MongoDB CRUD Operations: A Comprehensive Guide
This article answers your questions about performing CRUD (Create, Read, Update, Delete) operations in MongoDB, focusing on best practices, handling large datasets, and avoiding common pitfalls.
Performing CRUD Operations in MongoDB
MongoDB uses a document-oriented model, meaning data is stored in flexible, JSON-like documents. CRUD operations are performed using the MongoDB driver of your choice (e.g., Node.js driver, Python's pymongo, Java driver). Let's examine each operation:
-
Create (Insert): The
insertOne()
method inserts a single document into a collection.insertMany()
inserts multiple documents. For example, using the Python driver:
import pymongo myclient = pymongo.MongoClient("mongodb://localhost:27017/") mydb = myclient["mydatabase"] mycol = mydb["customers"] mydict = { "name": "John", "address": "Highway 37" } x = mycol.insert_one(mydict) print(x.inserted_id) #Prints the inserted document's ID mydocs = [ { "name": "Amy", "address": "Apple st 652"}, { "name": "Hannah", "address": "Mountain 21"}, { "name": "Michael", "address": "Valley 345"} ] x = mycol.insert_many(mydocs) print(x.inserted_ids) #Prints a list of inserted document IDs
-
Read (Find): The
find()
method retrieves documents. You can use query operators to filter results.findOne()
retrieves a single document.
myquery = { "address": "Mountain 21" } mydoc = mycol.find(myquery) for x in mydoc: print(x) mydoc = mycol.find_one(myquery) print(mydoc)
-
Update: The
updateOne()
method updates a single document.updateMany()
updates multiple documents. You use the$set
operator to modify fields.
myquery = { "address": "Valley 345" } newvalues = { "$set": { "address": "Canyon 123" } } mycol.update_one(myquery, newvalues) myquery = { "address": { "$regex": "^V" } } newvalues = { "$set": { "address": "updated address" } } mycol.update_many(myquery, newvalues)
-
Delete: The
deleteOne()
method deletes a single document.deleteMany()
deletes multiple documents.
myquery = { "address": "Canyon 123" } mycol.delete_one(myquery) myquery = { "address": { "$regex": "^M" } } x = mycol.delete_many(myquery) print(x.deleted_count)
Remember to replace "mongodb://localhost:27017/"
with your MongoDB connection string.
Best Practices for Optimal Performance
- Use Indexes: Indexes significantly speed up queries. Create indexes on frequently queried fields. Consider compound indexes for queries involving multiple fields.
-
Batch Operations: For inserting or updating many documents, use
insertMany()
andupdateMany()
to reduce the number of round trips to the database. -
Efficient Queries: Write concise and targeted queries. Avoid using
$where
clauses as they can be slow. Utilize appropriate query operators. - Data Modeling: Design your data model carefully. Consider denormalization to reduce joins and improve query performance if appropriate for your use case.
- Connection Pooling: Reuse database connections instead of creating a new connection for each operation. This reduces overhead.
- Appropriate Data Types: Choose the most efficient data type for each field (e.g., use integers instead of strings when appropriate).
Efficiently Handling Large Datasets
- Sharding: For extremely large datasets, shard your MongoDB cluster to distribute data across multiple servers.
- Aggregation Framework: Use the aggregation framework for complex data processing and analysis tasks on large datasets. It offers optimized operations for filtering, sorting, and grouping.
- Change Streams: Monitor changes in your data in real-time using change streams. This is helpful for building reactive applications that respond to data updates.
- Data Validation: Implement robust data validation to ensure data integrity and prevent the insertion of incorrect or malformed data.
Common Pitfalls to Avoid
-
Overuse of
$where
:$where
clauses can be significantly slower than other query operators. Avoid them whenever possible. - Ignoring Indexes: Failing to create appropriate indexes can lead to extremely slow query performance.
- Incorrect Data Modeling: A poorly designed data model can make queries inefficient and complex.
-
Unnecessary Data Retrieval: Retrieve only the necessary fields using projection (
{field1: 1, field2: 1}
) to reduce network traffic and improve performance. - Lack of Error Handling: Implement proper error handling to gracefully handle potential issues during CRUD operations.
- Ignoring Data Validation: Failing to validate data before insertion can lead to inconsistencies and errors in your database.
