This jQuery code snippet synchronizes image alt
and title
attributes, enhancing SEO and browser compatibility. The script iterates through all images lacking a title
attribute, copying the alt
attribute's value to the title
attribute. If the alt
attribute is missing, it uses the image source (src
) as a fallback.
jQuery(document).ready(function($) { $("img:not([title])").each(function() { const altText = $(this).attr("alt"); const titleText = altText || $(this).attr("src"); $(this).attr("title", titleText); }); });
Frequently Asked Questions (FAQ) about jQuery, Image Titles, and Alt Tags
This FAQ section addresses common queries regarding using jQuery to manage image alt
and title
attributes for improved SEO and accessibility.
Q: How do I set image alt
and title
attributes using jQuery?
A: Use the .attr()
method:
$('img').attr({ 'alt': 'Alt text description', 'title': 'Title text for hover' });
This sets the alt
and title
attributes for all images. For more targeted updates, use more specific selectors.
Q: Why are alt
and title
tags important for SEO?
A: alt
text describes the image to search engines and screen readers, improving SEO and accessibility. The title
attribute provides additional context displayed on hover, enhancing the user experience. Both help search engines understand image content.
Q: How can I access the alt
attribute of an image within an <a></a>
tag?
A: Use .find()
to locate the image:
$('a').find('img').attr('alt');
This retrieves the alt
attribute of the image within the first <a></a>
tag found. Adjust the selector as needed.
Q: How do I replace an image's title
with its alt
attribute using jQuery?
A: Use .attr()
with a function:
$('img').attr('title', function() { return $(this).attr('alt'); });
This dynamically sets each image's title
to its alt
value.
Q: How do I add or modify alt
text for better SEO?
A: Use .attr()
to set or update the alt
attribute:
$('img').attr('alt', 'Descriptive alt text here');
Always use concise, accurate descriptions relevant to the image's context.
Q: How do I remove the alt
attribute using jQuery?
A: Use .removeAttr()
$('img').removeAttr('alt');
Use this cautiously, as removing alt
text negatively impacts accessibility.
Q: How do I add a title
attribute to an <a></a>
tag?
A: Use .attr()
jQuery(document).ready(function($) { $("img:not([title])").each(function() { const altText = $(this).attr("alt"); const titleText = altText || $(this).attr("src"); $(this).attr("title", titleText); }); });
This adds or modifies the title
attribute for all <a></a>
tags.
Q: How do I change an image's alt
attribute?
A: Use .attr()
$('img').attr({ 'alt': 'Alt text description', 'title': 'Title text for hover' });
This updates the alt
attribute for all images.
Q: How do I get an <a></a>
tag's title
attribute?
A: Use .attr()
$('a').find('img').attr('alt');
This retrieves the title
attribute of the first <a></a>
tag.
Q: How do I remove an <a></a>
tag's title
attribute?
A: Use .removeAttr()
$('img').attr('title', function() { return $(this).attr('alt'); });
Remember to use specific selectors if you need to target only particular images or links. Always prioritize accurate and descriptive alt
text for accessibility.
The above is the detailed content of jQuery Set Image TITLE from ALT Tag. For more information, please follow other related articles on the PHP Chinese website!
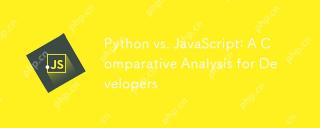
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
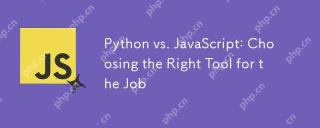
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
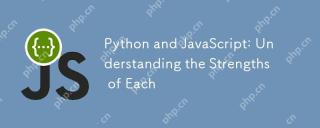
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
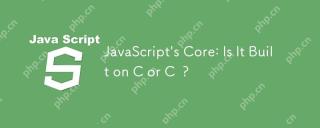
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
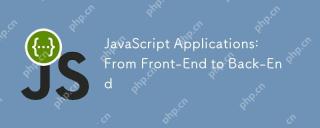
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
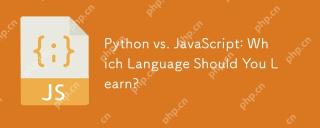
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
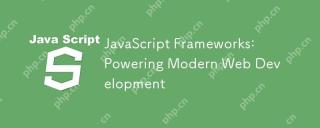
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
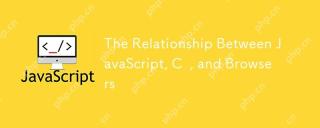
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
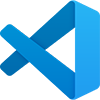
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
