


How do I profile and optimize the performance of concurrent Go applications?
How do I profile and optimize the performance of concurrent Go applications?
Profiling and optimizing concurrent Go applications requires a multi-faceted approach, combining tools and best practices. The process generally involves these steps:
1. Identify Bottlenecks: Begin by profiling your application to pinpoint performance bottlenecks. Go's built-in profiling tools are a great starting point. The pprof
tool allows you to profile CPU usage, memory allocation, and blocking profiles. You can use it with the runtime/pprof
package to generate profile data during your application's runtime. Analyze the resulting profile data (often visualized using tools like go tool pprof
) to identify functions consuming excessive CPU time, memory leaks, or significant blocking.
2. Optimize CPU Usage: High CPU usage often indicates inefficient algorithms or excessive computations within goroutines. Focus on optimizing these specific functions. Consider techniques like algorithm optimization, using more efficient data structures, and reducing redundant calculations. Profiling helps identify the specific functions to target.
3. Optimize Memory Allocation: Frequent garbage collection can severely impact performance. Minimize memory allocations by reusing buffers, using sync.Pool for temporary objects, and avoiding unnecessary object creation. The memory profiler from pprof
helps locate areas with excessive allocations. Consider using techniques like object pooling to reduce allocation overhead.
4. Reduce Concurrency Overhead: While concurrency is powerful, excessive goroutine creation and context switching can lead to performance degradation. Carefully manage the number of active goroutines, ensuring they are appropriately balanced with available resources. Use techniques like worker pools to limit the number of concurrently running goroutines. Avoid unnecessary channel operations, as they introduce overhead.
5. Analyze Blocking Profiles: Blocking profiles reveal where your goroutines are spending time waiting. This can highlight synchronization issues, such as excessive contention on shared resources or deadlocks. Address these blocking points by optimizing synchronization mechanisms, using more efficient data structures, or restructuring your code to reduce contention.
6. Iterative Optimization: Profiling and optimization is an iterative process. After making changes, re-profile your application to assess the impact of your optimizations and identify any new bottlenecks that may have emerged.
What tools are best suited for identifying performance bottlenecks in concurrent Go programs?
Several tools are invaluable for identifying performance bottlenecks in concurrent Go applications:
-
go tool pprof
: This is the core profiling tool in the Go ecosystem. It integrates with theruntime/pprof
package to generate various profiles (CPU, memory, block) that you can then analyze. It allows you to visualize call graphs, flame graphs, and identify hot spots in your code. -
go test -bench
: Thego test
command with the-bench
flag is used for benchmarking your code. It helps measure the performance of specific functions or parts of your application, allowing you to compare different implementations and identify areas for improvement. -
go vet
: While not strictly a profiler,go vet
is a static analysis tool that can detect potential issues in your code, including some that might lead to performance problems. It can help you catch errors early in the development process. - Delve (dlv): Delve is a debugger for Go that allows you to step through your code, inspect variables, and examine the execution flow. While not a direct profiler, it's invaluable for understanding the behavior of your concurrent code and identifying potential issues that may be impacting performance.
- Third-party profilers: Several third-party profiling tools offer more advanced features or integrations with other monitoring systems. These tools often provide more detailed visualizations and insights into application performance.
How can I effectively manage goroutines and channels to avoid deadlocks and improve concurrency efficiency in Go?
Effective goroutine and channel management is crucial for building robust and efficient concurrent Go applications. Here's how:
- Limit Goroutine Creation: Avoid creating an unbounded number of goroutines. Use techniques like worker pools to limit the maximum number of concurrently running goroutines. This prevents resource exhaustion and improves responsiveness.
- Proper Channel Usage: Use channels correctly for communication and synchronization between goroutines. Understand the difference between buffered and unbuffered channels. Unbuffered channels provide synchronization, while buffered channels allow for some degree of asynchronous communication. Ensure proper handling of channel closure to prevent deadlocks.
-
Avoid Data Races: Protect shared resources (variables, data structures) using synchronization primitives like mutexes (
sync.Mutex
) or atomic operations (sync/atomic
). Data races can lead to unpredictable behavior and performance issues. -
Context Management: Use the
context
package for managing the lifecycle of goroutines. Thecontext.WithCancel
function allows you to gracefully shut down goroutines when needed, preventing leaks and improving resource management. -
Deadlock Prevention: Deadlocks occur when two or more goroutines are blocked indefinitely, waiting for each other. Carefully analyze your code for potential deadlock situations. Use tools like the Go race detector (
go run -race
) to detect potential data races that might lead to deadlocks. - Error Handling: Implement proper error handling in your concurrent code. Ignoring errors can lead to unexpected behavior and potentially deadlocks. Use channels to propagate errors effectively between goroutines.
What are common performance anti-patterns to watch out for when developing concurrent Go applications?
Several common anti-patterns can significantly impact the performance of concurrent Go applications:
- Unbounded Goroutine Creation: Creating an unlimited number of goroutines without any control mechanism will quickly lead to resource exhaustion and performance degradation. Always use some form of limiting mechanism, such as a worker pool.
- Excessive Channel Operations: Frequent channel sends and receives can introduce overhead. Optimize your code to minimize unnecessary channel operations. Consider using buffered channels where appropriate to reduce blocking.
-
Ignoring Context: Failing to use the
context
package for managing the lifecycle of goroutines can lead to resource leaks and difficulties in shutting down the application gracefully. - Incorrect Synchronization: Improper use of synchronization primitives (mutexes, channels) can lead to data races, deadlocks, and unpredictable behavior, severely impacting performance.
-
Inefficient Data Structures: Using inappropriate data structures for concurrent access can introduce significant overhead. Choose data structures designed for concurrent access (e.g.,
sync.Map
) where appropriate. - Ignoring Error Handling: Ignoring errors can lead to unexpected behavior and potentially deadlocks. Always handle errors properly in concurrent code.
- Overuse of Mutexes: While mutexes are necessary for protecting shared resources, overuse can lead to contention and performance bottlenecks. Consider alternative synchronization techniques, such as atomic operations, where appropriate.
By understanding and avoiding these anti-patterns, and by leveraging the available profiling and debugging tools, you can significantly improve the performance and robustness of your concurrent Go applications.
The above is the detailed content of How do I profile and optimize the performance of concurrent Go applications?. For more information, please follow other related articles on the PHP Chinese website!
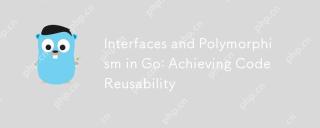
InterfacesandpolymorphisminGoenhancecodereusabilityandmaintainability.1)Defineinterfacesattherightabstractionlevel.2)Useinterfacesfordependencyinjection.3)Profilecodetomanageperformanceimpacts.
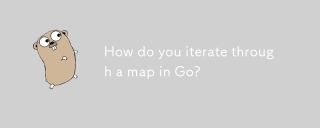
Article discusses iterating through maps in Go, focusing on safe practices, modifying entries, and performance considerations for large maps.Main issue: Ensuring safe and efficient map iteration in Go, especially in concurrent environments and with l
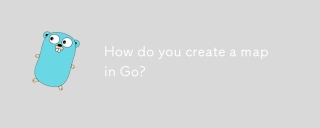
The article discusses creating and manipulating maps in Go, including initialization methods and adding/updating elements.
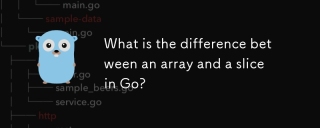
The article discusses differences between arrays and slices in Go, focusing on size, memory allocation, function passing, and usage scenarios. Arrays are fixed-size, stack-allocated, while slices are dynamic, often heap-allocated, and more flexible.
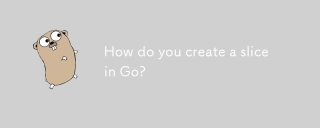
The article discusses creating and initializing slices in Go, including using literals, the make function, and slicing existing arrays or slices. It also covers slice syntax and determining slice length and capacity.
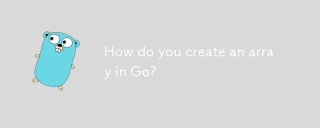
The article explains how to create and initialize arrays in Go, discusses the differences between arrays and slices, and addresses the maximum size limit for arrays. Arrays vs. slices: fixed vs. dynamic, value vs. reference types.
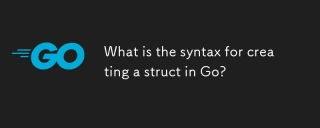
Article discusses syntax and initialization of structs in Go, including field naming rules and struct embedding. Main issue: how to effectively use structs in Go programming.(Characters: 159)
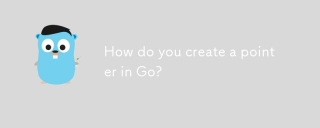
The article explains creating and using pointers in Go, discussing benefits like efficient memory use and safe management practices. Main issue: safe pointer use.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
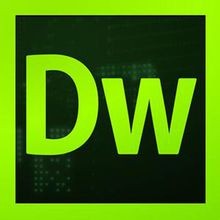
Dreamweaver CS6
Visual web development tools
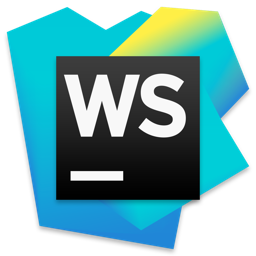
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
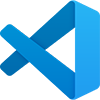
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