By following these best practices and avoiding common pitfalls, you can ensure efficient and reliable CRUD operations in your MongoDB applications, even when dealing with large datasets.
The above is the detailed content of How to add, delete, modify and search statements in mongodb. For more information, please follow other related articles on the PHP Chinese website!
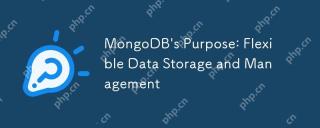
MongoDB's flexibility is reflected in: 1) able to store data in any structure, 2) use BSON format, and 3) support complex query and aggregation operations. This flexibility makes it perform well when dealing with variable data structures and is a powerful tool for modern application development.
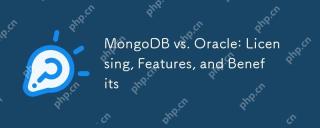
MongoDB is suitable for processing large-scale unstructured data and adopts an open source license; Oracle is suitable for complex commercial transactions and adopts a commercial license. 1.MongoDB provides flexible document models and scalability across the board, suitable for big data processing. 2. Oracle provides powerful ACID transaction support and enterprise-level capabilities, suitable for complex analytical workloads. Data type, budget and technical resources need to be considered when choosing.
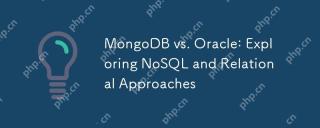
In different application scenarios, choosing MongoDB or Oracle depends on specific needs: 1) If you need to process a large amount of unstructured data and do not have high requirements for data consistency, choose MongoDB; 2) If you need strict data consistency and complex queries, choose Oracle.
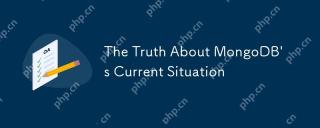
MongoDB's current performance depends on the specific usage scenario and requirements. 1) In e-commerce platforms, MongoDB is suitable for storing product information and user data, but may face consistency problems when processing orders. 2) In the content management system, MongoDB is convenient for storing articles and comments, but it requires sharding technology when processing large amounts of data.
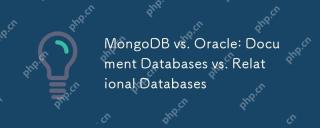
Introduction In the modern world of data management, choosing the right database system is crucial for any project. We often face a choice: should we choose a document-based database like MongoDB, or a relational database like Oracle? Today I will take you into the depth of the differences between MongoDB and Oracle, help you understand their pros and cons, and share my experience using them in real projects. This article will take you to start with basic knowledge and gradually deepen the core features, usage scenarios and performance performance of these two types of databases. Whether you are a new data manager or an experienced database administrator, after reading this article, you will be on how to choose and use MongoDB or Ora in your project
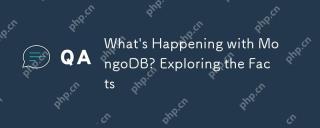
MongoDB is still a powerful database solution. 1) It is known for its flexibility and scalability and is suitable for storing complex data structures. 2) Through reasonable indexing and query optimization, its performance can be improved. 3) Using aggregation framework and sharding technology, MongoDB applications can be further optimized and extended.
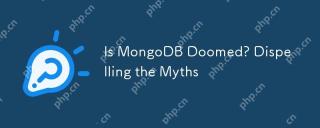
MongoDB is not destined to decline. 1) Its advantage lies in its flexibility and scalability, which is suitable for processing complex data structures and large-scale data. 2) Disadvantages include high memory usage and late introduction of ACID transaction support. 3) Despite doubts about performance and transaction support, MongoDB is still a powerful database solution driven by technological improvements and market demand.
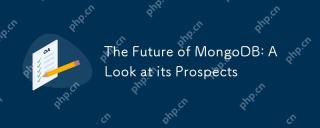
MongoDB'sfutureispromisingwithgrowthincloudintegration,real-timedataprocessing,andAI/MLapplications,thoughitfaceschallengesincompetition,performance,security,andeaseofuse.1)CloudintegrationviaMongoDBAtlaswillseeenhancementslikeserverlessinstancesandm


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
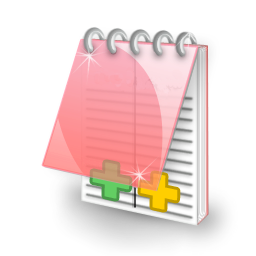
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
