Laravel's toQuery()
Method: Efficiently process large datasets
Flexible manipulation and processing data is crucial when processing large datasets in Laravel. Although collections provide powerful array manipulation methods, sometimes for efficiency we need to switch back to query builder operations. Laravel's toQuery()
method bridges this gap by converting the set back to the query builder, enabling database-level operations on the filtered dataset.
Usage toQuery()
toQuery()
method converts the Eloquent collection back to a query builder instance, allowing powerful operational chain calls:
// 基本转换 $users = User::where('status', 'active')->get(); $userQuery = $users->toQuery();
Practical Application
Let's build an order processing system with efficient batch operations:
<?php namespace App\Services; use App\Models\Order; use App\Events\OrdersProcessed; use Illuminate\Support\Facades\DB; class OrderProcessor { public function processReadyOrders() { $orders = Order::where('status', 'ready') ->where('scheduled_date', now()) ->get(); DB::transaction(function() use ($orders) { // 将集合转换为查询以进行批量更新 $orders->toQuery()->update([ 'status' => 'processing', 'processed_at' => now() ]); // 链式调用其他操作 $ordersByRegion = $orders->toQuery() ->join('warehouses', 'orders.warehouse_id', '=', 'warehouses.id') ->select('warehouses.region', DB::raw('count(*) as count')) ->groupBy('region') ->get(); event(new OrdersProcessed($ordersByRegion)); }); } public function updatePriorities() { $urgentOrders = Order::where('priority', 'high')->get(); $urgentOrders->toQuery() ->select('orders.*', 'customers.tier') ->join('customers', 'orders.customer_id', '=', 'customers.id') ->where('customers.tier', 'vip') ->update(['priority' => 'critical']); } }
This implementation demonstrates:
- Batch updates using transaction guaranteed
- Connection operation after collection conversion
- Aggregation and Grouping
By leveraging toQuery()
, you can seamlessly switch between collection and query builder operations, making your Laravel application more efficient and making your database interaction more flexible.
The above is the detailed content of Converting Collections to Queries in Laravel Using toQuery(). For more information, please follow other related articles on the PHP Chinese website!
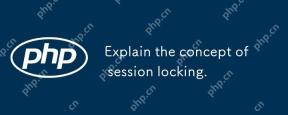
Sessionlockingisatechniqueusedtoensureauser'ssessionremainsexclusivetooneuseratatime.Itiscrucialforpreventingdatacorruptionandsecuritybreachesinmulti-userapplications.Sessionlockingisimplementedusingserver-sidelockingmechanisms,suchasReentrantLockinJ
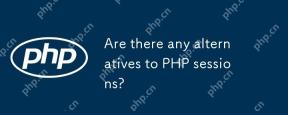
Alternatives to PHP sessions include Cookies, Token-based Authentication, Database-based Sessions, and Redis/Memcached. 1.Cookies manage sessions by storing data on the client, which is simple but low in security. 2.Token-based Authentication uses tokens to verify users, which is highly secure but requires additional logic. 3.Database-basedSessions stores data in the database, which has good scalability but may affect performance. 4. Redis/Memcached uses distributed cache to improve performance and scalability, but requires additional matching
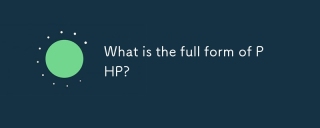
The article discusses PHP, detailing its full form, main uses in web development, comparison with Python and Java, and its ease of learning for beginners.
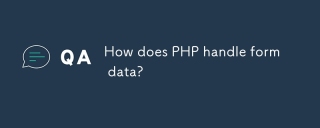
PHP handles form data using $\_POST and $\_GET superglobals, with security ensured through validation, sanitization, and secure database interactions.
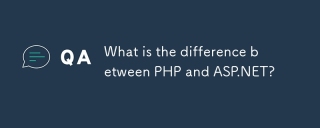
The article compares PHP and ASP.NET, focusing on their suitability for large-scale web applications, performance differences, and security features. Both are viable for large projects, but PHP is open-source and platform-independent, while ASP.NET,
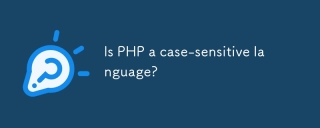
PHP's case sensitivity varies: functions are insensitive, while variables and classes are sensitive. Best practices include consistent naming and using case-insensitive functions for comparisons.
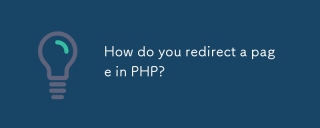
The article discusses various methods for page redirection in PHP, focusing on the header() function and addressing common issues like "headers already sent" errors.
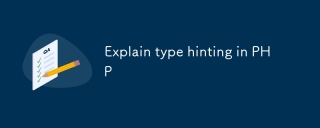
Article discusses type hinting in PHP, a feature for specifying expected data types in functions. Main issue is improving code quality and readability through type enforcement.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
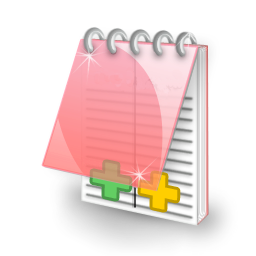
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use
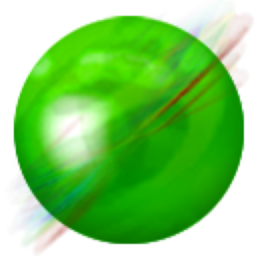
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
