Integrating Message Queues (e.g., RabbitMQ) with PHP 8
Integrating RabbitMQ with PHP 8 involves leveraging the php-amqplib
library. This library provides a robust and efficient way to interact with RabbitMQ servers. First, you need to install it using Composer:
composer require php-amqplib/php-amqplib
Then, you can establish a connection, create channels, declare exchanges and queues, publish messages, and consume messages. Here's a basic example demonstrating connection and publishing a message:
<?php require_once __DIR__ . '/vendor/autoload.php'; use PhpAmqpLib\Connection\AMQPStreamConnection; use PhpAmqpLib\Message\AMQPMessage; $connection = new AMQPStreamConnection('localhost', 5672, 'guest', 'guest'); $channel = $connection->channel(); $channel->queue_declare('my_queue', false, false, false, false); $msg = new AMQPMessage('Hello World!'); $channel->basic_publish($msg, '', 'my_queue'); echo " [x] Sent 'Hello World!'\n"; $channel->close(); $connection->close(); ?>
Remember to replace localhost
, 5672
, guest
, and guest
with your RabbitMQ server details. This code establishes a connection, declares a queue named my_queue
, and publishes a message to it. Consuming messages involves similar steps but utilizes the basic_consume
method. The php-amqplib
documentation provides comprehensive examples for various scenarios, including message acknowledgment, routing keys, and more.
Securing RabbitMQ Connections in a PHP 8 Application
Securing RabbitMQ connections in a PHP 8 application is crucial to prevent unauthorized access and data breaches. Here are several best practices:
-
Use HTTPS: Always connect to your RabbitMQ server using HTTPS. This encrypts the communication between your PHP application and the server, protecting your credentials and message data in transit. Configure your
AMQPStreamConnection
to use thessl_options
parameter for this. -
Strong Credentials: Avoid using default credentials (
guest
/guest
). Create dedicated user accounts with restricted permissions for your PHP application. Grant only the necessary permissions (e.g., publish to specific queues, consume from specific queues). - Least Privilege: Follow the principle of least privilege. Only grant the minimum necessary permissions to your application user. This limits the damage that could be caused if the application's credentials are compromised.
- Input Validation: Sanitize and validate any input data used to construct RabbitMQ messages or queue names to prevent injection attacks.
-
Regular Updates: Keep your RabbitMQ server and the
php-amqplib
library up-to-date to benefit from the latest security patches. - Firewall: Protect your RabbitMQ server with a firewall, allowing access only from trusted IP addresses or networks.
Efficient PHP 8 Libraries/Extensions for RabbitMQ
The php-amqplib
library is generally considered the most efficient and widely used library for handling RabbitMQ message processing in PHP 8. It's well-maintained, provides a comprehensive feature set, and offers good performance. While other libraries might exist, they often lack the maturity, community support, and feature completeness of php-amqplib
. Choosing a different library would require careful evaluation based on your specific needs and constraints, but for most use cases, php-amqplib
remains the preferred choice. Consider using asynchronous processing techniques (e.g., using swoole
or reactphp
extensions) for improved performance with high message volumes. These asynchronous frameworks can allow your PHP application to handle multiple connections and messages concurrently without blocking the main thread.
Implementing Reliable Message Delivery and Error Handling
Reliable message delivery and robust error handling are vital for building fault-tolerant RabbitMQ applications in PHP 8. Here's how to achieve this:
-
Message Acknowledgment: Use message acknowledgments (
basic_ack
) to ensure that messages are only considered processed after successful handling. This prevents message loss if the application crashes after receiving a message but before processing it. - Transaction Management: For critical operations requiring atomicity, use RabbitMQ transactions. This ensures that either all operations within a transaction succeed or none do.
- Dead-Letter Queues (DLQs): Configure DLQs to handle messages that fail processing. These queues capture messages that could not be processed successfully, allowing you to investigate and retry them later.
- Retry Mechanisms: Implement retry logic for failed message processing. This can involve exponential backoff strategies to avoid overwhelming the system during temporary outages.
- Error Logging: Log all errors encountered during message processing, including detailed context information to facilitate debugging and troubleshooting.
- Monitoring: Monitor your RabbitMQ server and application performance using tools like RabbitMQ's management plugin or other monitoring solutions. This helps you identify and address potential bottlenecks or errors proactively.
By implementing these strategies, you can build a resilient RabbitMQ-based system in PHP 8 that handles message delivery and errors reliably. Remember to choose appropriate retry strategies and error handling mechanisms based on the criticality of your messages and the tolerance for message loss.
The above is the detailed content of How Can I Integrate Message Queues (e.g., RabbitMQ) with PHP 8?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
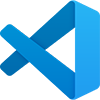
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
