ThinkPHP Pagination Tutorial: A Comprehensive Guide
This article will guide you through implementing pagination in ThinkPHP, addressing common issues and customization options.
Implementing Pagination in ThinkPHP
ThinkPHP offers a built-in pagination feature that simplifies the process of displaying large datasets across multiple pages. The core functionality relies on the ThinkPaginator
class. You'll primarily interact with this through the paginate()
method of your database query builder.
Let's assume you have a model named Article
and you want to display articles in a paginated list. Here's how you can do it:
use think\Db; // Fetch articles with pagination $articles = Db::name('article')->paginate(15); // 15 articles per page // Assign the paginated data to the template variable $this->assign('articles', $articles); // Render the view return $this->fetch();
This code snippet fetches articles from the article
table and paginates them with 15 articles per page. The paginate()
method returns a Paginator
object containing the paginated data and pagination links. The $articles
variable now holds both the articles for the current page and the pagination information. This information is automatically rendered within the view using the {$articles}
variable if you use ThinkPHP's default template engine. This includes links to previous and next pages, as well as page numbers. The default view rendering will take care of this. If you want to render the pagination manually, you can access properties of the Paginator
object, such as $articles->render()
.
Customizing Pagination Style in ThinkPHP
ThinkPHP's default pagination style might not always align with your design preferences. Fortunately, you can customize it extensively. You can achieve this primarily through the render()
method of the Paginator
object and by using template variables.
The render()
method accepts several parameters to control the appearance:
-
$config
: An array of configuration options. This allows you to modify various aspects of the pagination links, such as the list style, the number of page links displayed, and the link text. Consult the ThinkPHP documentation for the full list of configurable options.
Example:
$articles = Db::name('article')->paginate(15, false, ['type' => 'bootstrap']); // Using bootstrap style $this->assign('articles', $articles->render());
This will use a bootstrap style pagination. You can create your own custom pagination templates to have complete control over the appearance. This involves creating a custom view file and specifying its path in the configuration.
Different Pagination Methods in ThinkPHP
ThinkPHP predominantly uses the database-driven pagination approach described above. This is the most efficient method for large datasets as it only retrieves the data for the current page. There are no other distinct, officially supported "methods" in the sense of alternative algorithms. However, you could implement custom pagination logic, but this is generally not recommended unless you have very specific requirements that the built-in paginate()
method cannot handle. For instance, you might handle pagination manually for extremely large datasets by fetching data in chunks, but this comes with added complexity and potential performance issues.
Common Pitfalls to Avoid When Implementing Pagination in ThinkPHP
-
Incorrect Data Fetching: Ensure your database query correctly retrieves the data you intend to paginate. Errors in your
WHERE
clauses or joins can lead to incorrect pagination results. -
Missing or Incorrect Template Variables: Always double-check that you're correctly assigning the
Paginator
object (or itsrender()
output) to a template variable and using that variable in your view to render the pagination links. - Ignoring URL Parameters: If your application relies on URL parameters for filtering or sorting, ensure your pagination links correctly incorporate these parameters to maintain state across pages.
-
Inefficient Queries: For very large datasets, inefficient database queries can significantly impact performance. Optimize your queries using indexes and appropriate
WHERE
clauses. - Security Vulnerabilities: Sanitize user inputs used in pagination to prevent SQL injection vulnerabilities. Never directly use user-supplied values in your database queries without proper sanitization.
By following these guidelines and understanding the capabilities of ThinkPHP's pagination features, you can effectively implement and customize pagination in your applications. Remember to consult the official ThinkPHP documentation for the most up-to-date information and detailed configuration options.
The above is the detailed content of How to implement paging tutorial on thinkphp. For more information, please follow other related articles on the PHP Chinese website!
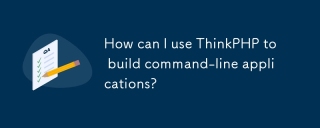
This article demonstrates building command-line applications (CLIs) using ThinkPHP's CLI capabilities. It emphasizes best practices like modular design, dependency injection, and robust error handling, while highlighting common pitfalls such as insu

The article discusses key considerations for using ThinkPHP in serverless architectures, focusing on performance optimization, stateless design, and security. It highlights benefits like cost efficiency and scalability, but also addresses challenges
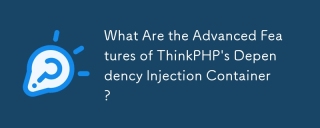
ThinkPHP's IoC container offers advanced features like lazy loading, contextual binding, and method injection for efficient dependency management in PHP apps.Character count: 159
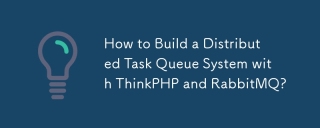
The article outlines building a distributed task queue system using ThinkPHP and RabbitMQ, focusing on installation, configuration, task management, and scalability. Key issues include ensuring high availability, avoiding common pitfalls like imprope
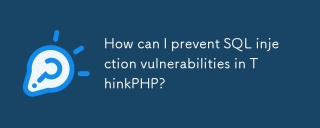
The article discusses preventing SQL injection vulnerabilities in ThinkPHP through parameterized queries, avoiding raw SQL, using ORM, regular updates, and proper error handling. It also covers best practices for securing database queries and validat
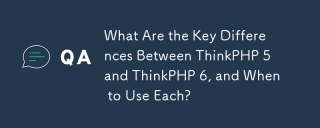
The article discusses key differences between ThinkPHP 5 and 6, focusing on architecture, features, performance, and suitability for legacy upgrades. ThinkPHP 5 is recommended for traditional projects and legacy systems, while ThinkPHP 6 suits new pr
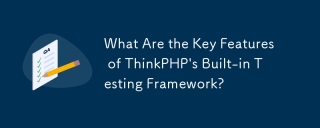
The article discusses ThinkPHP's built-in testing framework, highlighting its key features like unit and integration testing, and how it enhances application reliability through early bug detection and improved code quality.
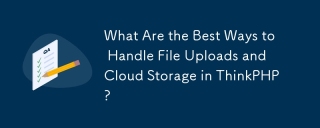
The article discusses best practices for handling file uploads and integrating cloud storage in ThinkPHP, focusing on security, efficiency, and scalability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
