Yii Framework Timestamp Setting Tutorial
This tutorial will guide you through setting up and customizing timestamps (created_at and updated_at) in your Yii models. Yii provides built-in functionality to automatically manage these timestamps, simplifying database interactions and ensuring data integrity. We'll cover various methods and customization options.
How Do I Automatically Generate Timestamps in My Yii Models?
Yii offers a straightforward way to automatically generate created_at
and updated_at
timestamps using the behaviors
property within your model. This leverages the TimestampBehavior
which handles the automatic population of these attributes.
To implement this, add the TimestampBehavior
to your model's behaviors()
method:
<?php namespace app\models; use yii\db\ActiveRecord; use yii\behaviors\TimestampBehavior; class MyModel extends ActiveRecord { public static function tableName() { return 'my_table'; } public function behaviors() { return [ TimestampBehavior::class, ]; } // ... other model code ... }
This simple addition automatically populates created_at
upon record creation and updated_at
on every update. The behavior assumes your table has columns named created_at
and updated_at
of a suitable timestamp data type (e.g., TIMESTAMP
, DATETIME
). If your column names differ, you can specify them using the attributes
property within the TimestampBehavior
configuration:
public function behaviors() { return [ [ 'class' => TimestampBehavior::class, 'attributes' => [ ActiveRecord::EVENT_BEFORE_INSERT => ['created_at', 'updated_at'], ActiveRecord::EVENT_BEFORE_UPDATE => ['updated_at'], ], //Optional: Customize value attribute (see next section for details) //'value' => new Expression('NOW()'), ], ]; }
This allows for fine-grained control over which attributes are updated during insertion and update events.
What Are the Different Ways to Handle Timestamps (created_at, updated_at) in Yii?
Besides the TimestampBehavior
, there are other ways to handle timestamps, though the behavior is generally the preferred and most efficient method. Alternative approaches include:
-
Manual Timestamping: You could manually set the timestamps within your model's
beforeSave()
method. This offers more control but requires more code and increases the risk of errors if not handled carefully.
<?php namespace app\models; use yii\db\ActiveRecord; use yii\behaviors\TimestampBehavior; class MyModel extends ActiveRecord { public static function tableName() { return 'my_table'; } public function behaviors() { return [ TimestampBehavior::class, ]; } // ... other model code ... }
- Database Triggers: You can create database triggers to automatically update timestamps. This approach is database-specific and requires knowledge of SQL. It decouples timestamp management from your Yii model but adds complexity to database maintenance.
-
Using a custom behavior: For more advanced customization beyond what
TimestampBehavior
offers, you can create your own behavior extendingTimestampBehavior
or creating a completely new one. This provides the greatest flexibility but necessitates a deeper understanding of Yii's behavior mechanism.
Can I Customize the Timestamp Format in My Yii Application?
While the TimestampBehavior
doesn't directly allow customizing the format of the timestamp (it uses the database's default handling), you can control the value assigned to the timestamp attributes. You can use a yiidbExpression
to achieve custom timestamp generation, for instance, to use a specific function from your database system.
For example, to always use the database's NOW()
function (regardless of your PHP timezone):
public function behaviors() { return [ [ 'class' => TimestampBehavior::class, 'attributes' => [ ActiveRecord::EVENT_BEFORE_INSERT => ['created_at', 'updated_at'], ActiveRecord::EVENT_BEFORE_UPDATE => ['updated_at'], ], //Optional: Customize value attribute (see next section for details) //'value' => new Expression('NOW()'), ], ]; }
Remember to adjust your database column type and potentially your database settings to handle the specific timestamp format generated by your chosen expression. Formatting for display purposes should be handled within your view using PHP's date functions or Yii's date formatting helpers. For instance, using Yii::$app->formatter->asDate($model->created_at)
in your view will format the timestamp according to your application's settings.
The above is the detailed content of How to set the yii frame timestamp tutorial. For more information, please follow other related articles on the PHP Chinese website!
