How to Prevent SQL Injection Attacks in PHP Applications?
Preventing SQL injection attacks in PHP applications requires a multi-layered approach focusing on parameterized queries, input validation, and secure coding practices. The most crucial aspect is avoiding the direct concatenation of user inputs into SQL queries. Instead, always use parameterized queries or prepared statements. These methods treat user inputs as data, not as executable code, effectively neutralizing any malicious SQL commands. Databases handle the parameterization, preventing the injection of harmful code. For example, instead of:
$username = $_GET['username']; $password = $_GET['password']; $query = "SELECT * FROM users WHERE username = '$username' AND password = '$password'";
Use parameterized queries like this (using PDO, a recommended approach):
$stmt = $pdo->prepare("SELECT * FROM users WHERE username = ? AND password = ?"); $stmt->execute([$username, $password]);
This significantly reduces the risk of SQL injection. Beyond parameterized queries, regularly updating your PHP and database software is crucial. Vulnerabilities are constantly discovered, and patches address these issues, preventing attackers from exploiting known weaknesses. Finally, implementing robust input validation and output encoding further strengthens your defenses.
What are the best PHP libraries or frameworks for secure database interaction?
Several PHP libraries and frameworks excel at providing secure database interaction, minimizing the risk of SQL injection and other vulnerabilities. The most prominent and widely recommended is PDO (PHP Data Objects). PDO offers a database-agnostic approach, meaning you can switch database systems (MySQL, PostgreSQL, SQLite, etc.) with minimal code changes. Its parameterized query support is a cornerstone of secure database interaction. It handles escaping special characters automatically, preventing injection attacks.
Another excellent choice is Eloquent ORM (Object-Relational Mapper), often used within the Laravel framework. Eloquent provides an elegant, object-oriented interface for database interaction. It abstracts away many low-level details, making it easier to write secure code. Eloquent inherently uses parameterized queries, significantly reducing the chance of SQL injection.
Frameworks like Symfony and CodeIgniter also offer robust database interaction layers with built-in security features, often employing prepared statements and escaping mechanisms to safeguard against SQL injection. Choosing a well-maintained and actively developed framework or library is essential, as they regularly receive updates addressing security vulnerabilities.
How can I effectively sanitize user inputs to mitigate SQL injection vulnerabilities in my PHP code?
While parameterized queries are the primary defense against SQL injection, input sanitization plays a supporting role. Sanitization alone is insufficient to prevent SQL injection; it should be considered a secondary layer of defense, always used in conjunction with parameterized queries. The goal of input sanitization is to remove or escape potentially harmful characters before they reach the database.
However, it's crucial to understand that sanitization is context-dependent. Different types of data require different sanitization techniques. For example, simply removing characters like single quotes ('
) might not be enough; an attacker could use other characters to bypass your sanitization.
Instead of relying on custom sanitization functions, utilize built-in PHP functions where appropriate, like htmlspecialchars()
for escaping HTML entities in output (preventing XSS, not directly SQL injection, but crucial for overall security), and filter_var()
for validating and sanitizing various data types.
Avoid using functions like mysql_real_escape_string()
, which is deprecated and less robust than parameterized queries.
What are the common SQL injection attack vectors I should be aware of when developing PHP applications?
Understanding common SQL injection attack vectors is crucial for building resilient applications. Attackers exploit vulnerabilities by manipulating user inputs to inject malicious SQL code. Here are some key vectors:
-
GET parameters: Data submitted through URLs (e.g.,
index.php?id=1
) is a common target. Attackers can inject code into theid
parameter to modify the query. - POST parameters: Data submitted through forms is another vulnerable entry point. Attackers can craft malicious POST requests to inject SQL code.
- Cookies: Cookies can contain sensitive information that attackers might attempt to manipulate to inject SQL code.
- Hidden form fields: Hidden fields in forms can be manipulated by attackers to inject malicious code.
- Database error messages: Error messages revealed to the user can sometimes leak information about the database schema and structure, aiding attackers in crafting effective injection attempts. Proper error handling is crucial to prevent this.
- Stored Procedures: Even stored procedures are not entirely immune. If parameters to stored procedures are not handled correctly, they can still be vulnerable.
By understanding these vectors and using the defensive techniques discussed above, developers can significantly reduce the risk of successful SQL injection attacks. Remember that a layered approach combining parameterized queries, input validation, and secure coding practices is the most effective strategy.
The above is the detailed content of How to Prevent SQL Injection Attacks in PHP Applications?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor
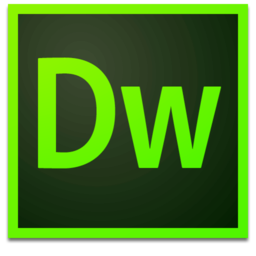
Dreamweaver Mac version
Visual web development tools
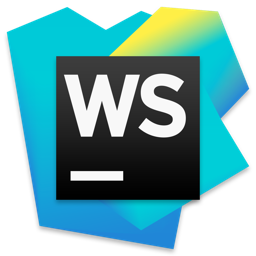
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
