This article details creating custom Nginx modules, covering development environment setup, module structure, directive definition, handler implementation, registration, compilation, testing, and deployment. It emphasizes avoiding memory leaks, ensu
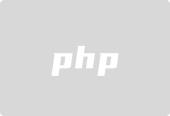
How to Implement Custom Nginx Modules for Specific Workloads?
Implementing custom Nginx modules requires a solid understanding of C programming and the Nginx architecture. The process generally involves several key steps:
1. Setting up the Development Environment: You'll need the Nginx source code, a C compiler (like GCC), and the necessary development libraries. Ensure you have the libpcre
(for regular expressions) and zlib
(for compression) libraries installed. A build system like autotools
(autoconf, automake, libtool) is commonly used.
2. Creating the Module Structure: A basic Nginx module consists of several files:
-
ngx_http_mymodule_module.c
: This is the core file containing the module's logic. It defines the module's directives, handlers, and other functions.
-
config
(optional): A configuration file to manage module-specific settings.
3. Defining Module Directives: These are configurations you'll define within your Nginx configuration file (nginx.conf
) to control the module's behavior. You'll use Nginx's API to parse and validate these directives.
4. Implementing Handlers: Handlers are functions that are executed at specific stages in the Nginx request processing cycle. Common handlers include ngx_http_handler
(for processing requests) and ngx_http_exit_handler
(for cleanup).
5. Registering the Module: This involves registering the module's directives and handlers with Nginx using the appropriate API functions.
6. Compiling and Installing: Use the Nginx build system to compile your module and then install it into the Nginx installation directory.
7. Testing and Debugging: Thoroughly test your module with various scenarios and use Nginx's logging capabilities to identify and fix any bugs.
What are the common pitfalls to avoid when developing custom Nginx modules?
Developing custom Nginx modules can be challenging. Here are some common pitfalls to avoid:
-
Memory Leaks: Nginx is highly sensitive to memory leaks. Always ensure you free allocated memory using
ngx_palloc
and ngx_pfree
. Use memory debugging tools to identify and fix leaks.
-
Incorrect Error Handling: Proper error handling is crucial. Always check the return values of Nginx API functions and handle errors gracefully. Avoid crashing the entire Nginx process due to an error in your module.
-
Ignoring Thread Safety: Nginx is multi-threaded. Your module must be thread-safe to prevent race conditions and data corruption. Use appropriate synchronization mechanisms (mutexes, atomic operations) when accessing shared resources.
-
Ignoring Nginx's Event Loop: Avoid blocking operations within your module's handlers. Blocking the event loop can lead to performance degradation and unresponsiveness. Use asynchronous operations or offload long-running tasks to external processes.
-
Insufficient Testing: Thorough testing is paramount. Test your module with different request patterns, configurations, and load levels. Use automated testing frameworks to streamline the process.
-
Ignoring Security Best Practices: Secure coding practices are essential. Sanitize user inputs to prevent vulnerabilities like SQL injection or cross-site scripting (XSS).
How can I ensure my custom Nginx module integrates seamlessly with existing infrastructure?
Seamless integration with existing infrastructure requires careful planning and adherence to best practices:
-
Configuration Compatibility: Design your module's configuration directives to be compatible with existing Nginx configurations and avoid conflicts with other modules.
-
Logging and Monitoring: Integrate your module's logging with existing monitoring systems. Use standard log formats and provide meaningful log messages.
-
API Consistency: Adhere to Nginx's API conventions to ensure compatibility and maintainability.
-
Version Control: Use a version control system (like Git) to manage your module's code and track changes.
-
Documentation: Provide clear and concise documentation for your module, including installation instructions, configuration options, and usage examples.
-
Deployment Strategy: Develop a robust deployment strategy that ensures smooth updates and rollbacks. Consider using a configuration management tool like Ansible or Puppet.
What performance optimizations are crucial for custom Nginx modules handling high-traffic workloads?
Performance optimization is critical for custom Nginx modules handling high-traffic workloads:
-
Minimize Memory Allocation: Avoid excessive memory allocations within the request processing path. Reuse memory buffers whenever possible.
-
Use Efficient Algorithms and Data Structures: Choose algorithms and data structures optimized for performance. Consider using hash tables for fast lookups.
-
Avoid Blocking Operations: As mentioned before, avoid blocking operations in your handlers. Use asynchronous I/O or offload tasks to external processes.
-
Optimize String Manipulation: String manipulation can be expensive. Use efficient string functions and avoid unnecessary string copies.
-
Caching: Implement caching mechanisms to reduce the number of expensive operations. Use Nginx's built-in caching features or create your own caching layer.
-
Profiling and Benchmarking: Use profiling tools to identify performance bottlenecks and benchmark your module under realistic load conditions. This will help you target your optimization efforts effectively.
-
Asynchronous Operations: Leverage Nginx's asynchronous capabilities whenever possible to avoid blocking the event loop. Use
ngx_http_postpone_event
and other asynchronous mechanisms to handle long-running tasks without impacting performance.
Remember that thorough testing and profiling are crucial throughout the development process to ensure your custom Nginx module performs optimally under high-traffic conditions.
The above is the detailed content of How to Implement Custom Nginx Modules for Specific Workloads?. For more information, please follow other related articles on the PHP Chinese website!