Python Beautifulsoup Example Cheat Sheet
This cheat sheet provides a quick overview of common Beautiful Soup methods for parsing HTML and XML. Remember to install it first using pip install beautifulsoup4
. We'll use a simple example HTML snippet:
<html> <head> <title>My Webpage</title> </head> <body> <h1 id="This-is-a-heading">This is a heading</h1> <p>This is a paragraph.</p> <a href="https://www.example.com">Link to Example</a> </body> </html>
Import BeautifulSoup:
from bs4 import BeautifulSoup
Parse the HTML:
html = """<html>...</html>""" # Your HTML string goes here. soup = BeautifulSoup(html, 'html.parser')
Common Methods:
-
soup.find()
: Finds the first matching tag.soup.find('h1')
would return<h1 id="This-is-a-heading">This is a heading</h1>
. -
soup.find_all()
: Finds all matching tags.soup.find_all('p')
would return a list containing<p>This is a paragraph.</p>
. -
tag.name
: Gets the tag name.soup.find('h1').name
returns'h1'
. -
tag.text
: Gets the text within a tag.soup.find('h1').text
returns'This is a heading'
. -
tag.get('attribute')
: Gets the value of an attribute.soup.find('a').get('href')
returns'https://www.example.com'
. -
tag.attrs
: Gets all attributes as a dictionary.
What are some common use cases for Beautiful Soup in web scraping with Python?
Beautiful Soup is a powerful tool for web scraping, excelling in several common use cases:
- Data Extraction from Websites: This is the most prevalent use. Beautiful Soup allows you to extract structured data from websites, such as product prices, reviews, news articles, contact information, or any other data presented in HTML or XML format. For example, you might scrape product details from an e-commerce site or gather news headlines from a news website.
- Web Content Monitoring: Track changes on websites over time. By periodically scraping a website and comparing the extracted data, you can detect updates, price changes, or other modifications. This is useful for price comparison tools, website monitoring services, or tracking competitor activity.
- Building Web Scrapers for Research: Researchers use Beautiful Soup to gather large datasets from websites for various research purposes, such as sentiment analysis of social media posts, analyzing public opinion from news articles, or studying trends in online discussions.
- Creating Data Pipelines: Integrate Beautiful Soup into larger data pipelines to automate data acquisition from websites and feed the data into other processes, such as data cleaning, analysis, or storage in a database.
- Testing Web Applications: Use Beautiful Soup to verify that a web application renders HTML correctly or to check for specific elements on a page, facilitating automated testing.
How can I efficiently extract specific data points from an HTML page using Beautiful Soup?
Efficiently extracting specific data points requires understanding the HTML structure and using appropriate Beautiful Soup methods. Here's a breakdown of strategies:
-
CSS Selectors: Utilize CSS selectors with
soup.select()
for powerful and concise selection. This is often more efficient than nestedfind()
calls. For example, to get all paragraph tags within a div with the class "content":soup.select("div.content p")
. -
Specific Attributes: If data is within tags possessing unique attributes, target them directly. For instance, if a price is in a
span
tag with theid="price"
attribute, usesoup.find('span', id='price').text
. -
Navigating the Tree: Use methods like
.find_next_sibling()
or.find_parent()
to traverse the HTML tree and locate data relative to known elements. This is crucial when data isn't directly accessible via simple selectors. -
Regular Expressions: For complex scenarios or unstructured data, combine Beautiful Soup with regular expressions to extract data based on patterns within the text. Use
re.findall()
after extracting the relevant text using Beautiful Soup. -
Lambda Functions: Employ lambda functions with
find_all()
to filter results based on specific criteria. This is helpful for selecting tags based on attribute values or text content. Example:soup.find_all(lambda tag: tag.name == 'p' and 'price' in tag.text)
Remember to handle potential errors, such as missing elements, gracefully. Use try-except blocks to prevent your script from crashing if a specific element is not found.
Where can I find more advanced Beautiful Soup examples and tutorials beyond the basics?
Beyond the basic tutorials, you can find advanced Beautiful Soup resources in several places:
- Official Documentation: The official Beautiful Soup documentation is an excellent starting point, covering advanced topics and providing detailed explanations of various methods.
- Online Tutorials and Blogs: Many websites and blogs offer advanced tutorials on web scraping with Beautiful Soup. Search for topics like "advanced Beautiful Soup techniques," "web scraping with Beautiful Soup and Selenium," or "handling dynamic websites with Beautiful Soup."
- GitHub Repositories: Explore GitHub for projects that utilize Beautiful Soup for complex web scraping tasks. Examine their code to learn advanced techniques and best practices. Look for projects related to specific websites or data extraction challenges.
