind()
queries to retrieve only the necessary fields. This reduces the amount of data transferred from the database, improving performance. -
Avoid
$or
with Unindexed Fields: Queries using$or
can be slow if the fields involved are not indexed. Consider alternative approaches, such as multiple queries or creating separate indexes. -
Limit Data Returned: Use the
limit()
method to restrict the number of documents returned. This is particularly important for large datasets. -
Efficient Operator Usage: Choose the most appropriate operator for the task. For example, using
$in
with a small array is generally more efficient than multiple$or
conditions. -
Analyze Query Execution Plans: Use
explain()
to analyze the execution plan of your queries. This helps identify bottlenecks and areas for optimization. Theexplain()
output shows the index used (or lack thereof), the number of documents examined, and other performance metrics. -
Aggregation Pipeline Optimization: When using aggregation pipelines, try to minimize the number of stages and ensure that each stage efficiently processes the data. Consider using
$lookup
for joins instead of multiple stages when possible.
By carefully selecting and using advanced operators, and by optimizing your queries through indexing and efficient data retrieval, you can significantly improve the performance of your MongoDB applications.
The above is the detailed content of How do I use MongoDB's query operators for advanced filtering?. For more information, please follow other related articles on the PHP Chinese website!
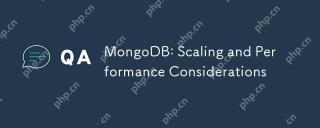
MongoDB's scalability and performance considerations include horizontal scaling, vertical scaling, and performance optimization. 1. Horizontal expansion is achieved through sharding technology to improve system capacity. 2. Vertical expansion improves performance by increasing hardware resources. 3. Performance optimization is achieved through rational design of indexes and optimized query strategies.
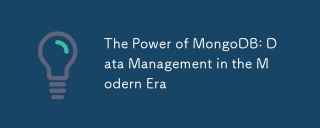
MongoDB is a NoSQL database because of its flexibility and scalability are very important in modern data management. It uses document storage, is suitable for processing large-scale, variable data, and provides powerful query and indexing capabilities.
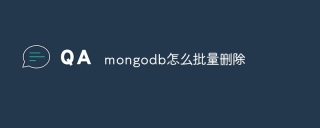
You can use the following methods to delete documents in MongoDB: 1. The $in operator specifies the list of documents to be deleted; 2. The regular expression matches documents that meet the criteria; 3. The $exists operator deletes documents with the specified fields; 4. The find() and remove() methods first get and then delete the document. Please note that these operations cannot use transactions and may delete all matching documents, so be careful when using them.

To set up a MongoDB database, you can use the command line (use and db.createCollection()) or the mongo shell (mongo, use and db.createCollection()). Other setting options include viewing database (show dbs), viewing collections (show collections), deleting database (db.dropDatabase()), deleting collections (db.<collection_name>.drop()), inserting documents (db.<collecti

Deploying a MongoDB cluster is divided into five steps: deploying the primary node, deploying the secondary node, adding the secondary node, configuring replication, and verifying the cluster. Including installing MongoDB software, creating data directories, starting MongoDB instances, initializing replication sets, adding secondary nodes, enabling replica set features, configuring voting rights, and verifying cluster status and data replication.

MongoDB is widely used in the following scenarios: Document storage: manages structured and unstructured data such as user information, content, product catalogs, etc. Real-time analysis: Quickly query and analyze real-time data such as logs, monitoring dashboard displays, etc. Social Media: Manage user relationship maps, activity streams, and messaging. Internet of Things: Process massive time series data such as device monitoring, data collection and remote management. Mobile applications: As a backend database, synchronize mobile device data, provide offline storage, etc. Other areas: diversified scenarios such as e-commerce, healthcare, financial services and game development.

How to view MongoDB version: Command line: Use the db.version() command. Programming language driver: Python: print(client.server_info()["version"])Node.js: db.command({ version: 1 }, (err, result) => { console.log(result.version); });
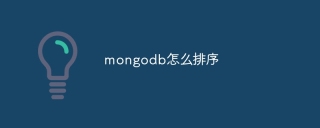
MongoDB provides a sorting mechanism to sort collections by specific fields, using the syntax db.collection.find().sort({ field: order }) ascending/descending order, supports compound sorting by multiple fields, and recommends creating indexes to improve sorting performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
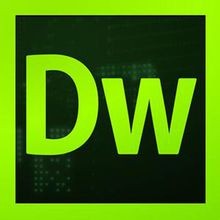
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
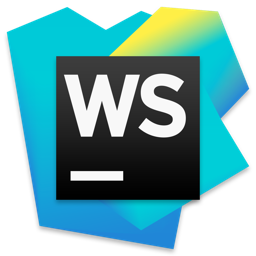
WebStorm Mac version
Useful JavaScript development tools