Guide to using Traits in PHP 5.4
Core points
- The Traits mechanism introduced in PHP 5.4 allows horizontal reuse of code between independent classes of inheritance hierarchy, solving the limitations of single inheritance and reducing code duplication.
- Single class can use multiple Traits, and Traits can also be composed of other Traits, enabling a flexible and modular way of organizing code.
- Use the
insteadof
keyword to resolve conflicts between Traits with the same method name, or to create method alias using theas
keyword. - Traits can access private properties or methods of a composite class, and vice versa, and can even contain abstract methods that the composite class must implement.
An important goal of object-oriented programming is to minimize code duplication through better organization and code reuse. But in PHP, this can sometimes be difficult due to the limitations of the single inheritance model it uses; you may have some methods you wish to use in multiple classes, but they may not be well suited for the inheritance hierarchy. Languages like C and Python allow us to inherit from multiple classes, which solves this problem to some extent, while mixin in Ruby allows us to mix the functionality of one or more classes without using inheritance. However, multiple inheritance has problems such as Diamond Problem, and mixin is also a complex mechanism. In this article, I will discuss Traits, a new feature introduced in PHP 5.4 to overcome such problems. The concept of Traits itself is not new in programming and is used in other languages such as Scala and Perl. They allow us to horizontally reuse code between independent classes with different classes inheriting hierarchies.
Trait's appearance
Trait is similar to an abstract class that cannot be instantiated separately (although it is more often compared to an interface). The PHP document defines Traits as follows: > Traits is a mechanism for code reuse in single inheritance languages (such as PHP). The purpose of Traits is to reduce some of the limitations of single inheritance by enabling developers to freely reuse method sets in several independent classes (existing in different class inheritance hierarchies).
Let's consider this example:
<?php class DbReader extends Mysqli { } class FileReader extends SplFileObject { }
It would be a problem if both classes require some common functionality, such as making them both singletons. Since PHP does not support multiple inheritance, each class must implement the necessary code that supports singleton pattern, or there will be a meaningless inheritance hierarchy. Traits provides a solution to this type of problem.
<?php trait Singleton { private static $instance; public static function getInstance() { if (!(self::$instance instanceof self)) { self::$instance = new self; } return self::$instance; } } class DbReader extends ArrayObject { use Singleton; } class FileReader { use Singleton; }
Singleton Trait is implemented in a direct implementation of singleton pattern, with a static method getInstance()
that uses this Trait to create an object of the class (if not already created) and return it. Let's try to create objects of these classes using the getInstance()
method.
<?php class DbReader extends Mysqli { } class FileReader extends SplFileObject { }
We can see that $a
is an object of DbReader
and $b
is an object of FileReader
, but both now appear as singletons. The method from Singleton has been injected horizontally into the class that uses it. Traits does not impose any extra semantics on the class. To some extent, you can think of it as a compiler-assisted copy-paste mechanism where Trait's methods are copied into a composite class. If we just subclass $instance
from the parent class with a private DbReader
attribute, the attribute will not be displayed in the dump of ReflectionClass::export()
. However, with Traits, it's there!
<?php trait Singleton { private static $instance; public static function getInstance() { if (!(self::$instance instanceof self)) { self::$instance = new self; } return self::$instance; } } class DbReader extends ArrayObject { use Singleton; } class FileReader { use Singleton; }
Multiple Traits
So far, we have only used one Trait in one class, but in some cases we may need to merge the functionality of multiple Traits.
<?php $a = DbReader::getInstance(); $b = FileReader::getInstance(); var_dump($a); //object(DbReader) var_dump($b); //object(FileReader)
Here we have two Traits, Hello
and World
. Hello
Trait can only say "Hello", World
Trait can only say "World". In the MyWorld
class, we applied Hello
and World
so that the MyWorld
object will have methods from these two Traits and will be able to say "Hello World".
Traits composed of Traits
As the application grows, we will likely have a set of Traits used in different classes. PHP 5.4 allows us to have Traits composed of other Traits so that we only need to include multiple Traits in one Traits, rather than multiple Traits in all these classes. This allows us to rewrite the previous example as follows:
<code>Class [ class FileReader ] { @@ /home/shameer/workplace/php54/index.php 19-22 - Constants [0] { } - Static properties [1] { Property [ private static $_instance ] } - Static methods [1] { Method [ static public method instance ] { @@ /home/shameer/workplace/php54/index.php 6 - 11 } } - Properties [0] { } - Methods [0] { } }</code>
Here, we created the HelloWorld
Trait, used the Hello
and World
Traits, and included it in the MyWorld
. Since HelloWorld
Trait has methods from the other two Traits, it is exactly the same as if we included these two Traits ourselves in the class.
(The following content will be briefly summarized due to space limitations and retained core information)
Priority order: Trait method has a higher priority than the parent class method, and class method has a higher priority than the Trait method.
Conflict resolution and alias: Use insteadof
to select which Trait method to use, and use as
to create a method alias to avoid conflicts.
Reflection: ReflectionClass
getTraits()
Provides methods to obtain Traits information in a class, such as getTraitNames()
, isTrait()
, getTraitAliases()
, and
Other features:
Traits can access private properties and methods of a combined class, and vice versa; Traits can contain abstract methods, requiring the combined class to implement these methods; Traits cannot have a constructor, but can have a public initialization method.Summary:
Traits is one of the most powerful features introduced in PHP 5.4, and this article discusses almost all of its features. They allow programmers to reuse code snippets horizontally between multiple classes that do not have to be in the same inheritance hierarchy. They provide a lightweight code reuse mechanism rather than complex semantics. Although there are some drawbacks of Traits, they can certainly help improve the design of your application, eliminate code duplication, and make it more DRY.
(The FAQs part is omitted here due to the length of the article. Core information has been covered in the above content.)
The above is the detailed content of Using Traits in PHP 5.4. For more information, please follow other related articles on the PHP Chinese website!
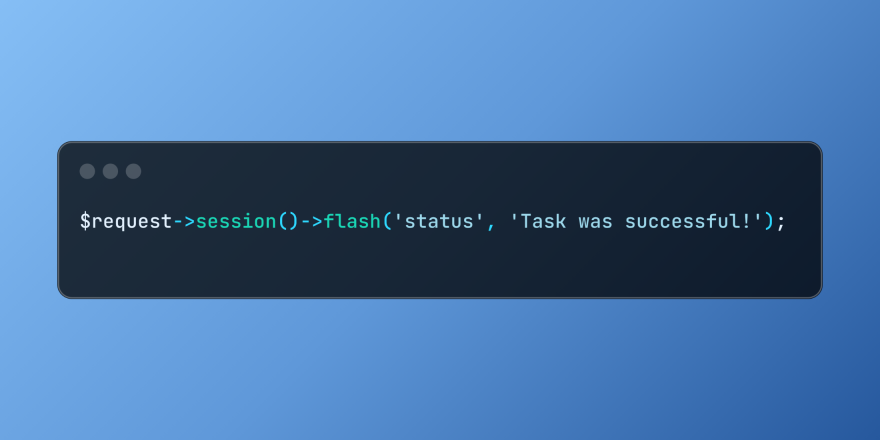
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
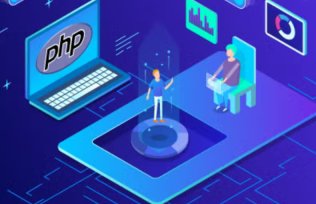
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
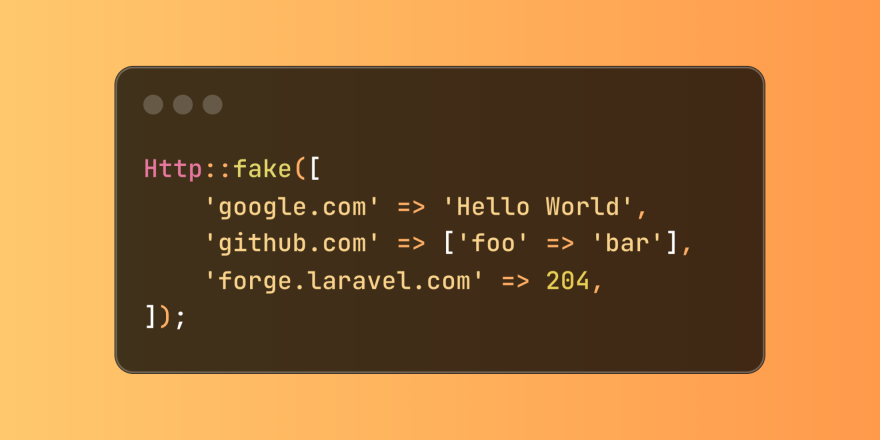
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
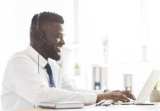
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
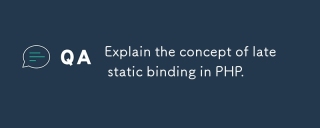
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
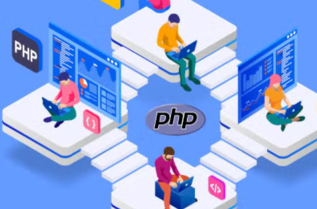
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
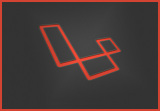
Laravel's service container and service providers are fundamental to its architecture. This article explores service containers, details service provider creation, registration, and demonstrates practical usage with examples. We'll begin with an ove
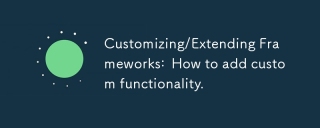
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
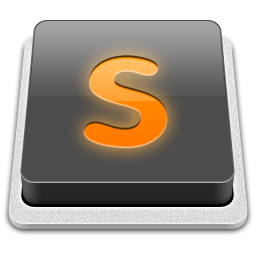
SublimeText3 Mac version
God-level code editing software (SublimeText3)
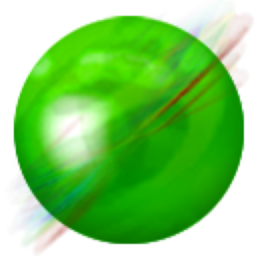
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!
