This article details securing user authentication and authorization in PHP 8. It emphasizes robust password hashing, secure session management, input validation, and appropriate authorization mechanisms (RBAC, ABAC, ACLs) to mitigate vulnerabilities
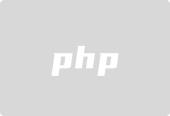
Securing User Authentication and Authorization in PHP 8
This article addresses key aspects of building secure user authentication and authorization systems within PHP 8 applications. We'll cover best practices, common vulnerabilities, and effective strategies for managing roles and permissions.
How Do I Secure User Authentication and Authorization in PHP 8?
Securing user authentication and authorization in PHP 8 involves a multi-layered approach encompassing robust password handling, secure session management, input validation, and the use of appropriate authorization mechanisms. Let's break down the key components:
-
Password Hashing: Never store passwords in plain text. Always use a strong, one-way hashing algorithm like Argon2i or bcrypt. These algorithms are computationally expensive, making brute-force attacks significantly more difficult. PHP's
password_hash()
and password_verify()
functions provide built-in support for these algorithms. Ensure you use a sufficient cost factor (e.g., a high iteration count for Argon2i) to increase the computational cost.
-
Salting and Peppering: Salting adds a unique random string to each password before hashing, preventing attackers from pre-computing hashes for common passwords. Peppering adds a secret, application-wide string to the salt, further enhancing security. While PHP's
password_hash()
automatically handles salting, consider using a secret pepper for added protection, stored securely outside of your codebase (e.g., in environment variables).
-
Secure Session Management: Use proper session handling techniques. Employ a strong session ID (using a cryptographically secure random number generator), set appropriate
session.gc_maxlifetime
and session.cookie_secure
(for HTTPS only), and session.cookie_httponly
(to prevent JavaScript access) settings. Consider using a session fixation prevention mechanism. Regularly regenerate session IDs to mitigate session hijacking risks.
-
Input Validation and Sanitization: Always validate and sanitize all user inputs before using them in queries or processing them within your application. This prevents SQL injection, cross-site scripting (XSS), and other attacks. Use parameterized queries (prepared statements) for database interactions to prevent SQL injection. Escape or encode user-supplied data appropriately before displaying it on the page to prevent XSS.
-
HTTPS: Always use HTTPS to encrypt communication between the client and the server, protecting sensitive data during transmission. Configure your web server to enforce HTTPS.
-
Rate Limiting: Implement rate limiting to mitigate brute-force attacks against login attempts. This limits the number of login attempts from a single IP address within a specific timeframe.
-
Output Encoding: Encode all output to prevent XSS vulnerabilities. Use appropriate encoding functions based on the context (e.g.,
htmlspecialchars()
for HTML output, json_encode()
for JSON responses).
What are the best practices for implementing secure user authentication in a PHP 8 application?
Beyond the points mentioned above, several best practices further enhance security:
-
Two-Factor Authentication (2FA): Implement 2FA to add an extra layer of security. This requires users to provide a second form of authentication, such as a one-time code from an authenticator app, in addition to their password.
-
Regular Security Audits: Conduct regular security audits and penetration testing to identify and address potential vulnerabilities.
-
Use Established Libraries: Leverage well-maintained and secure authentication libraries whenever possible. These libraries often provide pre-built functionality for password hashing, session management, and other security features. Thoroughly vet any third-party library before integrating it into your application.
-
Principle of Least Privilege: Grant users only the necessary permissions to perform their tasks. Avoid granting excessive privileges that could be exploited.
-
Regular Password Updates: Encourage users to regularly update their passwords and enforce password complexity requirements.
-
Robust Error Handling: Avoid revealing sensitive information in error messages. Handle errors gracefully and provide generic error messages to users.
How can I effectively handle authorization roles and permissions within my PHP 8 application?
Effective authorization management involves defining roles and assigning permissions to those roles. Several approaches can be used:
-
Role-Based Access Control (RBAC): This is a common approach where users are assigned to roles, and roles are associated with specific permissions. You can implement RBAC using a database table to store roles and permissions, and then check user permissions based on their assigned roles.
-
Attribute-Based Access Control (ABAC): This more granular approach allows for finer-grained control based on attributes of the user, resource, and environment. It's more complex to implement but offers greater flexibility.
-
Access Control Lists (ACLs): ACLs directly associate permissions with specific resources. This approach is suitable for scenarios with a relatively small number of resources.
Regardless of the chosen method, store permissions data securely in your database and ensure that permission checks are performed consistently throughout your application. Use a dedicated authorization layer to separate authorization logic from your application's core business logic. Consider using a library to simplify RBAC implementation.
What are the common vulnerabilities to avoid when building user authentication and authorization systems in PHP 8?
Several common vulnerabilities can severely compromise the security of your authentication and authorization system:
-
SQL Injection: Improperly handling user input in database queries can lead to SQL injection, allowing attackers to execute arbitrary SQL commands. Always use parameterized queries or prepared statements.
-
Cross-Site Scripting (XSS): Failing to properly sanitize user-supplied data can lead to XSS vulnerabilities, allowing attackers to inject malicious JavaScript code into your application. Always escape or encode user-supplied data before displaying it.
-
Session Hijacking: Improper session management can make your application vulnerable to session hijacking, allowing attackers to steal user sessions and impersonate users. Use strong session IDs, secure cookies, and regularly regenerate session IDs.
-
Brute-Force Attacks: Insufficient protection against brute-force attacks can allow attackers to guess user passwords by trying numerous combinations. Implement rate limiting and account lockout mechanisms.
-
Cross-Site Request Forgery (CSRF): CSRF attacks allow attackers to trick users into performing unwanted actions on your website. Use CSRF tokens to protect against these attacks.
-
Broken Authentication: Weak password policies, lack of input validation, and inadequate session management contribute to broken authentication. Follow secure coding practices and use strong authentication mechanisms.
-
Insecure Direct Object References (IDOR): Failing to properly validate object references can allow attackers to access unauthorized resources. Always validate user access to resources before granting access.
By addressing these points and following secure coding practices, you can significantly enhance the security of your user authentication and authorization system in PHP 8. Remember that security is an ongoing process, and regularly updating your application and staying informed about emerging threats is crucial.
The above is the detailed content of How Do I Secure User Authentication and Authorization in PHP 8?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn