Handling Large File Downloads in UniApp: A Comprehensive Guide
This article addresses common challenges faced when downloading large files within the UniApp framework. We'll cover strategies for efficient downloads, progress display, error handling, and the use of third-party libraries.
UniApp Download File: How to Handle Large File Downloads?
Downloading large files in UniApp requires careful consideration to avoid application crashes or slowdowns. The standard uni.downloadFile
API is a good starting point, but it needs enhancements for managing large files. The key is to implement a mechanism that handles the download in chunks rather than trying to download the entire file at once. This approach minimizes memory usage and allows for better progress tracking.
Here's a basic example showcasing a chunked download approach (note: this is a conceptual example and requires adaptation based on your backend API):
uni.downloadFile({ url: 'your-large-file-url', header: { 'Accept': '*/*' // Add necessary headers }, success: function (res) { if (res.statusCode === 200) { //Handle success, perhaps save to file system console.log('Download complete:', res.tempFilePath); } else { //Handle error, such as non-200 status code console.error('Download failed with status code:', res.statusCode); } }, fail: function (err) { console.error('Download failed:', err); } });
However, for large files, you'll need to integrate a mechanism to handle the download in smaller chunks. This typically involves making multiple requests to your server, specifying a byte range for each request. Your server needs to be configured to support this range-based downloading (e.g., using HTTP headers like Range
and Content-Range
). The client then concatenates these chunks to form the complete file. This process is more complex and might require a custom solution or a well-suited third-party library.
How Can I Optimize UniApp for Downloading Large Files to Avoid Crashes or Slowdowns?
Optimizing UniApp for large file downloads focuses on minimizing resource consumption. The following strategies are crucial:
- Chunked Downloads: As mentioned above, breaking the download into smaller chunks drastically reduces memory usage and the risk of crashes.
-
Background Downloads: Use the
downloadTask
API (available in some UniApp versions) to perform downloads in the background. This prevents blocking the main thread and maintains app responsiveness. - Efficient File Saving: Avoid loading the entire downloaded file into memory. Instead, stream the data directly to the file system as it's received. UniApp's file system APIs provide mechanisms for this.
- Progress Tracking: Regularly monitor the download progress to provide feedback to the user and to detect potential issues early.
- Error Handling: Implement robust error handling to gracefully manage network issues, server errors, and interruptions. Retry mechanisms can significantly improve download reliability.
- Resource Management: Close unnecessary resources promptly to free up memory and prevent leaks.
- User Experience: Provide clear visual feedback to the user, indicating download progress and estimated time remaining. This improves the user experience and manages expectations.
What Are the Best Practices for Displaying Download Progress and Handling Potential Errors During Large File Downloads in UniApp?
Best practices for progress display and error handling involve:
- Progress Bar: Use a visually appealing progress bar to show the download progress. Update it regularly based on the received data.
- Percentage Calculation: Calculate and display the download percentage to give the user a clear indication of how far along the download is.
- Speed Indication: Optionally, display the download speed to provide more context.
- Error Messages: Provide informative error messages to the user in case of failures, clearly explaining the issue.
- Retry Mechanism: Implement automatic retry logic for transient network errors.
- Cancellation Option: Allow the user to cancel the download if needed.
- Pause/Resume: Consider adding pause and resume functionality for better user control.
- Logging: Log download events (start, progress, completion, errors) for debugging and monitoring.
Are There Any Third-Party Libraries or Plugins That Can Simplify Large File Downloads in UniApp?
While UniApp's built-in functionalities can be used, several third-party libraries can simplify the process of handling large file downloads. These libraries often provide features like:
- Chunked Download Management: Automated handling of chunked downloads and concatenation.
- Built-in Progress Tracking: Easy-to-use progress indicators.
- Advanced Error Handling: Robust error handling and retry mechanisms.
- Resume Capability: Support for resuming interrupted downloads.
Searching for "UniApp download manager" or "UniApp file download library" on platforms like npm (if applicable for your UniApp setup) might reveal suitable options. However, always carefully evaluate the library's security, performance, and compatibility before integrating it into your application. Remember to check the library's documentation for specific usage instructions within the UniApp environment. It's crucial to thoroughly test any third-party library to ensure it meets your requirements and integrates seamlessly with your application.
The above is the detailed content of How to handle downloading large files in UniApp. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
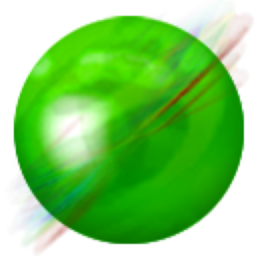
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
