PHP 8: Mastering Union Types for Cleaner Code
This section delves into the core concept of Union Types in PHP 8 and how they contribute to writing cleaner, more understandable code. Before PHP 8, if a function or method could accept multiple data types, you often relied on @param
docblocks or loose type hinting (or no type hinting at all). This led to potential runtime errors and made code harder to understand and maintain. Union types solve this problem elegantly. They allow you to specify that a parameter or return value can be one of several types, explicitly defining the allowed possibilities. For example, a function might accept either a string or an integer:
function greet(string|int $name): string { if (is_string($name)) { return "Hello, " . $name . "!"; } else { return "Hello, user #" . $name; } }
This clearly communicates to both the developer and the PHP interpreter that $name
can be a string or an integer. The type declaration is explicit, making the code self-documenting and reducing the likelihood of unexpected behavior caused by incorrect data types. This leads to more robust and maintainable codebases. The vertical bar (|
) acts as the "or" operator, combining the allowed types. Union types are a significant improvement over previous methods, enhancing code clarity and reducing ambiguity.
How can Union Types improve the readability and maintainability of my PHP 8 code?
Union types significantly boost readability and maintainability in several ways:
- Improved Code Clarity: By explicitly stating the allowed data types for a function parameter or return value, union types remove ambiguity. Developers immediately understand the possible inputs and outputs, reducing the need to delve into the function's implementation to determine acceptable data types.
- Enhanced Self-Documentation: Union types serve as built-in documentation. The type declaration itself explains the function's expected input and output, reducing reliance on separate docblocks (though docblocks are still valuable for adding further context).
- Early Error Detection: The PHP interpreter can perform type checking during development, identifying type errors early in the development cycle. This reduces the chances of runtime errors and facilitates easier debugging.
- Refactoring Made Easier: When refactoring code, understanding the data types a function handles is crucial. Union types make this understanding immediate, reducing the risk of introducing type-related bugs during the refactoring process.
- Better Collaboration: In team environments, clear type declarations are essential for smooth collaboration. Union types improve code understanding across the team, leading to more efficient and less error-prone development.
What are the best practices for effectively using Union Types in complex PHP 8 applications?
While union types are powerful, effective usage in complex applications requires careful consideration:
- Keep Unions Concise: Avoid overly long unions. If you have many possible types, it might indicate a design flaw. Consider refactoring your code to use more specific types or introduce new classes to encapsulate related data.
-
Use Meaningful Type Combinations: The types in a union should be logically related. A union of
string|int|DateTime
might indicate a need for better data structuring. - Document Complex Unions: While union types offer self-documentation, for very complex unions, adding a brief comment explaining the rationale behind the combination of types can be beneficial.
- Test Thoroughly: Test your code extensively to ensure that all branches of your union types are handled correctly. Use unit tests to verify that your functions behave as expected with different input types.
- Avoid Overuse: Don't use union types unnecessarily. If a function consistently accepts only one type, using a single type hint is simpler and clearer.
-
Consider Nullable Types: If a parameter can be null, remember to include
null
in your union type (e.g.,string|null
). This prevents unexpected null-related errors.
Are there any performance implications to consider when implementing Union Types in my PHP 8 projects?
The performance impact of union types is generally negligible. The runtime overhead associated with type checking is minimal and shouldn't significantly affect the performance of your application, especially compared to the benefits of improved code clarity and reduced runtime errors. The PHP engine is optimized to handle type checking efficiently. Focus on optimizing other aspects of your code for performance improvements rather than worrying about the minimal impact of union types. Premature optimization based on concerns about union type performance is rarely justified.
The above is the detailed content of PHP 8: Mastering Union Types for Cleaner Code. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use
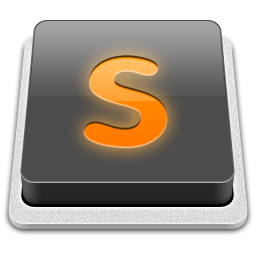
SublimeText3 Mac version
God-level code editing software (SublimeText3)