Easy to convert strings into arrays with simple jQuery code snippets. Use jQuery's split()
method (similar to PHP's explode()
method) to split the words in a string into an array.
Sample code:
var numbersString = "1,2,3,4,5,6"; var numbersArray = numbersString.split(',');
You can then iterate through the values in the array like this:
$.each(numbersArray, function(index, value) { alert(index + ': ' + value); });
You can also reconcatenate array elements into strings:
var numbersArray = new Array("1", "2", "3", "4", "5", "6"); var numbersString = numbersArray.join(','); alert(numbersString); // 弹出 1,2,3,4,5,6
Remember to put your jQuery code inside the document ready
function. For more information, please refer to four different jQuery document ready
examples.
jQuery String to Array FAQ (FAQ)
How to convert a string to an array using jQuery?
Converting strings to arrays in jQuery is very simple. You can use the split()
method of JavaScript. This method splits the string into a substring array based on the specified splitter. Example:
var str = "Hello World"; var arr = str.split(" ");
In this example, the "Hello World" string is split into an array containing two elements: "Hello" and "World". The space character (" ") is used as a separator.
How to convert a string to an array without using a delimiter?
Yes, you can convert strings to arrays without specifying delimiters. In this case, each character in the string will become a single element in the array. The method is as follows:
var str = "Hello"; var arr = str.split("");
In this example, the "Hello" string is split into an array of five elements: "H", "e", "l", "l", and "o".
How to convert comma separated strings into arrays?
To convert a comma-separated string into an array, you can use the split()
method and use a comma (",") as the separator. Example:
var str = "apple,banana,cherry"; var arr = str.split(",");
In this example, the "apple,banana,cherry" string is split into an array containing three elements: "apple", "banana" and "cherry".
Can jQuery be used to convert strings into arrays?
jQuery is a powerful JavaScript library that simplifies HTML document traversal, event processing, animation, and Ajax interaction. However, it does not provide a dedicated method to convert strings into arrays. You can use JavaScript's split()
method for this purpose.
How to concatenate array elements into strings?
You can use the join()
method of JavaScript to combine array elements into a string. This method creates a new string by concatenating all elements of the array, separated by commas or specified splitter strings. Example:
var arr = ["Hello", "World"]; var str = arr.join(" ");
In this example, the array ["Hello", "World"] is concatenated into the string "Hello World". The space character (" ") is used as a separator.
How to convert a string to an array of integers?
Yes, you can convert strings to arrays of integers. However, you need to convert each string element into an integer using JavaScript's map()
method. Example:
var numbersString = "1,2,3,4,5,6"; var numbersArray = numbersString.split(',');
In this example, the "1,2,3" string is split into an array of integers: [1, 2, 3].
How to convert a string to a multidimensional array?
Converting a string to a multidimensional array involves splitting the string into substrings, and then splitting each substring into an array. Example:
$.each(numbersArray, function(index, value) { alert(index + ': ' + value); });
In this example, the "1-2-3,4-5-6,7-8-9" string is split into a multi-dimensional array: [[1, 2, 3], [4, 5, 6], [7, 8, 9]].
How to convert a string to an array of objects?
Yes, you can convert strings to an array of objects. However, this requires a more complex process. Example:
var numbersArray = new Array("1", "2", "3", "4", "5", "6"); var numbersString = numbersArray.join(','); alert(numbersString); // 弹出 1,2,3,4,5,6
In this example, the "name:John,age:30;name:Jane,age:25" string is divided into an array of objects: [{name: "John", age: "30"}, {name: "Jane", age: "25"}].
How to convert a string to an array using a regular expression?
You can use regular expressions as delimiters in the split()
method. This allows you to split strings into arrays based on complex patterns. Example:
var str = "Hello World"; var arr = str.split(" ");
In this example, the "apple banana-cherry" string is split into an array of three elements: "apple", "banana" and "cherry". Regular expression /[s-] / Match one or more spaces or hyphen characters.
How to convert strings to arrays in reverse order?
Yes, you can convert strings into arrays in reverse order. You can use the split()
method to convert the string to an array, and then use the reverse()
method to reverse the order of the elements. Example:
var str = "Hello"; var arr = str.split("");
In this example, the "Hello World" string is split into an array containing two elements: "World" and "Hello".
The above is the detailed content of jQuery Create Array From String. For more information, please follow other related articles on the PHP Chinese website!
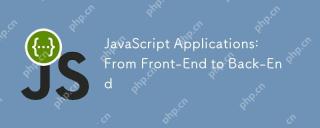
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
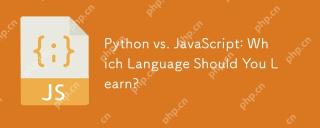
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
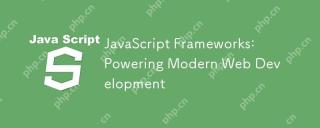
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
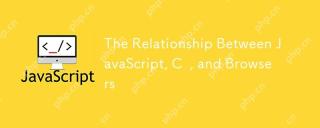
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
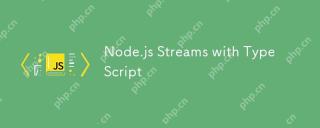
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
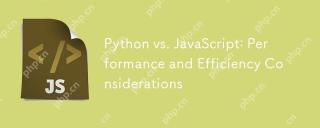
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
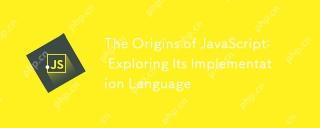
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
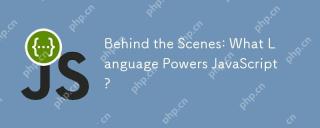
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use
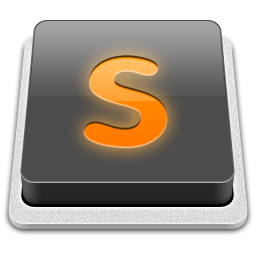
SublimeText3 Mac version
God-level code editing software (SublimeText3)
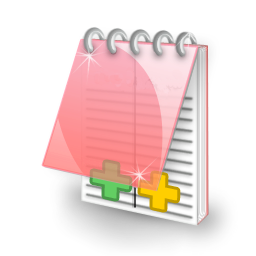
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
