


How to Implement a Web Touch Ripple Animation Effect Using JavaScript and CSS?
Implementing a touch ripple effect involves creating a dynamically generated element that expands from the point of touch. This is typically achieved using JavaScript to handle the touch event and CSS to style and animate the ripple. Here's a breakdown:
-
Event Handling (JavaScript): We use JavaScript to listen for the
touchstart
event. When a touch occurs, we create a new element (e.g., a<span></span>
or<div>) absolutely positioned within the target element. This new element represents the ripple. We then position this ripple element at the exact coordinates of the touch using the <code>touches[0].clientX
andtouches[0].clientY
properties from the touch event. -
Styling the Ripple (CSS): The CSS styles the ripple's appearance. Key properties include:
-
position: absolute;
: Ensures the ripple is positioned relative to its parent. -
background-color: ...;
: Sets the ripple's color. -
border-radius: 50%;
: Creates a circular ripple. -
transform: scale(...);
: Used in conjunction with animation to control the ripple's expansion. -
opacity: ...;
: Controls the ripple's fade-out effect.
-
-
Animation (CSS): CSS animations or transitions are used to create the expanding and fading effect. A
scale
animation increases the ripple's size, while anopacity
animation fades it out. The animation duration controls the speed of the ripple. We can use CSS variables (custom properties) to easily adjust these parameters. -
border-radius: 50%;
: This creates the perfectly circular shape characteristic of a ripple. -
transform: scale(x);
: This property is key for animating the expansion of the ripple. Thex
value is initially 0 and increases over time during the animation, making the ripple grow. -
opacity: x;
: This controls the ripple's transparency. Starting with an opacity of 1 (fully opaque) and animating it down to 0 (fully transparent) creates the fading effect as the ripple expands. -
animation
ortransition
: Eitheranimation
(for more complex animations) ortransition
(for simpler animations) are essential for creating the smooth expansion and fade-out of the ripple. Theduration
,timing-function
, andfill-mode
properties within these are crucial for fine-tuning the animation. -
position: absolute;
: This property allows precise positioning of the ripple within its parent container, based on the touch coordinates. -
box-shadow
(Optional): A subtlebox-shadow
can enhance the realism by adding a slight highlight or depth to the ripple. - Minimize DOM Manipulation: Creating and removing DOM elements frequently can impact performance. Consider techniques like object pooling or reusing elements instead of constantly creating new ones.
- Efficient Animations: Use CSS animations and transitions whenever possible, as they are hardware-accelerated and generally more performant than JavaScript-based animations. Keep the animation duration reasonable to avoid unnecessary processing.
- Limit Ripple Count: Don't create a ripple for every touch event if it's not necessary. Implement a strategy to limit the number of simultaneous ripples or to cancel previous ripples on a new touch.
- Optimize CSS: Avoid overly complex or computationally expensive CSS styles that could slow down rendering.
- Test Thoroughly: Test your implementation on various mobile devices and browsers to ensure smooth performance across different hardware capabilities.
-
Color: Modify the
background-color
property in your CSS to change the ripple's color. Using CSS variables makes it easier to change the color globally. For example: -
Size: Control the ripple's size by adjusting the
transform: scale(x);
value in your CSS animation or transition. A largerx
value results in a larger ripple. You can also dynamically adjust this value in JavaScript based on the target element's size. -
Duration: Change the
animation-duration
ortransition-duration
property in your CSS to adjust the ripple's lifespan. For example: - JavaScript Control: You can use JavaScript to dynamically change CSS variables or style properties, allowing for even more customized behavior based on user interaction or other factors. For example, you could adjust the ripple color based on the touched element's background color.
