This article details implementing asynchronous task processing in PHP using Swoole. It explains how Swoole's Coroutine\parallel enables concurrent task execution, improving performance by preventing blocking. The article addresses error handling an
How to Implement Asynchronous Task Processing Using Swoole in PHP?
Implementing asynchronous task processing with Swoole in PHP involves leveraging its asynchronous capabilities to handle tasks concurrently without blocking the main thread. This allows your application to remain responsive while performing long-running operations in the background. Here's a breakdown of the process:
First, you need to install the Swoole extension. This usually involves compiling it from source or using a pre-built package depending on your operating system and PHP version. Once installed, you can start using Swoole's asynchronous features.
The core component for asynchronous task processing is Swoole\Coroutine\parallel
. This allows you to run multiple coroutines concurrently. A coroutine is a lightweight thread, allowing for efficient concurrency management. Here's a simple example:
<?php use Swoole\Coroutine; $tasks = [ function () { // Simulate a long-running task Coroutine::sleep(2); return "Task 1 completed"; }, function () { // Simulate another long-running task Coroutine::sleep(1); return "Task 2 completed"; }, function () { // Simulate a task that might fail Coroutine::sleep(3); throw new Exception("Task 3 failed!"); } ]; $results = Coroutine\parallel($tasks); foreach ($results as $index => $result) { if ($result instanceof \Swoole\Coroutine\Parallel\Result) { if ($result->hasError()) { echo "Task " . ($index 1) . " failed: " . $result->getError()->getMessage() . "\n"; } else { echo "Task " . ($index 1) . " completed: " . $result->getData() . "\n"; } } } ?>
This code defines three tasks, each simulating a long-running operation using Coroutine::sleep()
. Coroutine\parallel()
executes them concurrently, and the results are handled individually, demonstrating error handling (which we'll expand upon later). Remember to handle potential exceptions within each task function. For more complex scenarios, consider using Swoole's task workers for better scalability and management of asynchronous operations.
What are the performance benefits of using Swoole for asynchronous tasks in PHP compared to traditional methods?
Traditional PHP, using synchronous methods, handles requests sequentially. This means each request waits for the previous one to finish before starting. With long-running tasks, this leads to significant performance bottlenecks and reduced responsiveness. Swoole, on the other hand, offers substantial performance improvements through its asynchronous, event-driven architecture:
- Concurrency: Swoole handles multiple requests concurrently using a non-blocking I/O model. This drastically reduces wait times and increases throughput, especially under high load.
- Reduced Latency: Asynchronous operations don't block the main thread. This means your application remains responsive even while processing long-running tasks. Users experience shorter wait times and improved user experience.
- Resource Efficiency: Swoole's lightweight coroutines consume far fewer resources than traditional threads. This allows you to handle more concurrent tasks with the same server resources.
- Scalability: Swoole's asynchronous nature makes it highly scalable. You can efficiently handle a larger number of concurrent requests compared to traditional PHP applications.
In short, Swoole provides significant performance gains by eliminating blocking operations and enabling concurrent task processing, resulting in faster response times, improved resource utilization, and enhanced scalability.
How can I handle errors and exceptions effectively in asynchronous Swoole tasks within a PHP application?
Error and exception handling in asynchronous Swoole tasks is crucial for maintaining application stability and providing informative error messages. The Swoole\Coroutine\parallel
function, as shown in the previous example, provides a mechanism for handling exceptions from individual tasks. The Result
object returned by parallel
indicates whether a task completed successfully or encountered an error.
Here's a more robust example demonstrating error handling:
<?php use Swoole\Coroutine; // ... (task definitions as before) ... try { $results = Coroutine\parallel($tasks); foreach ($results as $index => $result) { if ($result->hasError()) { $error = $result->getError(); // Log the error using a proper logging mechanism error_log("Task " . ($index 1) . " failed: " . $error->getMessage() . " Trace: " . $error->getTraceAsString()); // Optionally, retry the failed task or take other corrective actions. } else { // Process the successful result echo "Task " . ($index 1) . " completed: " . $result->getData() . "\n"; } } } catch (Exception $e) { // Handle exceptions that occur outside of individual tasks error_log("Global exception caught: " . $e->getMessage() . " Trace: " . $e->getTraceAsString()); } ?>
This improved example includes:
-
Error Logging: Error messages are logged using
error_log()
, which should be replaced with a more sophisticated logging solution in a production environment (e.g., Monolog). Including the stack trace provides valuable debugging information. -
Global Exception Handling: A
try-catch
block surrounds theCoroutine\parallel
call to handle exceptions that might occur outside individual tasks. - Retry Mechanism (Optional): The commented-out section suggests the possibility of implementing a retry mechanism for failed tasks.
