Use jQuery code snippet to traverse JSON data properties. You have an object/map array, so the outer loop goes through these arrays. The inner loop traverses the properties of each object element. Update: Check out this article for 5 in-depth examples of jQuery.each().
$.each(data, function() { $.each(this, function(k, v) { /// 执行操作 }); });
Frequently Asked Questions for jQuery to traverse JSON Data (FAQs)
How to traverse JSON objects in jQuery?
It is very simple to traverse JSON objects in jQuery. You can use the $.each()
function, which is a general function provided by jQuery to iterate over the properties of an object. Here is a simple example:
var jsonObject = { "name": "John", "age": "30", "city": "New York" }; $.each(jsonObject, function(key, value) { console.log(key + ": " + value); });
In this example, the $.each()
function is used to iterate through each property (key-value pair) in jsonObject
. The function takes two parameters: the first is a JSON object and the second is a callback function that will execute on each item of the object.
How to traverse JSON array in jQuery?
Iterating through JSON arrays in jQuery is similar to traversing JSON objects. You can use the $.each()
function. Here is an example:
var jsonArray = ["John", "Jane", "Doe"]; $.each(jsonArray, function(index, value) { console.log(index + ": " + value); });
In this example, the $.each()
function is used to traverse each item in jsonArray
. The function takes two parameters: the first is a JSON array and the second is a callback function that executes on each item in the array.
How to access nested JSON data in jQuery?
Accessing nested JSON data in jQuery can use dot notation or square bracket notation. Here is an example:
var jsonObject = { "person": { "name": "John", "age": "30", "city": "New York" } }; console.log(jsonObject.person.name); // 输出:John
In this example, the nested JSON data (the "name" attribute) is accessed using point notation (jsonObject.person.name
).
How to handle errors when parsing JSON data in jQuery?
You can use the try-catch block to handle errors when parsing JSON data in jQuery. Here is an example:
try { var jsonObject = $.parseJSON(jsonString); } catch (e) { console.error("解析错误:", e); }
In this example, if jsonString
is not a valid JSON string, the $.parseJSON()
function will throw an error that will be caught and processed by the catch block.
How to filter JSON data in jQuery?
You can use the $.grep()
function to filter JSON data in jQuery. Here is an example:
var jsonArray = ["John", "Jane", "Doe"]; var result = $.grep(jsonArray, function(value) { return value !== "John"; }); console.log(result); // 输出:["Jane", "Doe"]
In this example, the $.grep()
function is used to filter out the item "John" from jsonArray
. The function takes two parameters: the first is a JSON array and the second is a callback function that executes on each item in the array. If the callback function returns true, the item will be included in the result array; if false is returned, the item will be excluded.
How to convert a JSON string to a JSON object in jQuery?
You can use the $.parseJSON()
function to convert a JSON string to a JSON object in jQuery. Here is an example:
var jsonString = '{"name":"John", "age":"30", "city":"New York"}'; var jsonObject = $.parseJSON(jsonString); console.log(jsonObject); // 输出:{name: "John", age: "30", city: "New York"}
In this example, the $.parseJSON()
function is used to convert jsonString
into a JSON object.
How to convert JSON object to JSON string in jQuery?
You can use the JSON.stringify()
function to convert a JSON object to a JSON string in jQuery. Here is an example:
$.each(data, function() { $.each(this, function(k, v) { /// 执行操作 }); });
In this example, the JSON.stringify()
function is used to convert jsonObject
to a JSON string.
How to load JSON data using AJAX in jQuery?
You can use the $.ajax()
function to load JSON data in jQuery. Here is an example:
var jsonObject = { "name": "John", "age": "30", "city": "New York" }; $.each(jsonObject, function(key, value) { console.log(key + ": " + value); });
In this example, the $.ajax()
function is used to send an AJAX request to the server, loading JSON data from the "data.json" file. If the request is successful, the data will be recorded to the console; if an error occurs, the error message will be recorded to the console.
How to load JSON data using $.getJSON()
function in jQuery?
You can use the $.getJSON()
function to load JSON data in jQuery. Here is an example:
var jsonArray = ["John", "Jane", "Doe"]; $.each(jsonArray, function(index, value) { console.log(index + ": " + value); });
In this example, the $.getJSON()
function is used to send an AJAX request to the server, loading JSON data from the "data.json" file. If the request is successful, the data will be recorded to the console; if an error occurs, the error message will be recorded to the console.
How to print JSON data beautifully in jQuery?
You can use the JSON.stringify()
function and its second and third parameters to print JSON data beautifully in jQuery. Here is an example:
var jsonObject = { "person": { "name": "John", "age": "30", "city": "New York" } }; console.log(jsonObject.person.name); // 输出:John
In this example, the JSON.stringify()
function is used to convert jsonObject
to a JSON string. The second parameter is a replacement function that changes the behavior of the stringification process, and the third parameter is a number that specifies the number of spaces to be used for indentation. By taking null as the second argument and 2 as the third argument, the JSON string will be formatted with 2 space indents, making it easier to read.
The above is the detailed content of jQuery Loop through JSON Data. For more information, please follow other related articles on the PHP Chinese website!
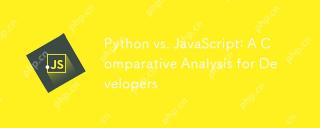
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
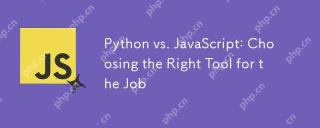
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
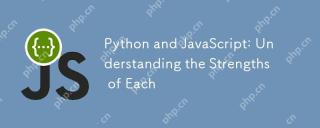
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
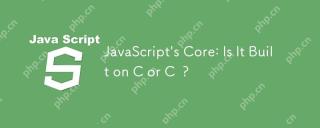
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
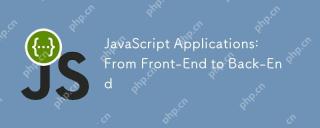
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
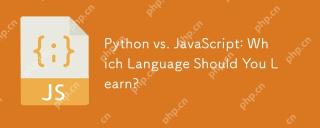
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
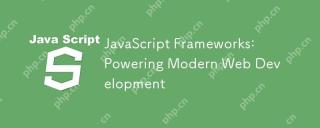
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
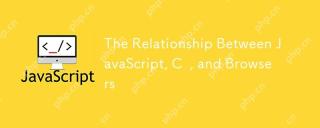
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
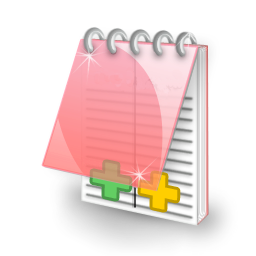
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
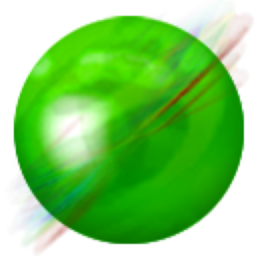
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
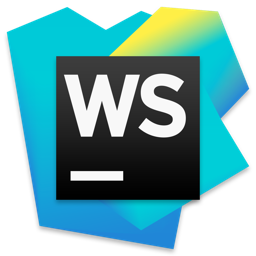
WebStorm Mac version
Useful JavaScript development tools