Here's a basic code example:
const container = document.getElementById('container'); container.addEventListener('touchstart', (event) => { const touchX = event.touches[0].clientX; const touchY = event.touches[0].clientY; const ripple = document.createElement('span'); ripple.classList.add('ripple'); ripple.style.left = `${touchX}px`; ripple.style.top = `${touchY}px`; container.appendChild(ripple); setTimeout(() => { container.removeChild(ripple); }, 1000); // Remove ripple after animation completes });
#container { position: relative; overflow: hidden; /* Important to contain the ripples */ } .ripple { position: absolute; background-color: rgba(0, 0, 255, 0.5); /* Example color */ border-radius: 50%; transform: scale(0); /* Initial state */ animation: rippleAnimation 1s ease-out forwards; /* Animation */ } @keyframes rippleAnimation { to { transform: scale(2.5); /* Final scale */ opacity: 0; /* Fade out */ } }
What CSS Properties Are Crucial for Creating a Realistic Ripple Animation on Touch?
Several CSS properties are vital for a realistic ripple:
Can I Achieve a Smooth and Performant Touch Ripple Effect with JavaScript and CSS on Mobile Devices?
Yes, you can achieve a smooth and performant touch ripple effect on mobile devices using JavaScript and CSS, but optimization is key. Here's how:
How Do I Customize the Appearance of a Touch Ripple Animation Created with JavaScript and CSS (e.g., color, size, duration)?
Customization is straightforward using CSS variables (custom properties) and JavaScript:
const container = document.getElementById('container'); container.addEventListener('touchstart', (event) => { const touchX = event.touches[0].clientX; const touchY = event.touches[0].clientY; const ripple = document.createElement('span'); ripple.classList.add('ripple'); ripple.style.left = `${touchX}px`; ripple.style.top = `${touchY}px`; container.appendChild(ripple); setTimeout(() => { container.removeChild(ripple); }, 1000); // Remove ripple after animation completes });
#container { position: relative; overflow: hidden; /* Important to contain the ripples */ } .ripple { position: absolute; background-color: rgba(0, 0, 255, 0.5); /* Example color */ border-radius: 50%; transform: scale(0); /* Initial state */ animation: rippleAnimation 1s ease-out forwards; /* Animation */ } @keyframes rippleAnimation { to { transform: scale(2.5); /* Final scale */ opacity: 0; /* Fade out */ } }
The above is the detailed content of How to use JavaScript and CSS to achieve web touch ripples animation effect?. For more information, please follow other related articles on the PHP Chinese website!
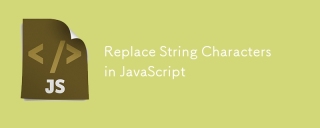
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
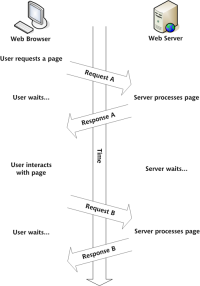
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
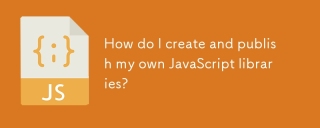
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
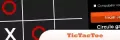
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
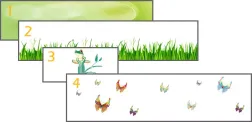
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the

The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
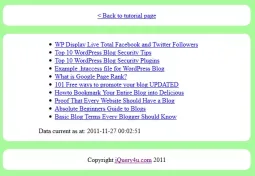
This article demonstrates how to automatically refresh a div's content every 5 seconds using jQuery and AJAX. The example fetches and displays the latest blog posts from an RSS feed, along with the last refresh timestamp. A loading image is optiona
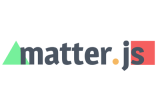
Matter.js is a 2D rigid body physics engine written in JavaScript. This library can help you easily simulate 2D physics in your browser. It provides many features, such as the ability to create rigid bodies and assign physical properties such as mass, area, or density. You can also simulate different types of collisions and forces, such as gravity friction. Matter.js supports all mainstream browsers. Additionally, it is suitable for mobile devices as it detects touches and is responsive. All of these features make it worth your time to learn how to use the engine, as this makes it easy to create a physics-based 2D game or simulation. In this tutorial, I will cover the basics of this library, including its installation and usage, and provide a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
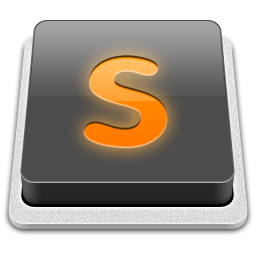
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment
