PHP 8 Tutorial: A Beginner's Guide to the Latest Features
This tutorial provides a foundational understanding of PHP 8, focusing on its key features and how to incorporate them into your projects. We'll cover aspects like improved performance, new language features, and best practices. PHP 8 represents a significant leap forward in the language's evolution, offering developers enhanced capabilities and streamlined workflows. This beginner-friendly guide aims to equip you with the essential knowledge to start using PHP 8 effectively.
What are the most significant improvements in PHP 8 compared to previous versions?
PHP 8 boasts several significant improvements over its predecessors, primarily focused on performance, safety, and developer experience. Here are some of the most impactful changes:
-
Union Types: PHP 8 introduces union types, allowing you to specify multiple possible types for a variable or function parameter. This improves type hinting and code clarity, reducing runtime errors. For example,
function greet(string|int $name): string
allows the$name
parameter to be either a string or an integer. -
Nullsafe Operator (
?->
): This operator provides a concise way to access properties or methods of an object, handling null values gracefully. If any part of the chain is null, the expression short-circuits and returns null, avoiding fatal errors. For instance,$user?->address?->street
will safely access thestreet
property, returning null if$user
or$user->address
is null. -
Named Arguments: Named arguments allow you to pass arguments to a function by name, regardless of their order. This enhances code readability and maintainability, especially in functions with many parameters. Example:
createUser(name: 'John', age: 30, email: 'john@example.com')
. -
Attributes (Metadata): Attributes provide a standardized way to add metadata to classes, methods, properties, and functions. This is useful for frameworks, static analysis tools, and other metaprogramming tasks. They replace the older
@
annotations with a more robust and structured approach. - JIT Compiler: While not a language feature itself, the introduction of a Just-In-Time (JIT) compiler significantly improves performance, especially for computationally intensive applications. This leads to faster execution speeds compared to previous PHP versions.
- Improved Error Handling: PHP 8 introduces stricter error handling, leading to fewer unexpected runtime errors. This improved type safety and the nullsafe operator contribute significantly to more robust code.
How can I effectively utilize the new features of PHP 8 in my projects?
Integrating PHP 8's new features into your projects requires a thoughtful and gradual approach. Here's how to effectively leverage them:
- Start with Union Types: Begin by incorporating union types into your codebase, particularly in function signatures and variable declarations. This improves type safety and makes your code easier to understand.
- Embrace the Nullsafe Operator: Utilize the nullsafe operator to simplify code that deals with potentially null objects. This reduces the amount of null checks required and makes your code cleaner and less prone to errors.
- Use Named Arguments Strategically: Employ named arguments in functions with multiple parameters to improve readability and reduce ambiguity. This is particularly helpful in situations where the order of arguments is not immediately obvious.
- Explore Attributes (Metadata): Investigate the use of attributes for tasks such as framework integration or custom annotations. This offers a powerful mechanism for adding metadata to your code in a structured manner.
- Refactor Gradually: Don't attempt to rewrite your entire project at once. Focus on specific areas or modules where the new features can provide the most significant benefit. A phased approach minimizes disruption and allows for thorough testing.
- Test Thoroughly: Always thoroughly test your code after introducing new features to ensure compatibility and prevent unexpected behavior.
Where can I find reliable resources beyond this tutorial to further enhance my PHP 8 skills?
Beyond this tutorial, several resources can help you expand your PHP 8 knowledge:
- Official PHP Documentation: The official PHP website provides comprehensive documentation covering all aspects of the language, including detailed explanations of PHP 8's new features.
- Online Courses and Tutorials: Platforms like Udemy, Coursera, and Codecademy offer numerous courses dedicated to PHP 8 and its advanced concepts.
- PHP Community Forums and Stack Overflow: Engage with the active PHP community on forums and Q&A sites like Stack Overflow. This is an excellent way to find solutions to specific problems and learn from experienced developers.
- Books on PHP 8: Several books focus specifically on PHP 8, offering in-depth explanations and practical examples.
- PHP Frameworks: Familiarize yourself with popular PHP frameworks like Laravel, Symfony, or CodeIgniter. These frameworks often leverage PHP 8's features to provide efficient and streamlined development workflows. Learning a framework will expose you to practical applications of PHP 8's capabilities.
By combining this tutorial with the resources mentioned above, you can effectively master PHP 8 and build robust, high-performance applications.
The above is the detailed content of PHP 8 Tutorial: A Beginner's Guide to the Latest Features. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
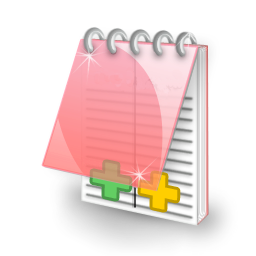
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
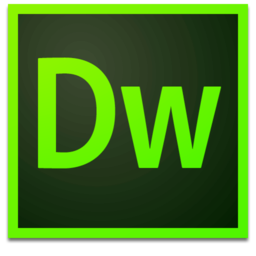
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor