Enhance your search box with jQuery: a simple yet effective guide! This tutorial demonstrates how to add default text to your search box, making it more user-friendly. The solution ensures the default text disappears on click, reappears when the box is empty, and provides visual feedback on hover.
See Live Demo
Here's the JavaScript code:
$('#search').blur(function(){ if (this.value == '') { this.value = 'Search BLOGOOLA'; } });
This jQuery code uses the blur
event to check if the search box is empty after the user interacts with it. If empty, it sets the default text.
The HTML structure (not shown in detail) should simply contain a form with an input field having the ID "search".
The CSS styling below provides the visual enhancements:
#searchform { opacity:0.8 } #searchform:hover { opacity:1.0 } #searchform fieldset { border:0px; padding:0px; margin:0px; } #searchform input { width:190px; height:16px; margin:0px 0px 0px 10px; padding:2px 5px 2px 5px; border-width:1px 1px 1px 1px; border-style:solid solid ridge solid; font-family:Arial, Helvetica, sans-serif; font-size:small; } #searchform button{ float:right; width:30px; height:22px; margin:0px 0px 0px 0px; padding:0px 0px 0px 0px; border-width:1px 1px 0px 1px; border-style:solid solid ridge solid; background-repeat:no-repeat; background-image:url('../images/search.png'); } #searchform button:hover { cursor:pointer; background-color:#E2E2E2; }
This CSS controls the opacity on hover, removes default form styling, and sets dimensions and styles for the input field and the search button.
Frequently Asked Questions (FAQs) about Adding Default Text to Search Input
This section addresses common questions regarding adding default text to a search input field, covering both HTML and CSS approaches. The original FAQ section is retained, but rephrased for clarity and conciseness. Specific code examples are included where beneficial.
HTML Placeholder Attribute
Use the HTML placeholder
attribute for a simple way to add default text. For example: <input type="search" placeholder="Enter search term...">
. The placeholder text will disappear as the user types.
Styling Placeholder Text with CSS
Control the placeholder text's color using CSS:
input::placeholder { color: red; }
This example sets the placeholder text to red. Remember that browser support for ::placeholder
varies; you may need vendor prefixes for older browsers.
JavaScript for Default Text
While the placeholder
attribute is often sufficient, you can use JavaScript to manage default text dynamically. The provided jQuery example above is one such method.
Adding an Icon
Use CSS's background-image
and background-position
properties to add an icon within the search box.
Expanding Search Box on Focus
Use the :focus
pseudo-class in CSS to expand the search box when it gains focus:
input[type="search"]:focus { width: 200px; }
Clear Button Styling
Styling the built-in clear button requires browser-specific CSS due to differing pseudo-elements. For WebKit browsers (Chrome, Safari), you might use:
input[type="search"]::-webkit-search-clear-button { color: red; }
Form Submission on Enter Key
Ensure the search box is within a <form></form>
element to automatically submit the form when the Enter key is pressed.
Autofocus on Page Load
Use the autofocus
attribute on the <input>
element to automatically focus the search box when the page loads: <input type="search" autofocus>
.
The above is the detailed content of jQuery Add Default Text To Search Input Box. For more information, please follow other related articles on the PHP Chinese website!
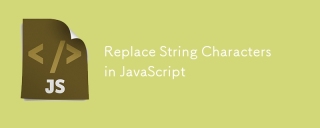
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
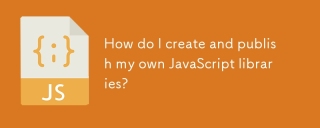
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.

The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
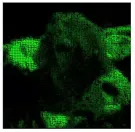
Bring matrix movie effects to your page! This is a cool jQuery plugin based on the famous movie "The Matrix". The plugin simulates the classic green character effects in the movie, and just select a picture and the plugin will convert it into a matrix-style picture filled with numeric characters. Come and try it, it's very interesting! How it works The plugin loads the image onto the canvas and reads the pixel and color values: data = ctx.getImageData(x, y, settings.grainSize, settings.grainSize).data The plugin cleverly reads the rectangular area of the picture and uses jQuery to calculate the average color of each area. Then, use
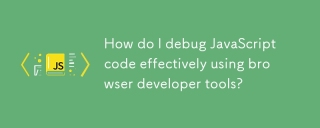
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
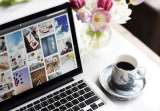
This article will guide you to create a simple picture carousel using the jQuery library. We will use the bxSlider library, which is built on jQuery and provides many configuration options to set up the carousel. Nowadays, picture carousel has become a must-have feature on the website - one picture is better than a thousand words! After deciding to use the picture carousel, the next question is how to create it. First, you need to collect high-quality, high-resolution pictures. Next, you need to create a picture carousel using HTML and some JavaScript code. There are many libraries on the web that can help you create carousels in different ways. We will use the open source bxSlider library. The bxSlider library supports responsive design, so the carousel built with this library can be adapted to any
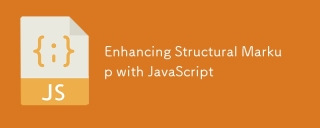
Key Points Enhanced structured tagging with JavaScript can significantly improve the accessibility and maintainability of web page content while reducing file size. JavaScript can be effectively used to dynamically add functionality to HTML elements, such as using the cite attribute to automatically insert reference links into block references. Integrating JavaScript with structured tags allows you to create dynamic user interfaces, such as tab panels that do not require page refresh. It is crucial to ensure that JavaScript enhancements do not hinder the basic functionality of web pages; even if JavaScript is disabled, the page should remain functional. Advanced JavaScript technology can be used (
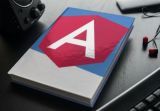
Data sets are extremely essential in building API models and various business processes. This is why importing and exporting CSV is an often-needed functionality.In this tutorial, you will learn how to download and import a CSV file within an Angular


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
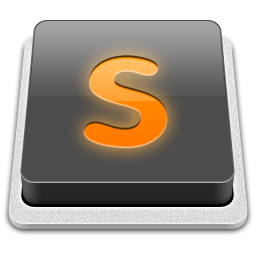
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
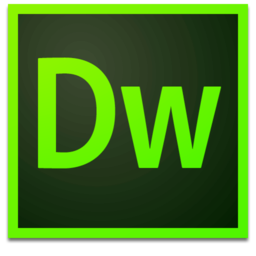
Dreamweaver Mac version
Visual web development tools