Building Security Awareness in PHP 8
Security awareness is paramount when developing PHP 8 applications. It's not just about knowing the technical vulnerabilities; it's about cultivating a mindset that prioritizes security at every stage of the development lifecycle. This involves several key aspects:
- Education and Training: Developers need ongoing training on secure coding practices specific to PHP 8. This includes understanding common vulnerabilities like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). Regular security awareness training, perhaps including interactive modules and simulated phishing attacks, can significantly improve developers' understanding of potential threats.
- Security-focused Code Reviews: Implementing rigorous code reviews is crucial. Reviewers should specifically look for potential security flaws, using checklists and static analysis tools (discussed later). Peer review helps catch vulnerabilities before they reach production. The review process should be documented and tracked to ensure consistent application of security standards.
- Threat Modeling: Before starting development, conduct a threat modeling exercise. Identify potential threats and vulnerabilities specific to your application's functionality and architecture. This proactive approach helps prioritize security efforts and focus on the most critical areas.
- Security by Design: Security should not be an afterthought; it must be integrated into the design process from the outset. This includes choosing secure libraries and frameworks, implementing appropriate authentication and authorization mechanisms, and designing the application with security constraints in mind. This proactive approach is far more effective and cost-efficient than trying to fix vulnerabilities after the fact.
- Staying Updated: The threat landscape is constantly evolving. Developers need to stay informed about new vulnerabilities and security best practices through security advisories, newsletters, and industry conferences. Regularly updating PHP itself and all related libraries and frameworks is also essential.
Common Security Vulnerabilities in PHP 8 and How to Avoid Them
PHP 8, while improved in many aspects, still inherits some vulnerabilities from previous versions and introduces new potential weaknesses. Some common vulnerabilities include:
- SQL Injection: This occurs when user-supplied data is directly incorporated into SQL queries without proper sanitization. To prevent this, always use parameterized queries or prepared statements, never directly concatenating user input into SQL queries. Utilize database-specific escaping functions only as a last resort and with extreme caution.
-
Cross-Site Scripting (XSS): XSS attacks allow attackers to inject malicious scripts into web pages viewed by other users. Prevent this by properly encoding user-supplied data before displaying it on web pages, using functions like
htmlspecialchars()
and ensuring proper output encoding based on the context (HTML, JavaScript, etc.). Employ a robust Content Security Policy (CSP) to further mitigate XSS risks. - Cross-Site Request Forgery (CSRF): CSRF attacks trick users into performing unwanted actions on a website they're already authenticated to. Use CSRF tokens, which are unique, unpredictable values generated for each request and validated on the server-side, to prevent CSRF attacks.
- Session Hijacking: Attackers can steal user sessions, gaining unauthorized access. Use secure session handling techniques, including HTTPS, strong session IDs, and regular session timeouts. Avoid storing sensitive information in sessions.
- File Inclusion Vulnerabilities: Improper file inclusion can allow attackers to include malicious files, executing arbitrary code. Always use absolute paths when including files and validate user-supplied filenames before including them. Avoid dynamically constructing file paths based on user input.
- Insecure Deserialization: Deserializing untrusted data can lead to arbitrary code execution. Always validate and sanitize data before deserialization, and consider using safer alternatives if possible.
Implementing Secure Coding Practices in PHP 8 Applications
Secure coding practices should be integrated into every aspect of the development process. Here are some key practices:
- Input Validation and Sanitization: Always validate and sanitize all user inputs. This involves checking data types, lengths, formats, and ranges, as well as escaping special characters to prevent injection attacks.
- Output Encoding: Encode data appropriately based on the context where it's displayed (HTML, JavaScript, etc.) to prevent XSS attacks.
- Authentication and Authorization: Implement robust authentication mechanisms to verify user identities and authorization mechanisms to control access to resources based on user roles and permissions. Use strong password hashing techniques like bcrypt or Argon2.
- Error Handling: Handle errors gracefully and avoid revealing sensitive information in error messages. Log errors thoroughly for debugging and security analysis but don't expose sensitive details to the end-user.
- Least Privilege Principle: Grant users and processes only the necessary permissions to perform their tasks. This minimizes the impact of potential security breaches.
- Regular Security Audits and Penetration Testing: Conduct regular security audits and penetration testing to identify and address vulnerabilities.
- Use of Secure Libraries and Frameworks: Utilize well-maintained and secure libraries and frameworks like Symfony, Laravel, or Zend Framework. Keep them updated with the latest security patches.
- Secure Configuration: Securely configure your web server, database, and other components to minimize the attack surface. Disable unnecessary services and features.
Best Security Tools and Techniques for PHP 8 Development
Several tools and techniques can significantly enhance PHP 8 application security:
- Static Analysis Tools: Tools like Psalm, Phan, and SonarQube analyze code for potential vulnerabilities without executing it. They can identify common security flaws like SQL injection and XSS vulnerabilities.
- Dynamic Analysis Tools: Tools like RIPS and OWASP ZAP perform runtime analysis, identifying vulnerabilities during application execution.
- Security Linters: Linters like PHP CodeSniffer with security-focused rulesets can help enforce coding standards and detect potential security issues.
- Intrusion Detection/Prevention Systems (IDS/IPS): These systems monitor network traffic and application activity for malicious behavior.
- Web Application Firewalls (WAFs): WAFs filter malicious traffic before it reaches your application, protecting against common web attacks.
- Regular Security Updates: Keep your PHP version, libraries, frameworks, and operating system up-to-date with the latest security patches.
- Security Information and Event Management (SIEM): SIEM systems collect and analyze security logs from various sources, providing a centralized view of security events.
By implementing these security awareness measures, addressing common vulnerabilities, employing secure coding practices, and leveraging available security tools, developers can significantly improve the security posture of their PHP 8 applications. Remember that security is an ongoing process, requiring continuous learning, adaptation, and vigilance.
The above is the detailed content of How to build security awareness in PHP 8. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
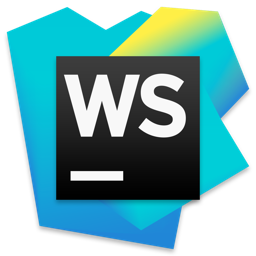
WebStorm Mac version
Useful JavaScript development tools
