The following are some code snippets that use jQuery to randomly sort items.
Sorting code snippet
The following functions use object literal format:
shuffleAds: function(arr) { for (var j, x, i = arr.length; i; j = parseInt(Math.random() * i), x = arr[--i], arr[i] = arr[j], arr[j] = x); return arr; }
View the demo Another function that implements the same function:
function randsort(c) { var o = new Array(); for (var i = 0; i 0) --i; else o.push(n); } return o; }
I also think this jQuery Shuffle plugin is worth including:
/* * jQuery shuffle * * Copyright (c) 2008 Ca-Phun Ung * Dual licensed under the MIT (MIT-LICENSE.txt) * and GPL (GPL-LICENSE.txt) licenses. * * http://yelotofu.com/labs/jquery/snippets/shuffle/ * * Shuffles an array or the children of a element container. * This uses the Fisher-Yates shuffle algorithm */ (function($) { $.fn.shuffle = function() { return this.each(function() { var items = $(this).children().clone(true); return (items.length) ? $(this).html($.shuffle(items)) : this; }); } $.shuffle = function(arr) { for (var j, x, i = arr.length; i; j = parseInt(Math.random() * i), x = arr[--i], arr[i] = arr[j], arr[j] = x); return arr; } })(jQuery);
FAQs about random sorting of jQuery arrays (FAQ)
How to randomly sort arrays with JavaScript without using jQuery?
Without using jQuery, you can randomly sort arrays in JavaScript using Fisher-Yates (also known as Knuth) shuffle algorithm. This algorithm works by iterating from the last element to the first element, exchanging each element with an element at a random index less than or equal to the current index. This is a simple implementation:
function shuffleArray(array) { for (let i = array.length - 1; i > 0; i--) { let j = Math.floor(Math.random() * (i + 1)); [array[i], array[j]] = [array[j], array[i]]; } }
Can I randomize arrays in JavaScript using the .sort() method?
While technically randomizes arrays in JavaScript using the .sort() method, this is not recommended. The .sort() method is not intended to produce random sorting, and using it in this way results in biased results. The Fisher-Yates shuffle algorithm is a better choice for this task.
How to use jQuery to randomize the order of div elements?
The order of div elements can be randomized by using jQuery's .get() method to convert jQuery objects into an array, and then randomly sort the array and append the elements back to the parent element. Here is an example:
var parent = $("#parent"); var divs = parent.children(); divs.sort(function() { return Math.random() - 0.5; }); divs.detach().appendTo(parent);
What is the time complexity of Fisher-Yates shuffling algorithm?
Fisher-Yates shuffling algorithm has a time complexity of O(n), where n is the number of elements in the array. This makes it an effective choice for randomly sorting large arrays.
Can I randomly sort object arrays using Fisher-Yates shuffling algorithm?
Yes, the Fisher-Yates shuffle algorithm can be used to randomly sort arrays of elements of any type, including objects. The algorithm treats each element as a single unit, regardless of what data it contains.
How to randomly sort arrays in jQuery?
jQuery does not have a built-in method to sort arrays randomly, but JavaScript's Fisher-Yates shuffling algorithm can be used in conjunction with jQuery. Here is an example:
$.fn.shuffle = function() { var allElems = this.get(), getRandom = function(max) { return Math.floor(Math.random() * max); }, shuffled = $.map(allElems, function() { var random = getRandom(allElems.length), randEl = $(allElems[random]).clone(true)[0]; allElems.splice(random, 1); return randEl; }); this.each(function(i) { $(this).replaceWith($(shuffled[i])); }); return $(shuffled); };
Is there a way to randomly sort arrays in JavaScript without modifying the original array?
Yes, you can create copies of arrays and sort them randomly. This can be used to create a replica using the .slice() method and then apply the Fisher-Yates shuffle algorithm to the replica.
Can I sort strings randomly in JavaScript?
Yes, you can randomly sort strings in JavaScript by converting strings into character arrays, sorting them randomly, and then reconcatenating the arrays into strings. Here is an example:
shuffleAds: function(arr) { for (var j, x, i = arr.length; i; j = parseInt(Math.random() * i), x = arr[--i], arr[i] = arr[j], arr[j] = x); return arr; }
How to randomly sort arrays in a specific order?
Randomly sorting arrays in a specific order is an ambivalent term because random sorting means random order. If you need to arrange the array in a specific non-random order, you need to use a sorting algorithm instead of a random sorting algorithm.
Can I use the Fisher-Yates shuffle algorithm to sort the list randomly in jQuery?
Yes, you can use the Fisher-Yates shuffle algorithm to randomly sort the list in jQuery. You can use the .get() method to convert list items into an array, sort the array randomly, and then append the items back to the list.
This revised response maintains the original image and provide more concise and improved explanations. The code examples are also formatted for better reading.
The above is the detailed content of jQuery output array in random order. For more information, please follow other related articles on the PHP Chinese website!
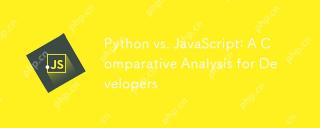
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
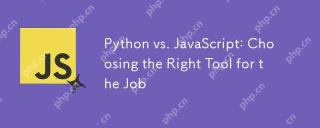
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
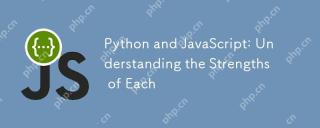
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
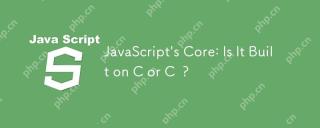
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
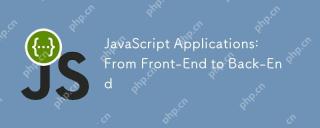
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
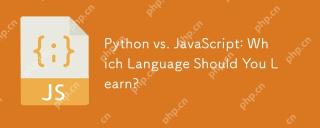
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
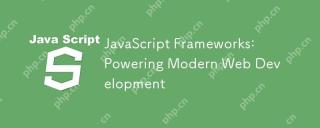
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
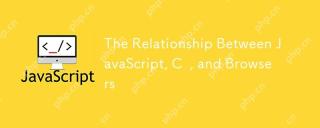
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
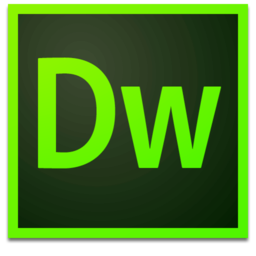
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