- Books on Web Scraping: Several books dedicated to web scraping provide in-depth coverage of Beautiful Soup and advanced scraping techniques, including handling JavaScript, dealing with pagination, and managing large datasets.
- Stack Overflow: This is a valuable resource for troubleshooting and finding solutions to specific problems encountered while using Beautiful Soup. Search for your specific issue or ask a question if you can't find an answer.
By combining these resources, you can build your skills and tackle increasingly complex web scraping projects with Beautiful Soup. Remember to always respect the website's robots.txt
file and terms of service.
The above is the detailed content of Python Beautifulsoup Example Cheat Sheet. For more information, please follow other related articles on the PHP Chinese website!
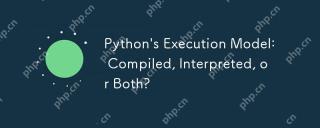
Pythonisbothcompiledandinterpreted.WhenyourunaPythonscript,itisfirstcompiledintobytecode,whichisthenexecutedbythePythonVirtualMachine(PVM).Thishybridapproachallowsforplatform-independentcodebutcanbeslowerthannativemachinecodeexecution.
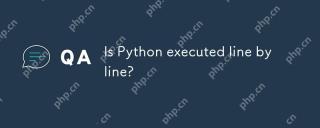
Python is not strictly line-by-line execution, but is optimized and conditional execution based on the interpreter mechanism. The interpreter converts the code to bytecode, executed by the PVM, and may precompile constant expressions or optimize loops. Understanding these mechanisms helps optimize code and improve efficiency.
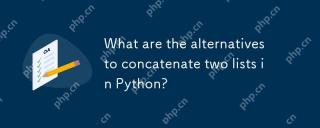
There are many methods to connect two lists in Python: 1. Use operators, which are simple but inefficient in large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use the = operator, which is both efficient and readable; 4. Use itertools.chain function, which is memory efficient but requires additional import; 5. Use list parsing, which is elegant but may be too complex. The selection method should be based on the code context and requirements.
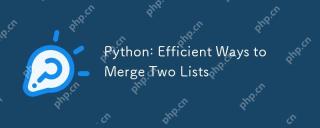
There are many ways to merge Python lists: 1. Use operators, which are simple but not memory efficient for large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use itertools.chain, which is suitable for large data sets; 4. Use * operator, merge small to medium-sized lists in one line of code; 5. Use numpy.concatenate, which is suitable for large data sets and scenarios with high performance requirements; 6. Use append method, which is suitable for small lists but is inefficient. When selecting a method, you need to consider the list size and application scenarios.
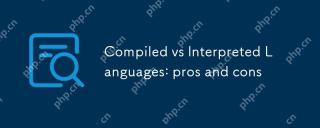
Compiledlanguagesofferspeedandsecurity,whileinterpretedlanguagesprovideeaseofuseandportability.1)CompiledlanguageslikeC arefasterandsecurebuthavelongerdevelopmentcyclesandplatformdependency.2)InterpretedlanguageslikePythonareeasiertouseandmoreportab
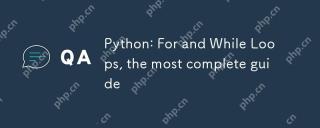
In Python, a for loop is used to traverse iterable objects, and a while loop is used to perform operations repeatedly when the condition is satisfied. 1) For loop example: traverse the list and print the elements. 2) While loop example: guess the number game until you guess it right. Mastering cycle principles and optimization techniques can improve code efficiency and reliability.
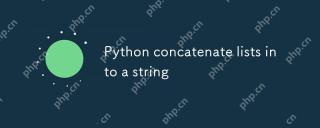
To concatenate a list into a string, using the join() method in Python is the best choice. 1) Use the join() method to concatenate the list elements into a string, such as ''.join(my_list). 2) For a list containing numbers, convert map(str, numbers) into a string before concatenating. 3) You can use generator expressions for complex formatting, such as ','.join(f'({fruit})'forfruitinfruits). 4) When processing mixed data types, use map(str, mixed_list) to ensure that all elements can be converted into strings. 5) For large lists, use ''.join(large_li
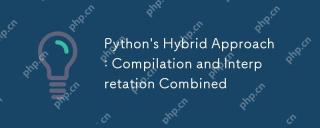
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
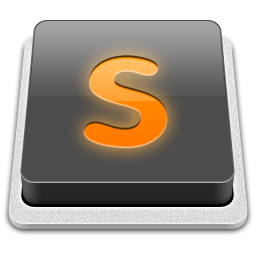
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
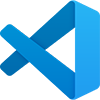
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
