UniApp Download File: How to Rename?
Renaming a downloaded file in UniApp requires a bit of a workaround since UniApp doesn't directly offer a file renaming feature within its download API. The core process involves downloading the file with a temporary name, then using the device's file system capabilities (via a plugin) to rename it after the download completes. This approach differs slightly depending on the platform (iOS, Android, H5).
For Android and iOS, you'll likely need a native plugin to interact with the file system. Popular choices include plugins that wrap the native file system APIs, allowing you to access file paths and perform operations like renaming. These plugins often provide functions similar to renameFile
or moveFile
that you can use after the download is finished.
For H5 (web), you have more limited control. You can't directly manipulate the file system on the client-side due to security restrictions. The best you can do is modify the suggested filename during the download process (if the browser allows it), which would effectively rename it on the client's device. However, the actual filename might still be different based on the browser's behavior.
The general process involves these steps:
-
Download with a temporary name: Download the file using UniApp's
uni.downloadFile
API, assigning a temporary filename (e.g., using a timestamp or UUID). -
Monitor download progress: Use the
uni.downloadFile
'ssuccess
callback to detect when the download is complete. - Use a native plugin (Android/iOS): If on Android or iOS, call the plugin's file renaming function, passing the temporary file path and the desired new file path.
- Handle potential errors: Implement error handling for cases where the download fails or the renaming operation fails (e.g., insufficient permissions).
- Inform the user: Provide feedback to the user about the download and renaming progress.
Example (Conceptual - requires a native plugin):
uni.downloadFile({ url: 'your_download_url', filePath: uni.env.USER_DATA_PATH + '/temp_' + Date.now() + '.zip', // Temporary filename success: function (res) { // Use a plugin to rename the file myPlugin.renameFile(res.filePath, uni.env.USER_DATA_PATH + '/my_renamed_file.zip', (err, success) => { if (err) { // Handle error } else { // Success } }); }, fail: function (err) { // Handle download error } });
How Can I Change the Filename of a Downloaded File in UniApp?
As explained above, directly changing the filename after download requires a native plugin for Android and iOS. For H5, influencing the filename is limited to providing a suggested filename during the download process; the browser may or may not use it. The process involves these key steps:
- Choosing a plugin: Select a suitable plugin from the UniApp marketplace or a platform-specific plugin repository. The plugin should provide file system access capabilities.
- Handling Permissions: Ensure you handle necessary file system permissions correctly, requesting them from the user if needed.
- Error Handling: Implement robust error handling to catch issues like permission denials, file access errors, or plugin malfunctions.
- User Feedback: Provide clear feedback to the user about the download and renaming progress.
What Are the Best Practices for Renaming Downloaded Files Within a UniApp Application?
Best practices for renaming downloaded files in UniApp revolve around robustness, user experience, and security:
- Use Unique Filenames: Employ a method to generate unique filenames, such as incorporating timestamps or UUIDs, to prevent overwriting existing files.
- Handle Errors Gracefully: Implement comprehensive error handling to manage situations where renaming fails (due to permissions, file existence, etc.). Provide informative error messages to the user.
- Use a Plugin Carefully: Thoroughly review the documentation and security implications of any native plugin used for file system access.
- User Permissions: Clearly request and handle necessary permissions (read/write access to the file system) from the user.
- Informative Feedback: Keep the user informed about the download and renaming process. Display progress indicators and success/failure messages.
- Avoid Sensitive Data: If dealing with sensitive data, ensure the plugin and file handling processes are secure and comply with relevant data protection regulations.
Is It Possible to Rename a Downloaded File Before It's Saved in UniApp?
No, you cannot directly rename a downloaded file before it's saved in UniApp using the standard uni.downloadFile
API. The API only allows you to specify the download path (filename). The renaming must occur after the download is complete, using a plugin to access the device's file system and rename the file at that location. Attempting to change the filename during the download process might be interpreted by the server or browser as an invalid request. Therefore, the temporary filename approach (as outlined previously) is necessary.
The above is the detailed content of How to rename UniApp download files. For more information, please follow other related articles on the PHP Chinese website!
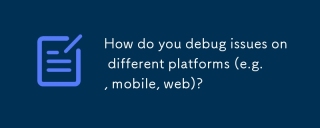
The article discusses debugging strategies for mobile and web platforms, highlighting tools like Android Studio, Xcode, and Chrome DevTools, and techniques for consistent results across OS and performance optimization.

The article discusses debugging tools and best practices for UniApp development, focusing on tools like HBuilderX, WeChat Developer Tools, and Chrome DevTools.

The article discusses end-to-end testing for UniApp applications across multiple platforms. It covers defining test scenarios, choosing tools like Appium and Cypress, setting up environments, writing and running tests, analyzing results, and integrat
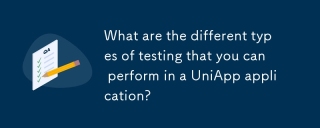
The article discusses various testing types for UniApp applications, including unit, integration, functional, UI/UX, performance, cross-platform, and security testing. It also covers ensuring cross-platform compatibility and recommends tools like Jes
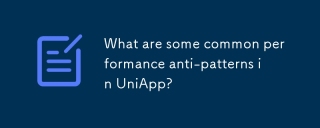
The article discusses common performance anti-patterns in UniApp development, such as excessive global data use and inefficient data binding, and offers strategies to identify and mitigate these issues for better app performance.
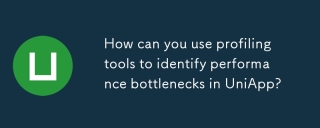
The article discusses using profiling tools to identify and resolve performance bottlenecks in UniApp, focusing on setup, data analysis, and optimization.
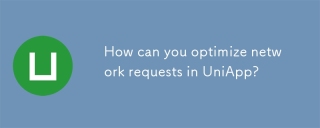
The article discusses strategies for optimizing network requests in UniApp, focusing on reducing latency, implementing caching, and using monitoring tools to enhance application performance.
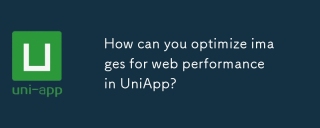
The article discusses optimizing images in UniApp for better web performance through compression, responsive design, lazy loading, caching, and using WebP format.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
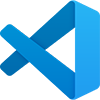
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
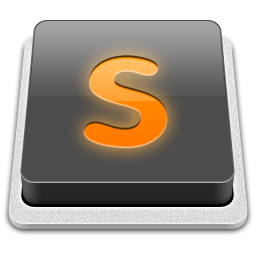
SublimeText3 Mac version
God-level code editing software (SublimeText3)