How to Use Polymorphism in PHP 7?
Polymorphism in PHP 7, like in other object-oriented programming languages, allows objects of different classes to be treated as objects of a common type. This is primarily achieved through interfaces and abstract classes.
Using Interfaces:
An interface defines a contract that classes must adhere to. It specifies method signatures without providing implementations. Classes then implement the interface, providing their own concrete implementations for the methods.
// Define an interface interface Shape { public function getArea(); } // Implement the interface in different classes class Circle implements Shape { private $radius; public function __construct($radius) { $this->radius = $radius; } public function getArea() { return pi() * $this->radius * $this->radius; } } class Square implements Shape { private $side; public function __construct($side) { $this->side = $side; } public function getArea() { return $this->side * $this->side; } } // Using polymorphism $shapes = [new Circle(5), new Square(4)]; foreach ($shapes as $shape) { echo "Area: " . $shape->getArea() . PHP_EOL; }
In this example, Circle
and Square
are treated as Shape
objects. The foreach
loop iterates through an array containing both types, calling getArea()
on each. The correct implementation is executed depending on the actual object type.
Using Abstract Classes:
Abstract classes are similar to interfaces but can provide default implementations for some methods. They cannot be instantiated directly; subclasses must extend them and provide implementations for any abstract methods.
// Define an abstract class abstract class Animal { public function speak() { echo "Generic animal sound" . PHP_EOL; } abstract public function move(); } // Extend the abstract class class Dog extends Animal { public function move() { echo "Dog is running" . PHP_EOL; } } class Bird extends Animal { public function move() { echo "Bird is flying" . PHP_EOL; } } // Using polymorphism $animals = [new Dog(), new Bird()]; foreach ($animals as $animal) { $animal->speak(); $animal->move(); }
Here, Dog
and Bird
inherit from Animal
and provide their specific implementations of the move()
method. The speak()
method has a default implementation in the abstract class, but subclasses can override it if needed.
What are the practical benefits of using polymorphism in PHP 7 applications?
The practical benefits of using polymorphism in PHP 7 applications are significant:
- Flexibility and Extensibility: Easily add new classes without modifying existing code. As long as the new class adheres to the interface or extends the abstract class, it can be seamlessly integrated.
- Code Reusability: Polymorphism promotes code reuse by allowing you to write generic code that works with multiple classes. This reduces code duplication and improves maintainability.
- Improved Design: Polymorphism leads to a more modular and well-structured design. It encourages the separation of concerns and promotes a cleaner architecture.
- Testability: Polymorphism makes unit testing easier. You can easily mock or stub interfaces or abstract classes, simplifying the testing process.
- Maintainability: Changes to one class are less likely to affect other parts of the application. This reduces the risk of introducing bugs and makes maintenance easier.
How does polymorphism improve code maintainability and extensibility in PHP 7 projects?
Polymorphism directly contributes to improved code maintainability and extensibility through:
- Loose Coupling: Polymorphism reduces dependencies between classes. Instead of directly interacting with specific classes, code interacts with interfaces or abstract classes. This means changes in one class are less likely to ripple through the rest of the system.
- Open/Closed Principle: New classes can be added without modifying existing code that uses the interface or abstract class. This adheres to the open/closed principle of SOLID design principles.
- Easier Refactoring: Because of loose coupling, refactoring is simplified. You can modify or replace implementations without impacting other parts of the system, as long as the interface or abstract class contract is maintained.
- Simplified Debugging: Because of the modularity, pinpointing the source of errors becomes easier. The impact of changes is localized and easier to track.
Can you provide examples of polymorphism in PHP 7 that demonstrate its use in different scenarios?
Scenario 1: Database Interaction:
Imagine you have different database systems (MySQL, PostgreSQL). You can create an interface Database
with methods like connect()
, query()
, and disconnect()
. Then, create concrete classes MySQLDatabase
and PostgreSQLDatabase
that implement this interface. Your application code can interact with the database using the Database
interface, regardless of the actual database system used. Switching databases only requires changing the instantiation of the concrete class.
Scenario 2: Payment Processing:
You might have different payment gateways (Stripe, PayPal). Create an interface PaymentGateway
with methods like processPayment()
. Implementations like StripePaymentGateway
and PayPalPaymentGateway
would handle the specifics of each gateway. Your shopping cart application can use the PaymentGateway
interface, making it easy to add new payment options without altering core functionality.
Scenario 3: Logging:
Different logging mechanisms (file, database, email) can be implemented using an interface Logger
with a log()
method. Concrete classes like FileLogger
, DatabaseLogger
, and EmailLogger
would handle the specific logging method. Your application can use the Logger
interface, providing flexibility in choosing the logging strategy without altering the core code.
These examples demonstrate how polymorphism promotes flexibility, maintainability, and extensibility by decoupling the application logic from specific implementations. This results in cleaner, more robust, and easier-to-maintain PHP 7 applications.
The above is the detailed content of How to Use Polymorphism in PHP 7?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
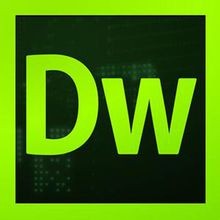
Dreamweaver CS6
Visual web development tools
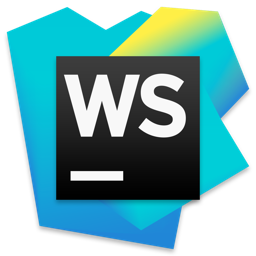
WebStorm Mac version
Useful JavaScript development tools
