What is Inheritance and How Does it Work in PHP 7?
Inheritance in PHP 7, like in other object-oriented programming languages, is a mechanism that allows a class (the child class or subclass) to inherit properties (variables) and methods (functions) from another class (the parent class or superclass). This creates an "is-a" relationship; the child class is a type of the parent class. The child class can then extend the functionality of the parent class by adding its own properties and methods, or overriding existing ones.
PHP 7 achieves inheritance using the extends
keyword. The syntax is straightforward:
<?php class ParentClass { public $name; public function __construct($name) { $this->name = $name; } public function greet() { echo "Hello, my name is " . $this->name . ".\n"; } } class ChildClass extends ParentClass { public $age; public function __construct($name, $age) { parent::__construct($name); // Call the parent class constructor $this->age = $age; } public function introduce() { echo "My name is " . $this->name . ", and I am " . $this->age . " years old.\n"; } } $child = new ChildClass("Alice", 30); $child->greet(); // Inherits greet() from ParentClass $child->introduce(); // ChildClass's own method ?>
In this example, ChildClass
inherits the name
property and the greet()
method from ParentClass
. It also adds its own age
property and introduce()
method. Crucially, the parent::__construct()
call in the ChildClass
constructor is essential to initialize the inherited properties from the parent class. Without it, the $name
property would be undefined in the ChildClass
. This demonstrates how inheritance allows for code reuse and the creation of a hierarchical class structure.
Can PHP 7 inheritance be used to improve code reusability?
Yes, PHP 7 inheritance significantly improves code reusability. By inheriting properties and methods from a parent class, child classes avoid redundant code. Instead of rewriting common functionality for each class, developers can define it once in the parent class and reuse it across multiple child classes. This leads to more concise, maintainable, and less error-prone code. Changes made to the parent class automatically propagate to all its child classes (unless overridden), simplifying updates and reducing the risk of inconsistencies. This is particularly beneficial when dealing with large projects with many classes that share common features.
What are the advantages and disadvantages of using inheritance in PHP 7 object-oriented programming?
Advantages:
- Code Reusability: As mentioned above, inheritance promotes code reuse, reducing development time and effort.
- Code Maintainability: Changes in the parent class are reflected in child classes, simplifying maintenance and reducing the chance of inconsistencies.
- Extensibility: Child classes can easily extend the functionality of parent classes by adding new methods and properties.
- Polymorphism: Inheritance supports polymorphism, allowing objects of different classes to be treated as objects of a common type. This enhances flexibility and simplifies code design.
Disadvantages:
- Tight Coupling: Inheritance creates a tight coupling between parent and child classes. Changes in the parent class can unexpectedly affect child classes, potentially breaking existing functionality.
- Fragile Base Class Problem: Modifications to the parent class can break child classes that rely on its specific implementation details.
- Limited Flexibility: Inheritance establishes a fixed "is-a" relationship, which might not always be the most appropriate design choice. Overuse can lead to complex and inflexible class hierarchies.
- Inheritance vs. Composition: The choice between inheritance and composition is crucial. Overuse of inheritance can make the code harder to understand and maintain compared to a well-designed composition-based approach.
How does inheritance differ from composition in the context of PHP 7?
Inheritance and composition are two fundamental ways to achieve code reuse in object-oriented programming. While inheritance creates an "is-a" relationship, composition establishes a "has-a" relationship.
Inheritance ("is-a"): A child class inherits properties and methods from a parent class. The child class is a specialized version of the parent class. The relationship is fixed at compile time.
Composition ("has-a"): A class contains instances of other classes as its members. The class has a relationship with other classes. This relationship is more flexible and can be changed at runtime.
Consider an example: A Car
class.
Inheritance approach: You might have a SportsCar
class that inherits from a Car
class. SportsCar is a Car
.
Composition approach: You might have a Car
class that contains instances of an Engine
class, a Transmission
class, and a BrakeSystem
class. Car has an Engine
, Car has a Transmission
, Car has a BrakeSystem
.
Composition offers greater flexibility. You can easily swap out components (e.g., using a different engine) without affecting the Car
class itself. Inheritance, on the other hand, is less flexible; changing the parent class directly affects the child class. Composition often leads to more loosely coupled and maintainable code, making it a preferred approach in many scenarios, particularly when dealing with complex systems. Choosing between inheritance and composition depends on the specific design requirements and the nature of the relationships between classes.
The above is the detailed content of What is Inheritance and How Does it Work in PHP 7?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
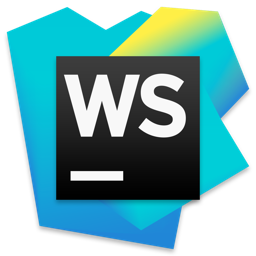
WebStorm Mac version
Useful JavaScript development tools
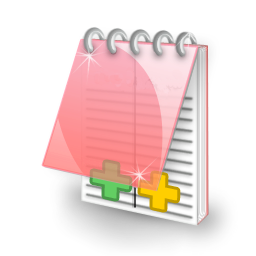
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
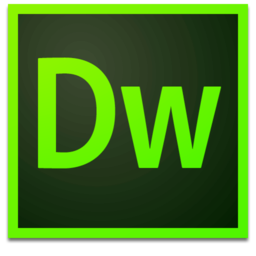
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.