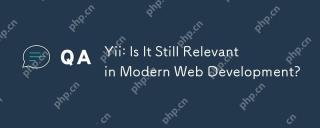
Yiiremainsrelevantinmodernwebdevelopmentforprojectsneedingspeedandflexibility.1)Itoffershighperformance,idealforapplicationswherespeediscritical.2)Itsflexibilityallowsfortailoredapplicationstructures.However,ithasasmallercommunityandsteeperlearningcu
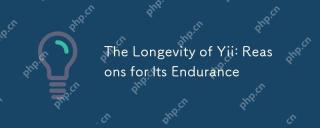
Yii frameworks remain strong in many PHP frameworks because of their efficient, simplicity and scalable design concepts. 1) Yii improves development efficiency through "conventional optimization over configuration"; 2) Component-based architecture and powerful ORM system Gii enhances flexibility and development speed; 3) Performance optimization and continuous updates and iterations ensure its sustained competitiveness.
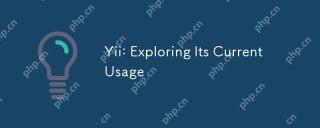
Yii is still suitable for projects that require high performance and flexibility in modern web development. 1) Yii is a high-performance framework based on PHP, following the MVC architecture. 2) Its advantages lie in its efficient, simplified and component-based design. 3) Performance optimization is mainly achieved through cache and ORM. 4) With the emergence of the new framework, the use of Yii has changed.
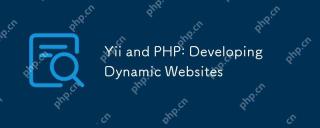
Yii and PHP can create dynamic websites. 1) Yii is a high-performance PHP framework that simplifies web application development. 2) Yii provides MVC architecture, ORM, cache and other functions, which are suitable for large-scale application development. 3) Use Yii's basic and advanced features to quickly build a website. 4) Pay attention to configuration, namespace and database connection issues, and use logs and debugging tools for debugging. 5) Improve performance through caching and optimization queries, and follow best practices to improve code quality.
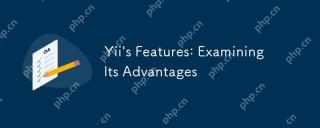
The Yii framework stands out in the PHP framework, and its advantages include: 1. MVC architecture and component design to improve code organization and reusability; 2. Gii code generator and ActiveRecord to improve development efficiency; 3. Multiple caching mechanisms to optimize performance; 4. Flexible RBAC system to simplify permission management.
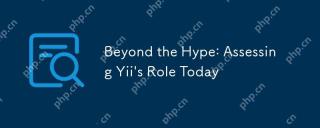
Yii remains a powerful choice for developers. 1) Yii is a high-performance PHP framework based on the MVC architecture and provides tools such as ActiveRecord, Gii and cache systems. 2) Its advantages include efficiency and flexibility, but the learning curve is steep and community support is relatively limited. 3) Suitable for projects that require high performance and flexibility, but consider the team technology stack and learning costs.
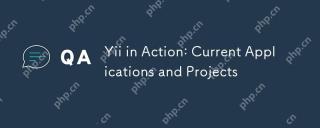
Yii framework is suitable for enterprise-level applications, small and medium-sized projects and individual projects. 1) In enterprise-level applications, Yii's high performance and scalability make it outstanding in large-scale projects such as e-commerce platforms. 2) In small and medium-sized projects, Yii's Gii tool helps quickly build prototypes and MVPs. 3) In personal and open source projects, Yii's lightweight features make it suitable for small websites and blogs.
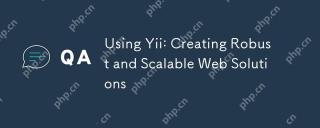
The Yii framework is suitable for building efficient, secure and scalable web applications. 1) Yii is based on the MVC architecture and provides component design and security features. 2) It supports basic CRUD operations and advanced RESTfulAPI development. 3) Provide debugging skills such as logging and debugging toolbar. 4) It is recommended to use cache and lazy loading for performance optimization.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
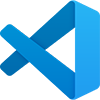
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
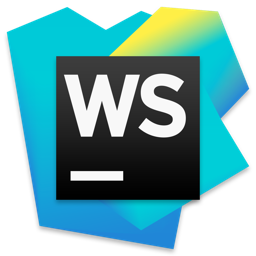
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
