


How do I write generic functions that work with different numeric types in Go?
How to Write Generic Functions that Work with Different Numeric Types in Go?
Go's generics, introduced in Go 1.18, allow you to write functions that operate on various types without resorting to type assertions or cumbersome type switches. For numeric types, this significantly enhances code reusability and readability. The key is to constrain the generic type parameter to a specific set of numeric types using the constraints
package.
To write a generic function that works with different numeric types, you need to import the constraints
package and use its predefined constraints like constraints.Integer
or constraints.Float
. These constraints restrict the generic type parameter to only those types satisfying the constraint. For example, let's create a generic function to find the maximum of two numbers:
package main import ( "fmt" "golang.org/x/exp/constraints" ) func Max[T constraints.Ordered](a, b T) T { if a > b { return a } return b } func main() { fmt.Println(Max[int](10, 5)) // Output: 10 fmt.Println(Max[float64](3.14, 2.71)) // Output: 3.14 //fmt.Println(Max[string]("hello", "world")) // This will result in a compile-time error }
This Max
function works with any type that implements the constraints.Ordered
interface, which includes int
, int8
, int16
, int32
, int64
, uint
, uint8
, uint16
, uint32
, uint64
, float32
, float64
, and others. Attempting to use it with a non-ordered type (like string
) will result in a compile-time error, preventing runtime crashes.
Can Go's Generics Handle Various Numeric Types Without Type Assertions?
Yes, Go's generics can handle various numeric types without type assertions. The use of type constraints eliminates the need for explicit type checks and conversions. Type assertions are error-prone and make code less readable. By using constraints, the compiler enforces type safety at compile time, ensuring that only appropriate types are used with the generic function. The previous example perfectly demonstrates this: the Max
function operates directly on the generic type T
, without any need for switch
statements or type assertions to handle different numeric types.
What Are the Best Practices for Writing Generic Numeric Functions in Go to Maintain Code Readability and Efficiency?
Several best practices can help maintain code readability and efficiency when writing generic numeric functions in Go:
-
Use descriptive names: Choose meaningful names for generic type parameters and functions to clearly indicate their purpose and constraints. For instance, using
Number
as a generic type parameter might be too broad;Integer
orFloat
would be more precise. - Keep functions focused: Design generic functions to perform a single, well-defined task. This improves readability and maintainability. Avoid creating overly complex generic functions that try to do too much.
-
Leverage existing constraints: The
constraints
package provides many useful constraints. Utilize them to avoid defining your own constraints unless absolutely necessary. This ensures consistency and avoids potential errors. - Consider performance implications: While generics improve code readability, be mindful of potential performance impacts. In some cases, type-specific implementations might be more efficient. Profile your code to identify performance bottlenecks and consider optimizations if needed.
- Use comments effectively: Clearly document the constraints and behavior of your generic functions to improve understanding.
- Error Handling: While type errors are caught at compile time thanks to constraints, consider other potential errors (e.g., division by zero). Implement appropriate error handling mechanisms.
How Do I Avoid Type-Related Errors When Using Generics with Numeric Types in Go?
The primary way to avoid type-related errors is by effectively using type constraints. The constraints
package provides a robust mechanism to restrict the types that can be used with your generic functions. By carefully choosing the appropriate constraint, you prevent the compiler from allowing incompatible types, eliminating runtime errors.
Beyond constraints:
- Careful testing: Thoroughly test your generic functions with various numeric types to ensure correctness and identify any unexpected behavior.
- Code reviews: Have another developer review your code to catch potential type-related errors that you might have missed.
- Static analysis tools: Utilize static analysis tools to identify potential issues in your code, including type-related errors. These tools can often detect problems that are missed during manual code reviews.
By following these practices, you can effectively leverage Go's generics for numeric types while maintaining code quality, readability, and preventing runtime errors.
The above is the detailed content of How do I write generic functions that work with different numeric types in Go?. For more information, please follow other related articles on the PHP Chinese website!
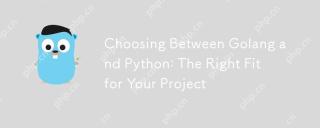
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
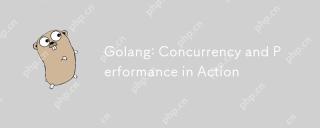
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
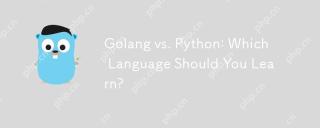
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
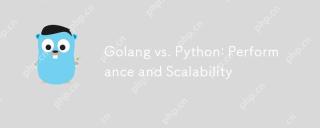
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
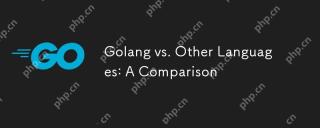
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
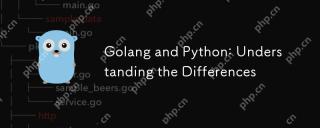
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
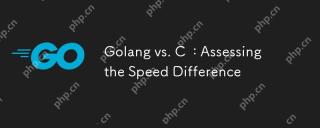
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
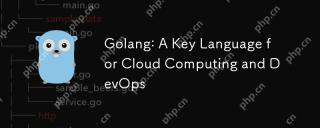
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
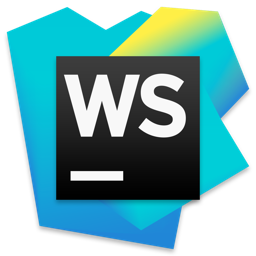
WebStorm Mac version
Useful JavaScript development tools
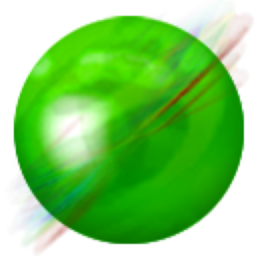
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.