How Can I Build Microservices with PHP 8?
Building microservices with PHP 8 involves leveraging its improved performance and features to create small, independent, and deployable services. The key is to adopt a well-structured approach focusing on single responsibility and loose coupling. Here's a breakdown:
1. Choose a lightweight framework: Avoid monolithic frameworks; instead, opt for micro-frameworks like Slim, Lumen (a micro-framework built on top of Laravel), or even a custom solution if your needs are very specific. These frameworks provide the necessary routing, request handling, and dependency injection capabilities without the overhead of a larger framework.
2. Define clear boundaries: Each microservice should have a single, well-defined responsibility. This promotes modularity and maintainability. Avoid creating services that handle multiple unrelated tasks.
3. Utilize API-first design: Design your services with clear and well-documented APIs (typically RESTful or gRPC) for communication. This ensures loose coupling and allows for independent evolution of each service. Consider using OpenAPI/Swagger for API specification.
4. Implement proper data management: Each microservice should manage its own data. This might involve using separate databases (e.g., MySQL, PostgreSQL, MongoDB) or data stores specific to the service's needs. Avoid shared databases across microservices to maintain independence.
5. Employ dependency injection: This promotes testability and maintainability. Dependency injection containers help manage dependencies and facilitate swapping implementations easily.
6. Implement robust logging and monitoring: Comprehensive logging and monitoring are crucial for debugging and ensuring the health of your microservices. Tools like Monolog for logging and Prometheus/Grafana for monitoring are excellent choices.
7. Versioning your APIs: Use API versioning (e.g., versioning in the URL) to allow for gradual changes and updates to your services without breaking existing clients.
8. Consider using a containerization technology (Docker): Docker allows you to package each microservice and its dependencies into a container, simplifying deployment and ensuring consistency across environments.
What are the best practices for securing PHP 8 microservices?
Securing PHP 8 microservices requires a multi-layered approach:
1. Input validation and sanitization: Always validate and sanitize all user inputs before using them in your application. Never trust user-provided data. Use parameterized queries to prevent SQL injection vulnerabilities.
2. Authentication and authorization: Implement robust authentication and authorization mechanisms. Consider using JWT (JSON Web Tokens) for authentication and role-based access control (RBAC) for authorization.
3. Secure communication: Use HTTPS to encrypt communication between your microservices and clients. Configure your web server to enforce HTTPS.
4. Regular security updates: Keep your PHP version, frameworks, and libraries up-to-date to patch known security vulnerabilities.
5. Output encoding: Encode all data before outputting it to the client to prevent cross-site scripting (XSS) attacks.
6. Rate limiting: Implement rate limiting to prevent denial-of-service (DoS) attacks.
7. Security auditing and penetration testing: Regularly audit your security practices and conduct penetration testing to identify vulnerabilities.
8. Secure your infrastructure: Secure your servers and network infrastructure against unauthorized access. Implement strong passwords and use multi-factor authentication where possible.
9. Least privilege principle: Grant only the necessary permissions to each microservice and its components.
Which message queue systems are most compatible with PHP 8 for microservice communication?
Several message queue systems integrate well with PHP 8 for microservice communication:
1. RabbitMQ: A widely used, robust, and feature-rich message broker that offers excellent PHP support through various client libraries (e.g., php-amqplib
). It supports various messaging patterns (e.g., publish/subscribe, point-to-point).
2. Redis: While primarily an in-memory data store, Redis can also be used as a message broker using its pub/sub functionality. It's known for its speed and simplicity, making it a good option for less complex scenarios. The predis
PHP client library is commonly used.
3. Kafka: A high-throughput, distributed streaming platform. It's a powerful choice for handling large volumes of data and offers excellent scalability. The kafka-php
client library provides PHP integration.
4. Amazon SQS (Simple Queue Service): A managed message queuing service offered by AWS. It's a good option if you're already using AWS services and prefer a managed solution. The AWS SDK for PHP provides seamless integration.
What frameworks or tools are recommended for building and deploying PHP 8 microservices efficiently?
Several frameworks and tools simplify building and deploying PHP 8 microservices:
1. Composer: The PHP dependency manager is essential for managing project dependencies and ensuring consistent environments.
2. PSR standards: Adhering to PHP Standards Recommendations (PSR) improves code interoperability and maintainability.
3. Docker: Containerization with Docker simplifies deployment and ensures consistency across environments. Docker Compose is helpful for managing multi-container applications.
4. Kubernetes (or similar orchestration tools): For managing and scaling your microservices in production, Kubernetes is a powerful choice. It automates deployment, scaling, and management of containerized applications.
5. CI/CD pipelines: Implement Continuous Integration and Continuous Delivery (CI/CD) pipelines using tools like GitLab CI, Jenkins, or GitHub Actions to automate the build, test, and deployment process.
6. Monitoring and logging tools: Use tools like Prometheus, Grafana, Elasticsearch, Fluentd, and Kibana (EFK stack) for comprehensive monitoring and logging. These tools help track the health and performance of your microservices.
7. API gateways: Consider using an API gateway (e.g., Kong, Tyk) to manage and route requests to your microservices, providing features like authentication, authorization, and rate limiting.
By following these recommendations and choosing the right tools for your specific needs, you can effectively build, secure, and deploy robust and scalable PHP 8 microservices.
The above is the detailed content of How Can I Build Microservices with PHP 8?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
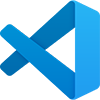
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
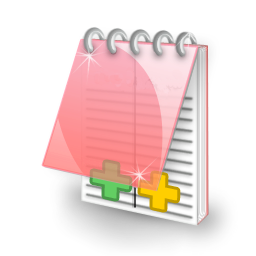
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