Remember to choose an appropriate error handling strategy based on your application's requirements. Consider factors like retry policies, alerting mechanisms, and error reporting to external services.
What are some common use cases for implementing asynchronous task processing with Swoole in a PHP project?
Swoole's asynchronous capabilities are well-suited for a wide range of tasks in PHP applications. Here are some common use cases:
- Background Tasks: Processing large datasets, sending emails, image resizing, generating reports – these time-consuming operations can be handled asynchronously without blocking the main application flow.
- Real-time Applications: Swoole excels in real-time applications such as chat applications, online games, and stock tickers. It efficiently handles numerous concurrent connections and updates.
- Microservices Communication: Asynchronous communication between microservices can be achieved using Swoole's message queue capabilities or by leveraging its event-driven architecture.
- Task Queues: Swoole can be used to implement robust task queues, allowing for efficient distribution and processing of tasks. This is especially useful for handling large volumes of asynchronous requests.
- WebSockets: Swoole provides excellent support for WebSockets, enabling real-time bidirectional communication between clients and servers.
- Long-polling: Handling long-polling requests efficiently, keeping connections open without blocking the server.
By using Swoole for these tasks, you improve the responsiveness and scalability of your PHP applications significantly. The ability to perform these operations concurrently allows for better resource utilization and enhanced user experience.
The above is the detailed content of How to Implement Asynchronous Task Processing Using Swoole in PHP?. For more information, please follow other related articles on the PHP Chinese website!
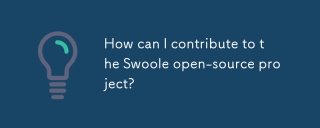
The article outlines ways to contribute to the Swoole project, including reporting bugs, submitting features, coding, and improving documentation. It discusses required skills and steps for beginners to start contributing, and how to find pressing is
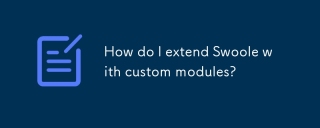
Article discusses extending Swoole with custom modules, detailing steps, best practices, and troubleshooting. Main focus is enhancing functionality and integration.
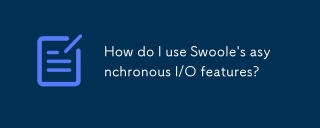
The article discusses using Swoole's asynchronous I/O features in PHP for high-performance applications. It covers installation, server setup, and optimization strategies.Word count: 159
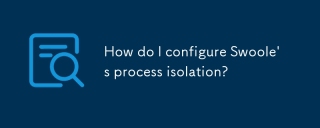
Article discusses configuring Swoole's process isolation, its benefits like improved stability and security, and troubleshooting methods.Character count: 159
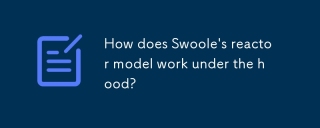
Swoole's reactor model uses an event-driven, non-blocking I/O architecture to efficiently manage high-concurrency scenarios, optimizing performance through various techniques.(159 characters)
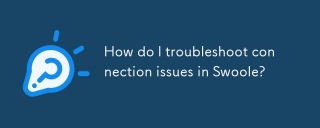
Article discusses troubleshooting, causes, monitoring, and prevention of connection issues in Swoole, a PHP framework.
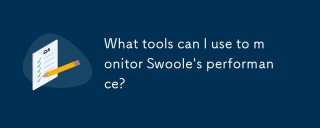
The article discusses tools and best practices for monitoring and optimizing Swoole's performance, and troubleshooting methods for performance issues.
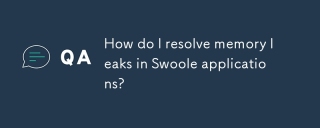
Abstract: The article discusses resolving memory leaks in Swoole applications through identification, isolation, and fixing, emphasizing common causes like improper resource management and unmanaged coroutines. Tools like Swoole Tracker and Valgrind


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
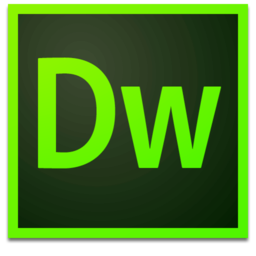
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool