Core points
- The exception handling of JavaScript is implemented through the "try…catch…finally" statement. The "try" clause contains code that may produce exceptions, the "catch" clause is executed when an exception occurs, and the "finally" clause will be executed regardless of the result.
- JavaScript has seven built-in error objects for exception handling: Error, RangeError, ReferenceError, SyntaxError, TypeError, URIError, and EvalError. Each object represents a specific type of error.
- JavaScript allows developers to throw their own exceptions using the "throw" statement. This can make the code easier to debug and maintain because it allows the creation of meaningful error messages.
- Can create custom exception types by extending the existing "Error" type. This allows for more specific and relevant error handling in complex applications.
In software development, Murphy's law also applies: anything that can go wrong will go wrong. For non-simple programs, the question is not whether will make an error, but when will make an error. Standard incompatible, unsupported features and browser features are just a few sources of potential problems faced by web developers. Given all the problems that may arise, JavaScript has a surprisingly simple error handling method - it just gives up and fails silently. At least, this is the behavior seen by the user. In fact, a lot of things happened behind the scenes. When a JavaScript statement produces an error, it is said that it throws an
exception. The JavaScript interpreter does not continue to execute the next statement, but checks for exception handling code. If there is no exception handler, the program will return from any function that throws the exception. This will repeat each function on the call stack until the exception handler is found or the top-level function is reached, causing the program to terminate. Error object
When an exception occurs, an object representing the error is created and thrown. The JavaScript language defines seven types of built-in error objects. These error types are the basis for exception handling. Each error type is described in detail below. Error
"Error" type is used to indicate a general exception. This exception type is most commonly used to implement user-defined exceptions. The topic of creating user-defined exceptions will be revisited later in this article. The "Error" object is instantiated by calling its constructor, as shown in the following example:
"Error" object contains two properties, "name" and "message". The "name" property specifies the exception type (in this case "Error"). The "message" property provides a more detailed description of the exception. "message" gets its value from the string passed to the exception constructor. The remaining exception types represent more specific error types, but they are used in the same way as the general "Error" type.
RangeError
When the number exceeds the specified range, a "RangeError" exception is generated. For example, JavaScript numbers have the toFixed() method, which takes the "digits" parameter to represent the number of digits that appear after the decimal point. This parameter should be between 0 and 20 (although some browsers support a wider range). If the value of "digits" is outside this range, a "RangeError" is thrown. The following example shows this.
var error = new Error("error message");
ReferenceError
When accessing non-existent variables, a "ReferenceError" exception will be thrown. These exceptions usually occur when there is an existing variable name that is misspelled. In the following example, a "ReferenceError" occurs when accessing "bar". Note that this example assumes that "bar" does not exist in any active scope when attempting to increment operations.
var pi = 3.14159; pi.toFixed(100000); // RangeError
SyntaxError
When JavaScript language rules are violated, a "SyntaxError" will be thrown. Developers familiar with languages such as C and Java are used to encountering syntax errors during compilation. However, since JavaScript is an interpreted language, syntax errors are not recognized until the code is executed. Syntax errors are unique because they are the only exception type that cannot be recovered from. The following example generates a syntax error because the "if" statement lacks the right brace.
function foo() { bar++; // ReferenceError }
TypeError
When the value is not the expected type, a "TypeError" exception occurs. Trying to call a non-existent object method is a common cause of such exceptions. The following example creates an empty object named "foo" and then tries to call its bar() method. Since bar() is not defined, a "TypeError" is thrown when trying to call it.
if (foo) { // SyntaxError // 缺少右花括号 }
URIError
When methods such as encodeURI() and decodeURI() encounter malformed URI, a "URIError" exception will be thrown. The following example generates a "URIError" when trying to decode the string "%". The "%" character indicates the beginning of the URI escape sequence. Since there is nothing after "%" in this example, the string is an invalid escape sequence and is therefore a malformed URI component.
var foo = {}; foo.bar(); // TypeError
EvalError
When the eval() function is not used, an "EvalError" exception will be thrown. These exceptions are not used in the latest version of the EcmaScript standard. However, in order to maintain backward compatibility with older version standards, they are still supported.
Exception handling
Now that we know what exceptions are, we should learn how to prevent them from causing the program to crash. JavaScript handles exceptions through the "try…catch…finally" statement. A common example statement is shown below.
decodeURIComponent("%"); // URIError
The first part of the "try...catch...finally" statement is the "try" clause. The "try" clause is required to separate blocks of code that programmers suspect may generate exceptions. The "try" clause must be followed by one or two "catch" and "finally" clauses.
"catch" clause
The second part of "try…catch…finally" is the "catch" clause. The "catch" clause is a block of code that is executed only when an exception occurs in the "try" clause. Although the "catch" clause is optional, it is impossible to really handle exceptions without it. This is because the "catch" clause prevents exceptions from propagating in the call stack, allowing program recovery. If an exception occurs in the "try" block, control is immediately passed to the "catch" clause. The exception that occurs is also passed to the "catch" block for processing. The following example shows how to use the "catch" clause to handle a "ReferenceError". Note that the "ReferenceError" object can be used in the "catch" clause through the "exception" variable.
var error = new Error("error message");
Complex applications can generate various exceptions. In this case, the "instanceof" operator can be used to distinguish various types of exceptions. In the following example, it is assumed that the "try" clause can generate several types of exceptions. The corresponding "catch" clause uses "instanceof" to handle the "TypeError" and "ReferenceError" exceptions separately from all other types of errors.
var pi = 3.14159; pi.toFixed(100000); // RangeError
"finally" clause
The last component of the "try…catch…finally" statement is the optional "finally" clause. The "finally" clause is a block of code executed after the "try" and "catch" clauses, regardless of whether an error occurs. The "finally" clause is useful for including cleaning code (closing files, etc.) that needs to be executed anyway. Note that the "finally" clause executes even if an uncaught exception occurs. In this case, the "finally" clause is executed, and the thrown exception continues normally.
An interesting note about the "finally" clause is that even if the "try" or "catch" clause executes the "return" statement, it will execute. For example, the following function returns false because the "finally" clause is the last executed content.
function foo() { bar++; // ReferenceError }
Top an exception
JavaScript allows programmers to throw their own exceptions through statements named "throw". This concept can be a bit confusing for inexperienced developers. After all, developers struggle to write code without errors, but the "throw" statement deliberately introduces errors. However, intentionally throwing exceptions can actually make the code easier to debug and maintain. For example, by creating meaningful error messages, it is easier to identify and resolve problems.
The following shows several examples of the "throw" statement. There is no limit on the data types that can be thrown as exceptions. There is also no limit on the number of times the same data can be captured and thrown. In other words, an exception can be thrown, caught, and then thrown again.
if (foo) { // SyntaxError // 缺少右花括号 }
While the "throw" statement can be used with any data type, using built-in exception types has some advantages. For example, Firefox specializes in these objects by adding debug information, such as file names and line numbers where exceptions occur.
For example, suppose that a division operation occurs somewhere in your application. Since there may be a situation where division by zero occurs, division can be troublesome. In JavaScript, such operations result in "NaN". This can lead to confusing results that are difficult to debug. If the app complains loudly about dividing by zero, the situation will be much simpler. The following "if" statement does this by throwing an exception.
var error = new Error("error message");
Of course, it may be more appropriate to use "RangeError", as shown below:
var pi = 3.14159; pi.toFixed(100000); // RangeError
Custom exception object
We just learned how to generate custom error messages using built-in exception types. However, another way is to create a new exception type by extending the existing "Error" type. Since the new type inherits from "Error", it can be used like other built-in exception types. Although this article does not discuss inheritance topics in JavaScript, a simple technique is introduced here.
The following example will return to the problem of handling the division by zero. Instead of using the "Error" or "RangeError" object as before, we want to create our own exception type. In this example, we are creating the "DivisionByZeroError" exception type. The function in the example acts as the constructor of our new type. The constructor is responsible for assigning the "name" and "message" attributes. The last two lines of the example allow the new type to inherit from the "Error" object.
function foo() { bar++; // ReferenceError }
Things to remember
- The "try…catch…finally" statement is used to handle exceptions.
- "try" clause identifies the code that may produce exceptions. The "catch" clause is only executed when an exception occurs.
- The "finally" clause is always executed, no matter what.
- The "throw" statement is used to generate an exception.
- Custom exception objects should inherit from the existing "Error" type.
- Pictures from Fotolia
JavaScript exception handling FAQ
What is the difference between syntax errors and runtime errors in JavaScript?
Syntax errors, also known as parsing errors, occur at compile time in traditional programming languages and at interpretation time in JavaScript. A syntax error occurs when the code is syntactically incorrect and cannot be parsed. For example, if you forget the closing bracket or use illegal characters, a syntax error occurs.
On the other hand, a runtime error occurs during program execution, after successful compilation. These errors are usually caused by illegal operations performed by the code. For example, trying to access an undefined variable or calling a function that does not exist can cause a runtime error.
How to handle exceptions in JavaScript?
JavaScript provides a try…catch statement to handle exceptions. The try block contains code that may throw exceptions, while the catch block contains code that will be executed if an exception is thrown in the try block. Here is a simple example:
var error = new Error("error message");
What is the purpose of the "finally" block in JavaScript?
The "finally" block in JavaScript is used to execute code after the try and catch blocks, regardless of the result. This means that the code in the "finally" block will be executed regardless of whether the exception is thrown or not. It is usually used to clean up after the code is executed, such as closing files or clearing resources.
Can I throw my own exception in JavaScript?
Yes, JavaScript allows you to throw your own exception using the "throw" statement. You can throw any type of exception, including strings, numbers, boolean values, or objects. Once an exception is thrown, the normal process of the program will be stopped and control will be passed to the most recent exception handler.
How to create a custom error type in JavaScript?
JavaScript allows you to create custom error types by extending the built-in Error object. This is useful when you want to throw a specific type of error in your code. Here is an example:
var pi = 3.14159; pi.toFixed(100000); // RangeError
What is the error propagation in JavaScript?
Error propagation refers to the process of passing an error up from where it was thrown to the nearest exception handler in the call stack. If no error is caught in the function that throws the error, it will be propagated upward to the calling function, and so on until it is captured or reached the global scope, at which point the program will terminate.
How to log errors in JavaScript?
JavaScript provides the console.error() method to log errors. This method works the same way as console.log() , but it also contains a stack trace in the log and highlights the message as an error in the console. Here is an example:
function foo() { bar++; // ReferenceError }
What is the difference between "null" and "undefined" in JavaScript?
In JavaScript, both "null" and "undefined" indicate missing values. However, they are used in slightly different contexts. "Undefined" means that a variable has been declared but has not been assigned a value. "null" on the other hand is an assignment value, indicating no value or no object.
How to handle asynchronous errors in JavaScript?
Asynchronous errors can be handled using Promise or async/await in JavaScript. Promise has a "catch" method that is called when Promise is rejected. With async/await you can use the try/catch block just like you would with synchronous code. Here is an example using async/await:
if (foo) { // SyntaxError // 缺少右花括号 }
What is TypeError in JavaScript?
When the operation cannot be performed, usually when the value is not the expected type, a TypeError is thrown in JavaScript. For example, attempting to call a non-function or access an undefined property will result in a TypeError.
The above is the detailed content of Exceptional Exception Handling in JavaScript. For more information, please follow other related articles on the PHP Chinese website!
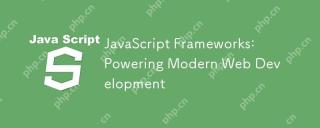
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
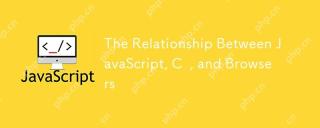
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
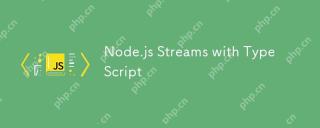
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
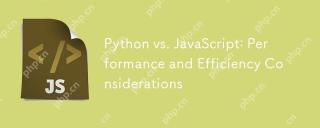
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
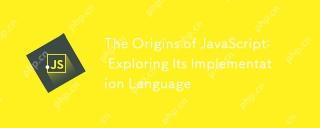
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
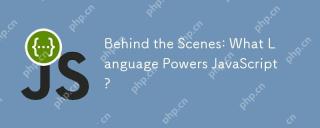
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
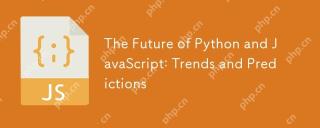
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
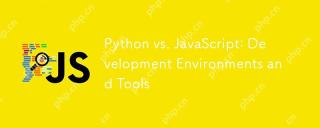
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
