How to Use Domain-Driven Design (DDD) Principles in PHP 8 Applications?
Applying DDD Principles in PHP 8
Implementing Domain-Driven Design (DDD) in PHP 8 involves focusing on the domain model, representing real-world business concepts as software objects. This requires a deep understanding of the business domain and close collaboration with domain experts. Here's a breakdown of the key steps:
-
Ubiquitous Language: Establish a shared vocabulary between developers and domain experts. This language should be consistently used throughout the codebase, documentation, and communication. In PHP, this translates to using descriptive class and method names that directly reflect the business terms. For example, instead of
processOrder
, you might usesubmitOrder
orfulfillOrder
, depending on the specific business context. - Domain Model: Create a rich domain model that encapsulates business rules and logic. This involves identifying entities (objects with unique identity), value objects (objects representing a value, like an address), and aggregates (clusters of related entities treated as a unit). PHP's object-oriented capabilities are well-suited for this, allowing you to define classes with properties and methods that reflect the domain's behavior.
- Bounded Contexts: Divide the domain into smaller, manageable parts called bounded contexts. Each context has its own ubiquitous language and domain model. This helps to manage complexity and prevents the domain model from becoming overly large and unwieldy. In PHP, this might involve separating your code into different modules or namespaces based on bounded contexts.
- Repositories and Factories: Use repositories to abstract data access from the domain model. Repositories provide an interface for retrieving and persisting domain objects. Factories are used to create domain objects, encapsulating the object creation logic. PHP's interfaces and dependency injection capabilities are crucial for implementing these patterns effectively.
- Infrastructure: Separate infrastructure concerns (database interaction, external services) from the domain model. This ensures that the domain model remains clean and focused on business logic. In PHP, you can achieve this through layers of abstraction and the use of design patterns like the Repository pattern and the Strategy pattern.
What are the best practices for implementing DDD patterns in a PHP 8 project?
Best Practices for DDD in PHP 8
Implementing DDD effectively requires adhering to several best practices:
- Start Small: Don't try to implement DDD across the entire application at once. Begin with a small, well-defined area of the application and gradually expand your DDD implementation.
- Iterative Development: Use an iterative development process to refine your domain model and DDD implementation based on feedback and evolving requirements.
- Test-Driven Development (TDD): Write unit tests for your domain model and other components to ensure correctness and facilitate refactoring. PHP's PHPUnit framework is excellent for this purpose.
- Dependency Injection: Use dependency injection to decouple components and improve testability. This is essential for maintaining a clean separation of concerns in your DDD application.
- Event Sourcing (Optional): Consider using event sourcing for persistence if your application requires a history of changes to domain objects. This provides added benefits for auditing and replaying events.
- Clear Code Structure: Organize your code into clear and well-defined layers (domain, application, infrastructure). This improves readability and maintainability. Use namespaces effectively to avoid naming conflicts.
- Continuous Collaboration: Maintain close communication between developers and domain experts throughout the development process to ensure that the domain model accurately reflects the business domain.
Can you provide examples of how to apply DDD concepts like aggregates and repositories within a PHP 8 application?
Example: Applying Aggregates and Repositories
Let's consider a simple e-commerce application. An Order
could be an aggregate root, containing associated entities like OrderItems
and a Customer
.
// Aggregate Root class Order { private $id; private $customer; private $orderItems; // ... other properties and methods ... public function addItem(OrderItem $orderItem): void { $this->orderItems[] = $orderItem; } // ... other methods ... } // Entity class OrderItem { private $product; private $quantity; // ... other properties and methods ... } // Repository interface OrderRepository { public function findById(int $id): ?Order; public function save(Order $order): void; } // Concrete Repository (e.g., using Eloquent ORM) class EloquentOrderRepository implements OrderRepository { // ... implementation using Eloquent ... }
This example shows an Order
aggregate with an OrderItem
entity. The OrderRepository
interface abstracts the data access logic, allowing for different implementations (e.g., using a database, in-memory storage, etc.).
How does using DDD improve the maintainability and scalability of PHP 8 applications?
Improved Maintainability and Scalability with DDD
DDD significantly improves maintainability and scalability in several ways:
- Improved Code Organization: DDD promotes a clear separation of concerns, leading to a more organized and maintainable codebase. The domain model is clearly separated from infrastructure concerns, making it easier to understand, modify, and test individual components.
- Reduced Complexity: By breaking down the domain into smaller, manageable bounded contexts, DDD reduces the overall complexity of the application. This makes it easier to understand and reason about individual parts of the system.
- Enhanced Testability: The clear separation of concerns fostered by DDD makes it easier to write unit tests for individual components. This improves the overall quality and reliability of the application.
- Increased Flexibility: The use of abstractions (like repositories and factories) makes it easier to adapt the application to changing requirements. For example, you can easily switch to a different database or external service without affecting the core domain logic.
- Better Scalability: By focusing on the core business logic, DDD helps to create a more robust and scalable application. The modular design makes it easier to scale individual components independently as needed. The clear separation of concerns also facilitates parallel development and deployment of different parts of the system. DDD helps to avoid tight coupling which is crucial for scalability.
The above is the detailed content of How to Use Domain-Driven Design (DDD) Principles in PHP 8 Applications?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
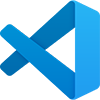
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
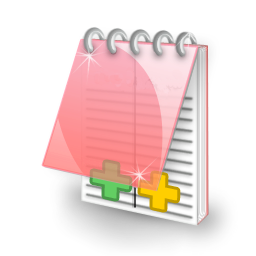
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
