Optimizing Algorithms for Performance in Go
This question delves into the core of efficient Go programming. Optimizing algorithms for performance in Go involves a multi-faceted approach, focusing on both the algorithm's design and its implementation within the Go language's specific characteristics. The key is to minimize unnecessary computations and memory allocations. Here's a breakdown of strategies:
- Choose the right algorithm: The foundation of performance lies in selecting an algorithm with the optimal time and space complexity for your specific problem. For example, using a binary search on a sorted array is significantly faster than a linear search. Understanding Big O notation (O(n), O(log n), O(n^2), etc.) is crucial for making informed decisions.
- Data Structures: The choice of data structure significantly impacts performance. For instance, using a map (hash table) for fast lookups is preferable to iterating through a slice if you need to frequently access elements by key. Consider the trade-offs between different data structures in terms of insertion, deletion, and search times.
- Minimize allocations: Go's garbage collector is efficient, but frequent allocations can still cause performance issues. Reusing buffers and avoiding unnecessary allocations, particularly within loops, can dramatically improve performance. Techniques like object pooling can be helpful in scenarios with high object churn.
- Avoid unnecessary computations: Identify and eliminate redundant calculations. Memoization, caching frequently accessed results, and loop unrolling (in appropriate cases) can significantly reduce computational overhead.
- Concurrency: Go's concurrency features (goroutines and channels) can be leveraged to parallelize computations and improve performance, especially for I/O-bound or CPU-bound tasks. However, be mindful of the overhead introduced by concurrency and ensure that the gains outweigh the costs.
Common Go Performance Bottlenecks and How to Identify Them
Several common bottlenecks can hinder the performance of Go applications. Identifying them is crucial for targeted optimization.
- Garbage Collection: Excessive garbage collection pauses can significantly impact responsiveness. This often stems from frequent memory allocations. Profiling tools (discussed later) can highlight areas with high allocation rates.
-
I/O Operations: Slow I/O (disk, network) can be a major bottleneck. Asynchronous I/O operations, using techniques like
net/http
's non-blocking features, can mitigate this. - Inefficient Algorithms: Using algorithms with poor time complexity (e.g., O(n^2) for large datasets) is a primary source of performance issues. Profiling and algorithmic analysis are essential for identifying these.
- Context Switching: Excessive context switching between goroutines can introduce overhead. Careful design of concurrent programs, avoiding excessive goroutine creation and using appropriate synchronization primitives, is important.
- Unoptimized Data Structures: Using inappropriate data structures (e.g., using a slice for frequent lookups instead of a map) leads to performance degradation.
Identifying Bottlenecks: The pprof
tool (part of the Go standard library) is invaluable for profiling Go applications. It allows you to analyze CPU usage, memory allocation, and blocking profiles to pinpoint performance hotspots. Using benchmarks (testing
package) is also crucial for quantifying performance improvements after optimizations.
Profiling Go Code to Pinpoint Areas for Algorithmic Optimization
The pprof
tool is the key to profiling Go code for algorithmic optimization. It provides several profiling modes:
- CPU Profiling: This identifies functions consuming the most CPU time. High CPU usage in specific functions often points to inefficient algorithms or computations within those functions.
- Memory Profiling: This highlights areas with high memory allocation rates. Excessive allocations can lead to increased garbage collection pauses and decreased performance. It helps identify potential areas where memory reuse or more efficient data structures could be beneficial.
- Blocking Profiling: This reveals goroutines that are blocked waiting for resources (e.g., I/O, mutexes). It helps in identifying concurrency bottlenecks.
Using pprof: You can instrument your code to generate profile data, then use the pprof
command-line tool to analyze the data. Visualizing the profiles using tools like go tool pprof
(command-line) or web-based profilers provides a clear view of performance bottlenecks. Focus on functions consuming a disproportionate amount of CPU time or allocating excessive memory – these are prime candidates for algorithmic optimization.
Best Practices for Writing Efficient Algorithms in Go
Several best practices contribute to writing efficient algorithms in Go:
- Use appropriate data structures: Select data structures based on their time complexity for your specific operations (e.g., maps for fast lookups, slices for ordered sequences).
- Minimize allocations: Reuse buffers, avoid unnecessary allocations within loops, and consider object pooling for frequently created objects.
- Optimize for the common case: Focus on optimizing the most frequently executed parts of your code. Profiling can help identify these hotspots.
- Write clear and concise code: Clean code is easier to understand and optimize. Avoid unnecessary complexity.
- Use built-in functions: Go's standard library provides highly optimized functions for many common tasks. Leverage these whenever possible.
-
Benchmark your code: Use the
testing
package's benchmarking capabilities to measure the performance of your algorithms and track improvements after optimizations. - Profile regularly: Profiling is an iterative process. Regular profiling helps identify new bottlenecks as your code evolves.
By following these best practices and utilizing Go's profiling tools, you can write efficient and high-performing algorithms. Remember that optimization is an iterative process; continuous profiling and refinement are key to achieving optimal performance.
The above is the detailed content of How do I optimize algorithms for performance in Go?. For more information, please follow other related articles on the PHP Chinese website!
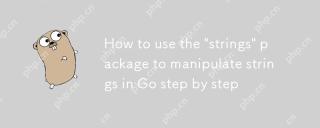
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
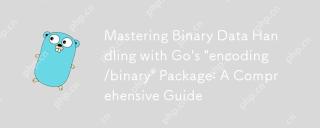
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
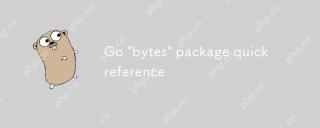
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
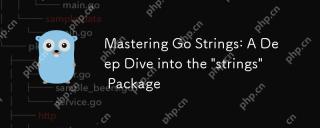
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
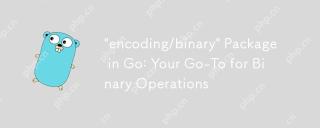
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
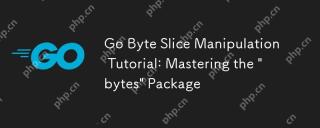
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.
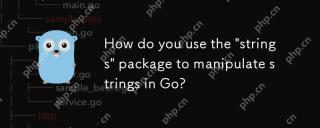
You can use the "strings" package in Go to manipulate strings. 1) Use strings.TrimSpace to remove whitespace characters at both ends of the string. 2) Use strings.Split to split the string into slices according to the specified delimiter. 3) Merge string slices into one string through strings.Join. 4) Use strings.Contains to check whether the string contains a specific substring. 5) Use strings.ReplaceAll to perform global replacement. Pay attention to performance and potential pitfalls when using it.
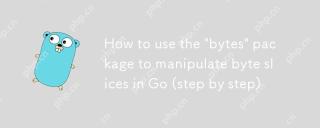
ThebytespackageinGoishighlyeffectiveforbyteslicemanipulation,offeringfunctionsforsearching,splitting,joining,andbuffering.1)Usebytes.Containstosearchforbytesequences.2)bytes.Splithelpsbreakdownbyteslicesusingdelimiters.3)bytes.Joinreconstructsbytesli


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
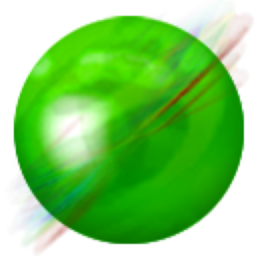
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
