How to Call Common Functions in Yii?
Calling common functions in Yii depends on where you've placed them. There are several ways to achieve this, depending on your organizational structure. If your common functions are within a class, you'll instantiate that class and call the method. If they're in a helper file, you'll typically use include
or require
to bring them into scope. For example, if you have a Helper
class with a function formatString()
, you would call it like this:
use app\helpers\Helper; // Assuming your Helper class is in app/helpers/Helper.php $helper = new Helper(); $formattedString = $helper->formatString("This is a test string"); echo $formattedString;
Alternatively, if formatString()
is a static method, you can call it directly:
use app\helpers\Helper; $formattedString = Helper::formatString("This is another test string"); echo $formattedString;
If your common function is in a helper file (e.g., common_functions.php
), you'd include it at the top of the file where you need it:
require_once(__DIR__ . '/common_functions.php'); $result = myCommonFunction(); // myCommonFunction() defined in common_functions.php echo $result;
Remember to adjust the file paths according to your project structure. The best approach is to encapsulate common functions within classes, promoting better organization, reusability, and testability.
What are the Best Practices for Organizing Common Functions in a Yii Application?
Organizing common functions effectively is crucial for maintainability and scalability. The best practice is to encapsulate them within classes, preferably within a dedicated helpers
directory within your application's structure (e.g., app/helpers
). This promotes code reusability and adheres to object-oriented principles. Within these helper classes, group related functions logically. For example, you might have classes like StringHelper
, DateHelper
, ArrayHelper
, etc., each containing functions relevant to their respective areas. This approach improves readability and makes it easier to find specific functions. Avoid creating excessively large helper classes; instead, break down functionality into smaller, more manageable units. Using namespaces effectively will also prevent naming collisions and improve code organization. Finally, consider using traits for reusable blocks of code that can be easily included in multiple classes.
Can I Use Common Functions Across Different Controllers and Models in Yii?
Yes, absolutely. The primary method is by placing your common functions in classes (as discussed above) and then using those classes in your controllers and models. Autoloading in Yii will handle the inclusion of these classes automatically. If you have common functions that are truly universal and don't depend on any specific model or controller context, helper classes are ideal. If a function is more closely tied to a specific model or controller, consider placing it within that model or controller directly; however, if multiple controllers or models need the same function, refactoring it into a helper class is a much cleaner solution. This prevents code duplication and makes maintaining your application significantly easier.
Where Should I Place My Common Functions in a Yii Project for Optimal Accessibility and Maintainability?
The optimal location for your common functions is within the app/helpers
directory (or a similarly named directory reflecting your project's structure). This location is easily accessible from your controllers and models, promoting code organization and discoverability. Creating a dedicated helpers
directory also signals the purpose of these files clearly to other developers. Using namespaces within this directory is highly recommended to prevent naming conflicts and to improve code organization. For example, you might have a namespace like apphelpersstring
or apphelpersdatabase
. This structure makes your codebase more modular and easier to understand. Avoid placing common functions directly in your controllers
or models
directories unless they're very specific to those areas. Keeping your common functions centralized in the helpers
directory improves maintainability and reduces the risk of duplicated code.
The above is the detailed content of How to call public functions yii How to call public functions yii Tutorial. For more information, please follow other related articles on the PHP Chinese website!
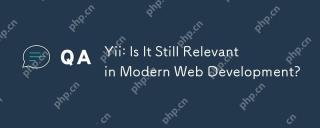
Yiiremainsrelevantinmodernwebdevelopmentforprojectsneedingspeedandflexibility.1)Itoffershighperformance,idealforapplicationswherespeediscritical.2)Itsflexibilityallowsfortailoredapplicationstructures.However,ithasasmallercommunityandsteeperlearningcu
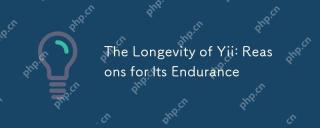
Yii frameworks remain strong in many PHP frameworks because of their efficient, simplicity and scalable design concepts. 1) Yii improves development efficiency through "conventional optimization over configuration"; 2) Component-based architecture and powerful ORM system Gii enhances flexibility and development speed; 3) Performance optimization and continuous updates and iterations ensure its sustained competitiveness.
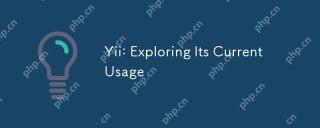
Yii is still suitable for projects that require high performance and flexibility in modern web development. 1) Yii is a high-performance framework based on PHP, following the MVC architecture. 2) Its advantages lie in its efficient, simplified and component-based design. 3) Performance optimization is mainly achieved through cache and ORM. 4) With the emergence of the new framework, the use of Yii has changed.
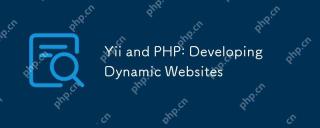
Yii and PHP can create dynamic websites. 1) Yii is a high-performance PHP framework that simplifies web application development. 2) Yii provides MVC architecture, ORM, cache and other functions, which are suitable for large-scale application development. 3) Use Yii's basic and advanced features to quickly build a website. 4) Pay attention to configuration, namespace and database connection issues, and use logs and debugging tools for debugging. 5) Improve performance through caching and optimization queries, and follow best practices to improve code quality.
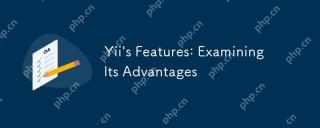
The Yii framework stands out in the PHP framework, and its advantages include: 1. MVC architecture and component design to improve code organization and reusability; 2. Gii code generator and ActiveRecord to improve development efficiency; 3. Multiple caching mechanisms to optimize performance; 4. Flexible RBAC system to simplify permission management.
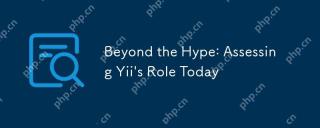
Yii remains a powerful choice for developers. 1) Yii is a high-performance PHP framework based on the MVC architecture and provides tools such as ActiveRecord, Gii and cache systems. 2) Its advantages include efficiency and flexibility, but the learning curve is steep and community support is relatively limited. 3) Suitable for projects that require high performance and flexibility, but consider the team technology stack and learning costs.
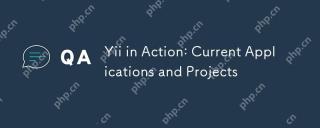
Yii framework is suitable for enterprise-level applications, small and medium-sized projects and individual projects. 1) In enterprise-level applications, Yii's high performance and scalability make it outstanding in large-scale projects such as e-commerce platforms. 2) In small and medium-sized projects, Yii's Gii tool helps quickly build prototypes and MVPs. 3) In personal and open source projects, Yii's lightweight features make it suitable for small websites and blogs.
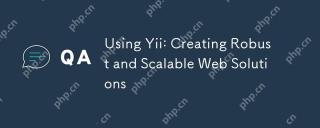
The Yii framework is suitable for building efficient, secure and scalable web applications. 1) Yii is based on the MVC architecture and provides component design and security features. 2) It supports basic CRUD operations and advanced RESTfulAPI development. 3) Provide debugging skills such as logging and debugging toolbar. 4) It is recommended to use cache and lazy loading for performance optimization.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
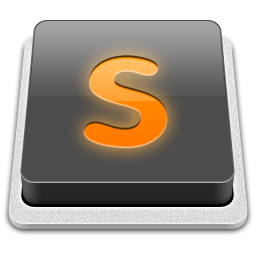
SublimeText3 Mac version
God-level code editing software (SublimeText3)
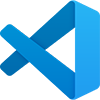
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
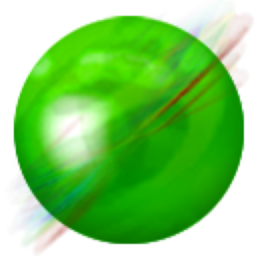
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
