How Can I Implement Secure File Uploads in PHP?
Secure file uploads in PHP require a multi-layered approach, focusing on validation, sanitization, and proper file handling. The core principle is to never trust user-supplied data. Instead, rigorously verify all aspects of the uploaded file before processing it. This includes checking file type, size, and content. Here's a breakdown:
-
Strict File Type Validation: Avoid relying solely on the client-side file extension. Instead, use the
finfo
class (recommended) or thegetimagesize()
function for image files to verify the actual file type on the server-side. This prevents users from disguising malicious files by changing the extension. For example:$finfo = new finfo(FILEINFO_MIME_TYPE); $mime_type = $finfo->file($_FILES['file']['tmp_name']); if ($mime_type != 'image/jpeg' && $mime_type != 'image/png') { // Handle invalid file type }
-
File Size Limits: Set both client-side and server-side limits on file size. The client-side limit provides a user experience improvement, preventing large uploads that will ultimately be rejected. The server-side limit is crucial for security and resource management. Use
ini_set()
to adjust theupload_max_filesize
andpost_max_size
directives in yourphp.ini
file, or use theini_get()
function to retrieve the current values and adapt your code accordingly. - File Name Sanitization: Never use the original file name directly. Instead, generate a unique file name using a combination of a timestamp, a random string, or a hash function. This prevents potential issues with file name collisions and prevents users from injecting malicious code into the file name.
- Temporary Directory: Uploaded files are initially stored in a temporary directory. Ensure this directory has appropriate permissions (only writable by the web server) and regularly clean up old temporary files.
- Destination Directory: Create a dedicated directory for storing uploaded files, outside of the webroot directory. This prevents direct access to the files via a web browser.
- Error Handling: Implement comprehensive error handling to gracefully handle issues such as exceeding file size limits, invalid file types, or disk space issues.
What are the common vulnerabilities in PHP file uploads and how can I prevent them?
Common vulnerabilities in PHP file uploads include:
-
File Type Spoofing: Users disguising malicious files by changing the file extension. Prevention: Use server-side validation with
finfo
orgetimagesize()
, as described above. -
Directory Traversal: Users attempting to access files outside the intended upload directory by manipulating the file path. Prevention: Strictly validate and sanitize file paths, avoiding the use of user-supplied data in constructing paths. Use functions like
realpath()
to canonicalize paths and prevent directory traversal attacks. - Remote File Inclusion (RFI): Users attempting to include a file from a remote server. Prevention: Never allow user input to directly influence the inclusion of files.
- Code Injection: Users uploading files containing malicious code that gets executed by the server. Prevention: Avoid directly executing uploaded files. Instead, process the files appropriately, depending on their type (e.g., image resizing, document conversion).
- Denial of Service (DoS): Users uploading excessively large files or many files to consume server resources. Prevention: Implement strict file size limits and rate limiting. Monitor server resource usage.
- Cross-Site Scripting (XSS): If the file names or file metadata are displayed directly on the website without proper sanitization, this can lead to XSS vulnerabilities. Prevention: Always sanitize and escape any user-supplied data before displaying it on the website.
How can I validate file types and sizes securely during a PHP file upload?
Secure file type and size validation requires a combination of client-side and server-side checks. Client-side checks improve the user experience, but should never be relied upon for security. Server-side validation is absolutely essential.
File Type Validation:
-
finfo
Class: This is the most reliable method. It examines the file's binary data to determine its MIME type. -
getimagesize()
Function: Useful for validating image files. It returns image dimensions and MIME type.
Avoid relying on file extensions alone!
File Size Validation:
-
$_FILES['file']['size']
: This variable contains the uploaded file's size in bytes. Compare this value against your predefined limit. -
ini_set()
/ini_get()
: Use these functions to manage theupload_max_filesize
andpost_max_size
directives in yourphp.ini
file. Ensure these limits are appropriate for your application and server resources.
Example combining both:
$finfo = new finfo(FILEINFO_MIME_TYPE); $mime_type = $finfo->file($_FILES['file']['tmp_name']); if ($mime_type != 'image/jpeg' && $mime_type != 'image/png') { // Handle invalid file type }
What are the best practices for handling uploaded files in PHP to ensure security and efficiency?
Best practices for handling uploaded files in PHP encompass security, efficiency, and maintainability:
- Use a Framework or Library: Consider using a PHP framework (like Laravel, Symfony, or CodeIgniter) or a dedicated file upload library. These often provide built-in security features and streamline the upload process.
- Input Validation and Sanitization: Always validate and sanitize all user-supplied data, including file names, types, and sizes, before processing them.
- Error Handling: Implement robust error handling to gracefully handle potential issues like file upload failures, invalid file types, or exceeding size limits.
- File Storage: Store uploaded files in a dedicated directory outside the webroot, ensuring they are not directly accessible via a web browser.
- Database Integration: Store metadata about uploaded files (like file name, size, type, and upload date) in a database. This allows for better organization and management of files.
- Unique File Names: Generate unique file names to prevent conflicts and security risks associated with predictable file names.
- Regular Cleanup: Regularly delete old or unused files to free up disk space and prevent potential security vulnerabilities.
- Logging: Log all file upload events, including successful uploads, failures, and errors. This aids in debugging, auditing, and security monitoring.
- Content Security Policy (CSP): Implement a robust CSP to mitigate XSS vulnerabilities.
- Regular Security Audits: Conduct regular security audits of your file upload system to identify and address potential vulnerabilities.
By following these guidelines, you can significantly improve the security and efficiency of your PHP file upload system. Remember that security is an ongoing process, requiring continuous vigilance and updates to adapt to evolving threats.
The above is the detailed content of How Can I Implement Secure File Uploads in PHP?. For more information, please follow other related articles on the PHP Chinese website!
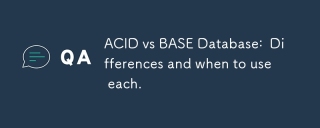
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
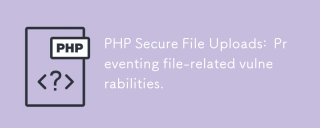
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
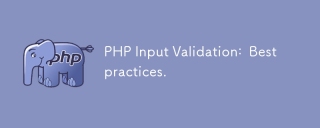
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
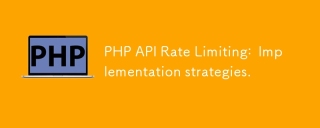
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
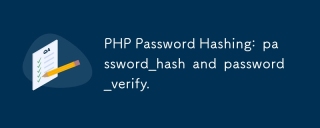
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
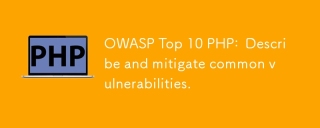
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
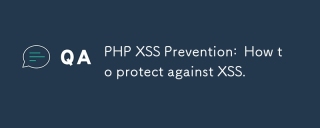
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
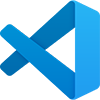
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
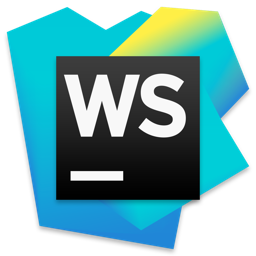
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version