This article demonstrates Go's network programming capabilities using TCP, UDP, and HTTP. It details server creation, handling concurrency with goroutines, and effective error management. Popular libraries (net/http, gorilla/mux, fasthttp, grpc) a
How to Use Go for Network Programming (TCP, UDP, HTTP)
Go offers built-in support for network programming, making it a popular choice for building efficient and scalable network applications. Let's explore how to use Go for TCP, UDP, and HTTP:
TCP: For TCP communication, the net
package provides the necessary tools. You create a listener using net.Listen("tcp", ":8080")
(replacing ":8080" with your desired port). This listener accepts incoming connections. Each accepted connection is a net.Conn
, allowing you to read and write data using Read
and Write
methods. Here's a basic example of a TCP server:
package main import ( "fmt" "net" ) func handleConnection(conn net.Conn) { defer conn.Close() buffer := make([]byte, 1024) for { n, err := conn.Read(buffer) if err != nil { fmt.Println("Error reading:", err) break } fmt.Println("Received:", string(buffer[:n])) _, err = conn.Write([]byte("Hello from server!")) if err != nil { fmt.Println("Error writing:", err) break } } } func main() { listener, err := net.Listen("tcp", ":8080") if err != nil { fmt.Println("Error listening:", err) return } defer listener.Close() fmt.Println("Listening on :8080") for { conn, err := listener.Accept() if err != nil { fmt.Println("Error accepting:", err) continue } go handleConnection(conn) } }
UDP: UDP uses a similar approach, but instead of net.Listen("tcp", ...)
, you use net.ListenPacket("udp", ":8081")
. You send and receive data using WriteTo
and ReadFrom
methods on the net.PacketConn
. UDP is connectionless, so each packet is independent.
HTTP: Go's net/http
package simplifies HTTP server creation. You define handlers for different routes and start a server using http.ListenAndServe
. For example:
package main import ( "fmt" "net/http" ) func helloHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") } func main() { http.HandleFunc("/", helloHandler) fmt.Println("Server listening on :8080") http.ListenAndServe(":8080", nil) }
Best Go Libraries for Building High-Performance Network Servers
Several Go libraries excel at building high-performance network servers. The choice depends on your specific needs:
-
net/http
: For general-purpose HTTP servers, the standard library'snet/http
package is often sufficient and highly optimized. Its built-in features like connection pooling and request multiplexing contribute to high performance. -
gorilla/mux
: If you need more sophisticated routing capabilities thannet/http
provides (e.g., URL parameters, named routes), thegorilla/mux
router is a popular choice. It's efficient and adds significant flexibility. -
fasthttp
: For extremely high-performance HTTP applications where every millisecond counts,fasthttp
offers significant speed improvements over the standard library. It sacrifices some ease of use for raw performance. -
grpc
: For building RPC (Remote Procedure Call) services, Google'sgrpc
library is a powerful and efficient option. It uses Protocol Buffers for serialization, resulting in compact and fast communication.
The best library often depends on the trade-off between performance, ease of use, and the complexity of your application's requirements. For many applications, net/http
or gorilla/mux
offer an excellent balance.
Handling Concurrency and Error Handling Effectively in Go Network Applications
Go's concurrency model, built around goroutines and channels, is ideal for network programming. Effective concurrency and error handling are crucial for building robust and scalable network applications.
Concurrency: Use goroutines to handle each incoming connection or request concurrently. This prevents one slow connection from blocking others. Channels can be used for communication between goroutines, for example, to signal completion or share data.
// Example using goroutines to handle multiple connections go func() { for { conn, err := listener.Accept() if err != nil { // Handle error continue } go handleConnection(conn) } }()
Error Handling: Always check for errors after each network operation. Use defer
to ensure resources (like network connections) are closed even if errors occur. Consider using context cancellation to gracefully shut down goroutines when the server is closing. Implement proper logging to track errors and diagnose problems.
Common Design Patterns for Go Network Programs
Several design patterns prove beneficial in Go network programming:
- Listener Pattern: This pattern uses a listener to accept incoming connections or requests. Each accepted connection is then handled by a separate goroutine, ensuring concurrency. This is fundamental to most network servers.
- Reactor Pattern: A single thread manages a set of connections. When events (like data arrival) occur on a connection, the reactor dispatches the event to a handler. This is efficient for a large number of concurrent connections.
- Worker Pool Pattern: This pattern creates a pool of worker goroutines that handle tasks (like processing requests). This limits the number of concurrent goroutines, preventing resource exhaustion. This is helpful for CPU-bound tasks.
- Pipeline Pattern: This pattern arranges a series of processing stages (like request validation, data processing, and response generation) in a pipeline. Each stage is a separate goroutine, allowing for parallel processing. This enhances throughput.
The choice of pattern depends on the specific needs of your application. For simple servers, the listener pattern might suffice. For high-throughput, low-latency applications, the reactor or worker pool patterns may be more appropriate. For complex processing pipelines, the pipeline pattern is a strong choice.
The above is the detailed content of How can I use Go for network programming (TCP, UDP, HTTP)?. For more information, please follow other related articles on the PHP Chinese website!
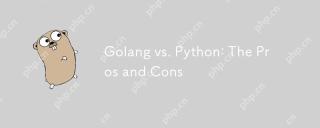
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
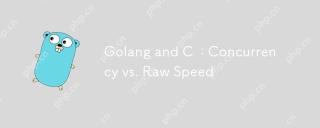
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
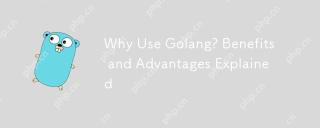
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
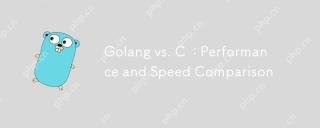
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
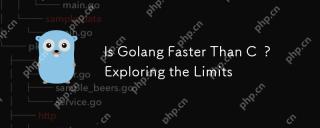
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
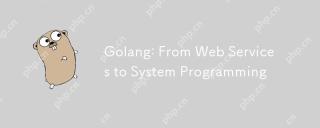
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
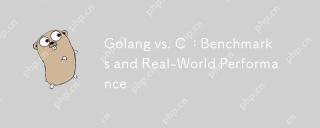
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
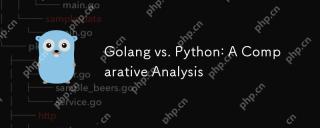
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
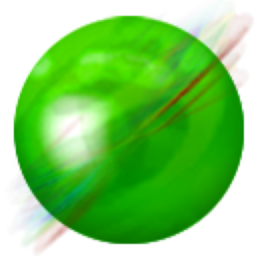
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment