Using CSS
In CSS, simply add the below definitions to the top of your page to customize the browser’s scrollbar colors. The great thing about CSS is that browsers that don’t understand it will just skip it. Scrollbar painting is supported by IE5.5 and up. Bet you never realized the scrollbar consisted of that many components! The first three definitions are the most important, as they correspond to the most visible aspects of the scrollbar. Feel free to play around with the other definitions to see what they affect.Using JavaScript
You can also use JavaScript to dynamically change the scrollbar color. This is useful when you wish to do something fancy, like alternating the scrollbar from one color to another. The JavaScript translation of the scrollbar CSS definitions are: document.body.style.scrollbarFaceColor="colorname" document.body.style.scrollbarArrowColor="colorname" document.body.style.scrollbarTrackColor="colorname" document.body.style.scrollbarShadowColor="colorname" document.body.style.scrollbarHighlightColor="colorname" document.body.style.scrollbar3dlightColor="colorname" document.body.style.scrollbarDarkshadowColor="colorname" Here’s an example of a “blinking” scrollbar, which changes color every second: <script> var mode=0 function blinkscroll(){ if (mode==0) document.body.style.scrollbarFaceColor=”blue” else document.body.style.scrollbarFaceColor=”green” mode=(mode==0)? 1 : 0 } setInterval(“blinkscroll()”,1000) </script> A more elaborate example of scrollbar manipulation using JavaScript, called onMouseover Scrollbar Effect, is written by Svetlin Staev. This changes the scrollbar colors when you move your mouse over and away from it. I’m seeing more and more sites customize the scrollbar color to blend in with the rest of their sites. I hope you find these tips useful in helping you do the same!Frequently Asked Questions about CSS and JavaScript Colors
How can I change the color of the scrollbar using CSS?
Changing the color of the scrollbar using CSS is quite simple. You can use the ::-webkit-scrollbar pseudo-element to select the scrollbar, and then apply your desired styles. Here’s an example:
::-webkit-scrollbar {
width: 10px;
}
::-webkit-scrollbar-track {
background: #f1f1f1;
}
::-webkit-scrollbar-thumb {
background: #888;
}
::-webkit-scrollbar-thumb:hover {
background: #555;
}
In this example, the scrollbar’s width is set to 10px, the track (the part that the handle slides along) is set to a light gray color, and the handle (or “thumb”) is set to a darker gray. When you hover over the handle, it changes to an even darker gray.
Can I use JavaScript to change the color of elements on a webpage?
Yes, you can use JavaScript to change the color of elements on a webpage. You can do this by accessing the style property of an element and then changing its color property. Here’s an example:
document.getElementById("myElement").style.color = "red";
In this example, the text color of the element with the id “myElement” is changed to red.
How can I use CSS to create a gradient color effect?
CSS provides a function called linear-gradient() that you can use to create a gradient color effect. Here’s an example:
background: linear-gradient(to right, red, orange, yellow, green, blue, indigo, violet);
In this example, the background of the element will be a gradient that transitions from red to violet, moving from left to right.
Can I use JavaScript to change the background color of an element?
Yes, you can use JavaScript to change the background color of an element. You can do this by accessing the style property of an element and then changing its backgroundColor property. Here’s an example:
document.getElementById("myElement").style.backgroundColor = "blue";
In this example, the background color of the element with the id “myElement” is changed to blue.
How can I use CSS to set the opacity of an element?
CSS provides a property called opacity that you can use to set the opacity of an element. Here’s an example:
.myElement {
opacity: 0.5;
}
In this example, the element with the class “myElement” will have its opacity set to 0.5, making it semi-transparent.
Can I use JavaScript to change the opacity of an element?
Yes, you can use JavaScript to change the opacity of an element. You can do this by accessing the style property of an element and then changing its opacity property. Here’s an example:
document.getElementById("myElement").style.opacity = "0.5";
In this example, the opacity of the element with the id “myElement” is changed to 0.5, making it semi-transparent.
How can I use CSS to set the border color of an element?
CSS provides a property called border-color that you can use to set the border color of an element. Here’s an example:
.myElement {
border-color: red;
}
In this example, the element with the class “myElement” will have its border color set to red.
Can I use JavaScript to change the border color of an element?
Yes, you can use JavaScript to change the border color of an element. You can do this by accessing the style property of an element and then changing its borderColor property. Here’s an example:
document.getElementById("myElement").style.borderColor = "red";
In this example, the border color of the element with the id “myElement” is changed to red.
How can I use CSS to set the text color of an element?
CSS provides a property called color that you can use to set the text color of an element. Here’s an example:
.myElement {
color: blue;
}
In this example, the element with the class “myElement” will have its text color set to blue.
Can I use JavaScript to change the text color of an element?
Yes, you can use JavaScript to change the text color of an element. You can do this by accessing the style property of an element and then changing its color property. Here’s an example:
document.getElementById("myElement").style.color = "blue";
In this example, the text color of the element with the id “myElement” is changed to blue.
The above is the detailed content of Manipulate Scrollbar Colors Using CSS and JavaScript Article. For more information, please follow other related articles on the PHP Chinese website!
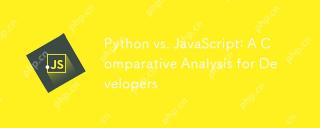
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
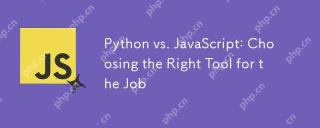
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
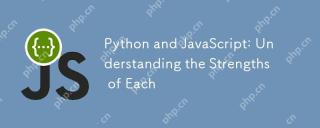
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
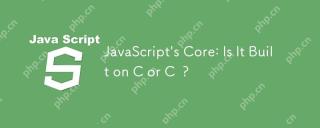
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
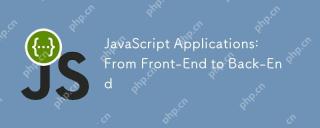
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
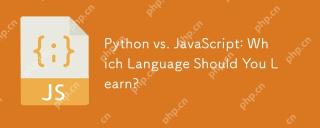
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
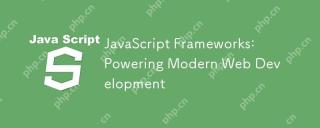
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
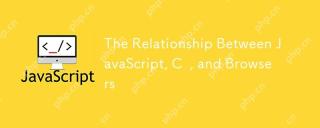
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment
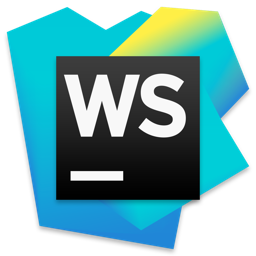
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Chinese version
Chinese version, very easy to use
