Get the JavaScript code snippet for the current date (dd/mm/yyyy)
The following JavaScript code snippet will get the current date and display it in dd/mm/yyyy format. The month will be displayed in two-digit format (for example, "04"). The code also demonstrates how to use jQuery to get a future date.
var fullDate = new Date(); console.log(fullDate); //Thu May 19 2011 17:25:38 GMT+1000 {} // 将月份转换为两位数字 var twoDigitMonth = ((fullDate.getMonth().length + 1) === 1) ? (fullDate.getMonth() + 1) : '0' + (fullDate.getMonth() + 1); var currentDate = fullDate.getDate() + "/" + twoDigitMonth + "/" + fullDate.getFullYear(); console.log(currentDate); //19/05/2011
Note: The console.log()
command is only used for debugging tools such as Firebug. If the above code is invalid, please try the following code (thanks pnilesh):
var fullDate = new Date(); console.log(fullDate); var twoDigitMonth = fullDate.getMonth() + ""; if (twoDigitMonth.length == 1) twoDigitMonth = "0" + twoDigitMonth; var twoDigitDate = fullDate.getDate() + ""; if (twoDigitDate.length == 1) twoDigitDate = "0" + twoDigitDate; var currentDate = twoDigitDate + "/" + twoDigitMonth + "/" + fullDate.getFullYear(); console.log(currentDate);
FAQs about jQuery and current dates (FAQ)
How to get the current date in jQuery?
jQuery itself does not have a built-in method to get the current date. However, you can use JavaScript's built-in Date
object to get the current date. Here is a simple example:
var currentDate = new Date(); console.log(currentDate);
This will return the current date and time. If you want to format this date in dd-mm-yyyy format, you can do this:
var day = currentDate.getDate(); var month = currentDate.getMonth() + 1; var year = currentDate.getFullYear(); var formattedDate = day + '-' + month + '-' + year; console.log(formattedDate);
What is the jQuery.now() method?
ThejQuery.now()
method is a utility function that returns the number of milliseconds elapsed since the Unix Era (00:00:00 UTC, January 1, 1970). It is essentially a wrapper for JavaScript Date.now()
methods. Here's how to use it:
var milliseconds = jQuery.now(); console.log(milliseconds);
How to format the current date using jQuery?
As mentioned earlier, jQuery does not have a built-in date handling function. However, you can use the Date
object of JavaScript with jQuery to format the current date. Here is an example:
var currentDate = new Date(); var day = ("0" + currentDate.getDate()).slice(-2); var month = ("0" + (currentDate.getMonth() + 1)).slice(-2); var year = currentDate.getFullYear(); var formattedDate = day + '-' + month + '-' + year; console.log(formattedDate);
Can I use jQuery to get the current date and time?
Yes, you can use jQuery to get the current date and time, but it is actually the JavaScript Date
object doing this. Here's how to do it:
var currentDate = new Date(); console.log(currentDate);
This will return the current date and time in the following format: Wed Sep 22 2021 10:30:15 GMT 0530 (Indian Standard Time)
How to use jQuery to get the current date, month and year respectively?
You can use the JavaScript Date
object to get the current date, month, and year respectively. Here's how to do it:
var currentDate = new Date(); var day = currentDate.getDate(); var month = currentDate.getMonth() + 1; var year = currentDate.getFullYear(); console.log('Day: ' + day); console.log('Month: ' + month); console.log('Year: ' + year);
This will return the current date, month, and year respectively.
The above is the detailed content of jQuery Get Todays Date dd/mm/yyyy. For more information, please follow other related articles on the PHP Chinese website!
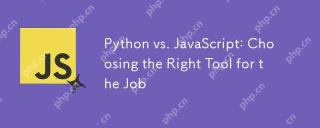
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
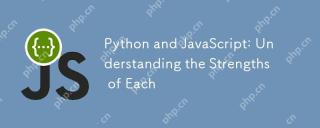
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
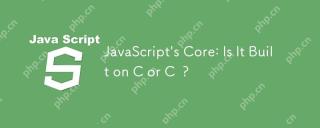
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
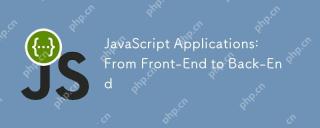
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
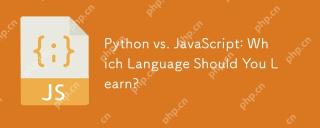
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
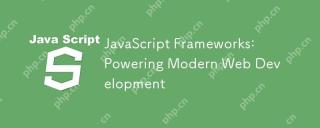
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
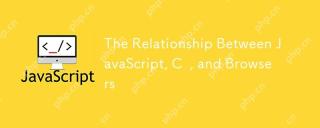
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
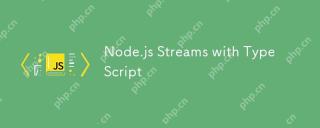
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
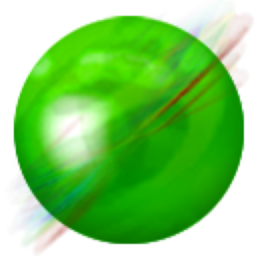
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
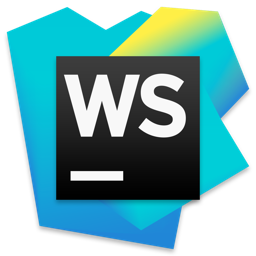
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
