jQuery Plug-in Development Guide: Creating Reusable Components
Core points:
- Create jQuery plug-in allows developers to create components that can be reused on any web page, reducing the risk of function name conflicts. The plug-in uses the
fn
function definition of jQuery. The method added to the jQuery library will pass the jQuery object as thethis
object of JavaScript. - When developing jQuery plug-ins, parameter processing is very important. To avoid complex parameter processing, pass a single JSON object instead of multiple parameters. You can use the
extend
function of jQuery to merge default parameters and user parameters. - Ensure that the method returns
this
to allow other functions to make chained calls, a key feature of jQuery called "chained calls". The plugin should be saved with the.js
extension and included in the HTML page after the jQuery library is loaded.
jQuery is the most popular JavaScript library, which many websites use to achieve dynamic effects and Ajax functionality. However, relatively few developers are delving into plugin development. This tutorial will create a simple plugin to explain its basics. Our code will invert text from one or more selected nodes – view the demo page.
Why create jQuery plugin?
In short: reuse. By extending jQuery, you can create components that can be reused on any web page. Your code is encapsulated and it is unlikely that you will use the same function name elsewhere.
How does jQuery work
In the core part, jQuery receives a DOM element or a string containing a CSS selector. It returns a jQuery object, which is an array-like collection of DOM nodes. Then one or more methods can be called in a chain to this group of nodes, for example:
// 将所有 <p> 标签的颜色设置为红色 $("p").css("color", "red");</p>
Note: Although the jQuery library is called "jQuery", "$" is a shortcut variable that references it. Note that other libraries may occupy "$".
How does jQuery plugin work
jQuery allows adding methods to its libraries. When these methods are called, the jQuery object is passed as a this
object of JavaScript. The DOM node can be operated as needed, and the method should return this
so that other functions can be called chained. Our sample plugin will be called using the following code:
// 反转所有 <p> 标签中的文本 $("p").reverseText();</p>
We will also add two optional parameters: minlength
and maxlength
. If these two parameters are defined, the string length must be between these two limits in order to be reversed.
Plugin Statement
Plugin uses jQuery's fn
function definition, for example:
// 将所有 <p> 标签的颜色设置为红色 $("p").css("color", "red");</p>
Using "jQuery" instead of "$" ensures that it does not conflict with other JavaScript libraries. All our internal code should also refer to "jQuery" instead of "$". However, we can use anonymous functions to save some input and reduce file size:
// 反转所有 <p> 标签中的文本 $("p").reverseText();</p>
This function runs immediately and passes jQuery as a parameter named "$". Since "$" is a local variable, we can assume that it always references the jQuery library, rather than the first other library to get the global "$" variable.
Plugin parameters
Our plugin requires two parameters: minlength
and maxlength
. The easiest way is to define them as function parameters, for example:
jQuery.fn.reverseText = function(params) { ... };
But what if we decide to add more parameters later? Our plugin may have dozens of options – parameter processing can quickly become complicated. As an alternative, we can pass a single JSON object, for example:
(function($) { $.fn.reverseText = function(params) { ... }; })(jQuery);The first line of the function should define a set of default parameters and then override the
parameters with any user-defined value reverseText
. jQuery's function can help us deal with this problem:
extend
(function($) { $.fn.reverseText = function(minlength, maxlength) { ... }; })(jQuery); // 示例 $("p").reverseText(0, 100);is 0 and
is 99999. params.minlength
params.maxlength
Now we can write our main plugin code:
Explanation:
(function($) { $.fn.reverseText = function(params) { ... } })(jQuery); // 示例 $("p").reverseText( { minlength: 0, maxlength: 100 } );
- Functions traverse all jQuery DOM nodes and call an anonymous function.
-
this.each
In the function, "this" contains a single node. A jQuery node collection is assigned to so that we can run the jQuery method. -
$t
Variable is assigned as a text string in the DOM node. - Set to an empty string.
origText
newText
If the length of is between - and
origText
, loop creates an inverted text string inparams.minlength
. Then update the DOM node accordingly.params.maxlength
newText
Finally, we should remember to return the jQuery object so that other methods can be called chained:
// 合并默认参数和用户参数 params = $.extend( {minlength: 0, maxlength: 99999}, params);Full code
Our plugin code is now completed:
Save this file as
// 遍历所有节点 this.each(function() { // 将单个节点表示为 jQuery 对象 var $t = $(this); // 查找文本 var origText = $t.text(), newText = ''; // 文本长度是否在定义的限制内? if (origText.length >= params.minlength && origText.length . We can then include the jQuery library in any HTML page after it is loaded, for example: <p> <code>jquery.reversetext.js</code> </p>The list in this page now reverses the text in the first and third bullets (remember, the first item starts with 0): <pre class="brush:php;toolbar:false">return this;
You should now have a good understanding of jQuery plug-in development. The SitePoint JavaScript forum is also a great resource for seeking help and advice. Coming soon: A new three-part tutorial on how to build useful page components into jQuery plugins. (The FAQ part in the original document is omitted here because the content of this part does not match the pseudo-original requirements and is too long.)
The above is the detailed content of How To Develop a jQuery Plugin. For more information, please follow other related articles on the PHP Chinese website!
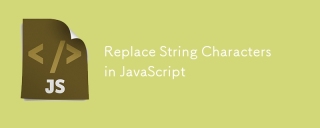
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
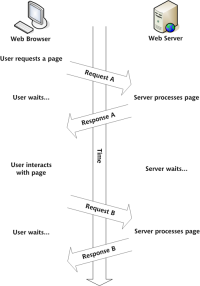
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
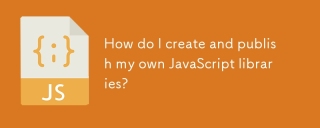
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.

The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
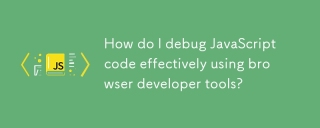
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
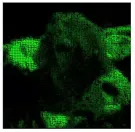
Bring matrix movie effects to your page! This is a cool jQuery plugin based on the famous movie "The Matrix". The plugin simulates the classic green character effects in the movie, and just select a picture and the plugin will convert it into a matrix-style picture filled with numeric characters. Come and try it, it's very interesting! How it works The plugin loads the image onto the canvas and reads the pixel and color values: data = ctx.getImageData(x, y, settings.grainSize, settings.grainSize).data The plugin cleverly reads the rectangular area of the picture and uses jQuery to calculate the average color of each area. Then, use
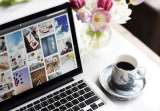
This article will guide you to create a simple picture carousel using the jQuery library. We will use the bxSlider library, which is built on jQuery and provides many configuration options to set up the carousel. Nowadays, picture carousel has become a must-have feature on the website - one picture is better than a thousand words! After deciding to use the picture carousel, the next question is how to create it. First, you need to collect high-quality, high-resolution pictures. Next, you need to create a picture carousel using HTML and some JavaScript code. There are many libraries on the web that can help you create carousels in different ways. We will use the open source bxSlider library. The bxSlider library supports responsive design, so the carousel built with this library can be adapted to any
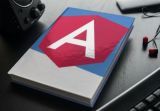
Data sets are extremely essential in building API models and various business processes. This is why importing and exporting CSV is an often-needed functionality.In this tutorial, you will learn how to download and import a CSV file within an Angular


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
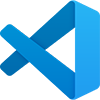
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
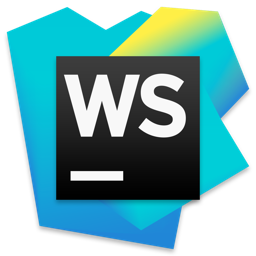
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor