Key Points
- Web Workers in JavaScript are a powerful feature in the browser that allows developers to run continuous processes in separate threads, thereby significantly improving client performance. However, they run independently of the browser UI thread and have some limitations, such as the inability to access DOM, global variables, or JavaScript functions in the page.
- To create and communicate with a dedicated Web Worker, the developer passes the JavaScript file name to a new instance of the Worker object and handles all communications through the event interface. The web script passes a single data parameter through the
postMessage()
method and receives a return data through theonmessage
event handler. - Modern browsers widely support Web Workers, but none of Internet Explorer's versions. They can use standard JavaScript data types, process XMLHttpRequest (Ajax) calls, use timers, import other Workers, and are ideal for handling time-consuming tasks such as analyzing large data blocks, game AI logic, and ray tracing.
Put aside conversions, native videos, semantic tags, and all other flashy HTML5 features; since JavaScript comes, Web Workers are the best feature in the browser! Web Workers ultimately allow developers to run ongoing processes in separate threads. Threading sounds complicated, and some development languages make it tricky, but you'll be glad to hear that JavaScript is implemented well and that the W3C working draft is stable. Web Workers offer huge client performance gains, but before we get started, there are some things to note...
Web Worker Limitations
Web Workers run independently of browser UI threads, so they do not have access to many of the features that JavaScript developers are familiar with and love. The main limitation is that Web Workers cannot access the DOM; they cannot read or modify HTML documents. Additionally, you cannot access global variables or JavaScript functions in the page. Finally, access to certain objects is restricted, for example, the window.location
attribute is read-only. However, Web Workers can use standard JavaScript data types, process XMLHttpRequest (Ajax) calls, use timers, and even import other Workers. They are ideal for handling time-consuming tasks such as analyzing large data blocks, gaming AI logic, ray tracing, and more.
Web Worker Browser Support
At the time of writing, all the latest versions of Firefox, Chrome, Safari, and Opera support Web Workers to some extent. Guess which browser does not support it? As expected, no version of Internet Explorer implements Web Workers. Even if IE9 doesn't provide support, I doubt/hope it will be implemented in the final version. Until then, you have three options:
- Forget Web Workers within a year or two.
- Accepting your application will crash in IE.
- Implement your own Web Worker shim, which falls back to a timer-based pseudo-thread or array processing. This may not be feasible or desirable in all applications.
What is a Web Worker?
Web Worker is a single JavaScript file that is loaded and executed in the background. There are two types:
- Special Workers: These Workers are associated with their creators (the scripts that load Workers).
- Shared Worker: Allows any script from the same domain (somesite.com) to communicate with Worker.
Today, we will discuss dedicated Web Workers…
Create a dedicated Web Worker
To create a dedicated Web Worker, you can pass the JavaScript file name to a new instance of the Worker object:
var worker = new Worker("thread1.js");
Communicate with dedicated Web Worker
As Web Worker cannot access the DOM or execute functions in page scripts, all communications are handled through the event interface. The web script passes a single data parameter through the postMessage()
method and receives a return data through the onmessage
event handler, for example pagescript.js:
var worker = new Worker("thread1.js"); // 接收来自 Web Worker 的消息 worker.onmessage = function(e) { alert(e.data); }; // 发送消息到 Web Worker worker.postMessage("Jennifer");
Web Worker script receives and sends data accordingly through its own onmessage
event handler and postMessage()
method: thread1.js:
self.onmessage = function(e) { self.postMessage("Hello " + e.data); };
Message data can be a string, a number, a boolean, an array, an object, null, or undefined. The data is always passed by value and is serialized and then deserialized during communication.
Processing Web Worker Error
Web Worker code is unlikely to be perfect, and logical errors can be caused by data passed by the page script. Fortunately, the error can be caught using the onerror
event handler. Handler event passes an object with 3 attributes:
-
filename
: The name of the script that caused the error; -
lineno
: The line number where the error occurred; and -
message
: Incorrect description.
pagescript.js:
worker.onerror = function(e) { alert("Error in file: "+e.filename+"\nline: "+e.lineno+"\nDescription: "+e.message); };
Load more JavaScript files
You can use importScripts()
to load one or more additional JavaScript libraries into a Web Worker, for example:
importScripts("lib1.js", "lib2.js", "lib3.js");
Or, you can load more web workers…but, I recommend keeping it simple until the browser catches up with your threading ambitions!
Stop dedicated Web Worker
You can use the close()
method to stop the Web Worker thread, such as thread1.js:
self.onmessage = function(e) { if (e.data == "STOP!") self.close(); };
This will discard any pending tasks and prevent further events from being queued. That's all you need to know about dedicated Web Workers. In my next post, we’ll discuss sharing Web Workers – a more complex guy!
FAQs (FAQs) about JavaScript Parallel Processing and HTML5 Web Workers
(The FAQ part is omitted here because the length is too long and does not match the pseudo-original goal. The FAQ part can be reorganized and rewritten as needed, but the original intention must be kept unchanged.)
The above is the detailed content of JavaScript Threading With HTML5 Web Workers. For more information, please follow other related articles on the PHP Chinese website!
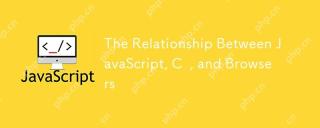
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
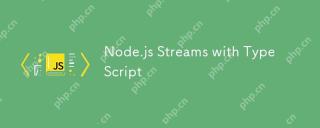
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
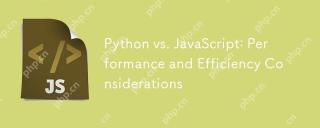
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
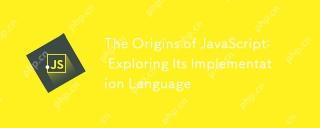
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
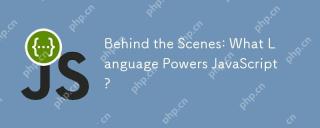
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
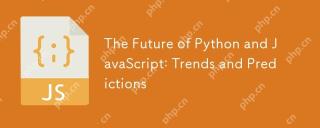
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
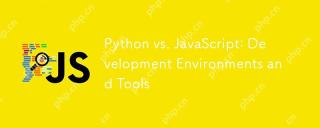
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
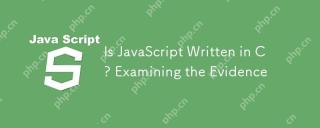
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
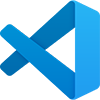
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
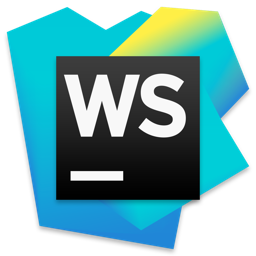
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment
