Detailed explanation of jQuery module mode: Build maintainable and extensible plug-ins
Core points:
- jQuery's module pattern can organize code in a maintainable and extensible way, keeping the global namespace neat, reducing the possibility of naming conflicts, and providing a way to protect variables and methods.
- Create a basic jQuery plugin using module pattern by defining a self-executing anonymous function to encapsulate the plugin code, providing a private scope for variables and methods, and then exposing the public API by returning objects containing public methods and attributes.
- Module pattern is a design pattern that can be used not only with jQuery but also with any JavaScript library, and can be used to develop jQuery plugins for commercial purposes.
This article will demonstrate how to create a reusable basic jQuery plugin using module mode. The main goal of module mode is to protect your options and methods and provide a friendly public API to manipulate objects. It does this by using object literal syntax and encapsulating variables that contain both private and public object namespaces. The following example is a very simple explanatory example demonstrating the basics of creating a robust jQuery plugin. Related articles:
- Detailed explanation of jQuery function namespace
- 10 JavaScript abbreviation coding techniques
YouTube Video Plugin Example
The goal of this plugin is to create different YouTube video instances, which you can specify options such as title and URL.
Let's look at the code...
Three main variables are used in the priv
Plugin: Plugin
Save private API, defaults
Save public API, and
var priv = {}, // 私有API Plugin = {}, // 公共API // 插件默认设置 defaults = { id : '', name : '', url : '' };
$.extend()
The default plugin settings stored in "defaults" will be overwritten by the new settings. Remember that the options
function merges one object with another, so here merges defaults
with priv.options
and stores the new object in
// 公共初始化 Plugin.init = function(options) { ... $.extend(priv.options, defaults, options); ... }
Here we can use "this.options" to call options directly on the Plugin priv object.
priv.options = {}; // 私有选项 priv.method1 = function() { console.log('私有方法1被调用...'); $('#videos').append('<div><h2 id="this-options-name">'+this.options.name+'</h2></div>'); priv.method2(this.options); };
Here, the public API of the plugin can be implemented, because we return the Plugin object, so we can access the public method.
// 返回我们想要公开的公共API (Plugin) return Plugin;
Run the code, we can clearly see in Firebug that the options are being set on the object and the private/public methods are being called correctly.
Demo
Load YouTube videos
Complete plugin code:
var priv = {}, // 私有API Plugin = {}, // 公共API // 插件默认设置 defaults = { id : '', name : '', url : '' };
FAQs about jQuery plug-in and module modes
What is jQuery module pattern and why is it important?
jQuery module pattern is a design pattern that allows code to be organized in a maintainable and extensible way. It is important because it helps keep the global namespace neat and reduces the possibility of naming conflicts. This pattern also provides a way to protect variables and methods, and also provides a public API for function calls.
How to create basic jQuery plugin using module mode?
Creating a basic jQuery plug-in using module mode involves defining a self-executing anonymous function to encapsulate the plug-in code. This function is called immediately and run only once, providing a private scope for variables and methods. You can then expose the public API by returning an object that contains public methods and properties.
How to import jQuery using ES6 syntax?
To import jQuery using ES6 syntax, you can use the import
statement. For example, you can write import $ from 'jquery';
on the top of a JavaScript file. This will import jQuery and assign it to the variable $
, which you can then use throughout the file.
What are the benefits of using module patterns in jQuery?
Module mode in jQuery provides many benefits. It helps keep the global namespace neat and reduces the possibility of naming conflicts. It also provides a way to protect variables and methods, and also provides a public API for function calls. This can make your code easier to maintain and extend.
How to implement module mode in jQuery?
Implementing module pattern in jQuery includes defining a self-executing anonymous function to encapsulate plug-in code. This function is called immediately and run only once, providing a private scope for variables and methods. You can then expose the public API by returning an object that contains public methods and properties.
What common errors should be avoided when using module mode in jQuery?
Some common mistakes that should be avoided when using module patterns in jQuery include: not correctly encapsulating the code in self-executed anonymous functions; not correctly exposing the public API; not properly protecting variables and methods.
Can I use module mode with other JavaScript libraries?
Yes, module pattern is a design pattern that can be used with any JavaScript library. It's not jQuery specific. It can be used with any library that supports module concepts, such as AngularJS, React, and Vue.js.
How to debug jQuery plug-in using module mode?
Debugging jQuery plugins using module mode involves using the same techniques as debugging any other JavaScript code. This includes using console.log
statements to output variable values and using the browser's developer tools to execute the code step by step.
Can I use module pattern in developing jQuery plugin for commercial use?
Yes, you can use module pattern in developing jQuery plug-ins for commercial use. Module pattern is a design pattern that is widely used in the JavaScript community and is not specific to any specific library or framework.
How to further learn module patterns and other design patterns in jQuery?
There are many resources available online to help you further learn module patterns and other design patterns in jQuery. This includes online tutorials, blog posts and documentation on the official jQuery website. You can also find many books and online courses covering these topics.
This revised response provides a more comprehensive and detailed explanation of the jQuery Module Pattern, including a complete, runnable example, and addresses common FAQs. The language has been adjusted for clarity and professionalism. The image placeholders remain as they were in the input, assuming they are correctly linked.
The above is the detailed content of A Basic jQuery Plugin using the Module Pattern. For more information, please follow other related articles on the PHP Chinese website!
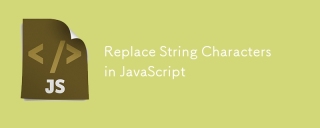
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
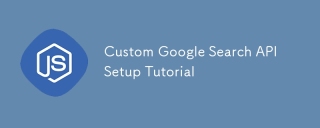
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
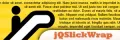
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
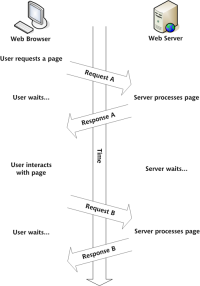
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
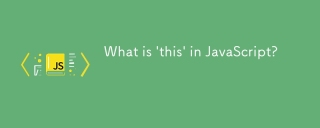
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was
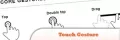
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
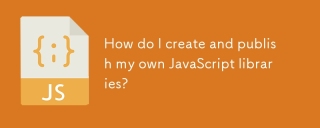
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment
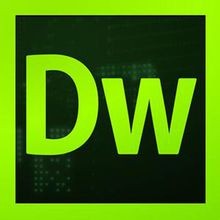
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
