The core functions of JavaScript are constantly being improved, and many new classes and functions have been added to assist programmers' work. However, some basic practical functions are still missing and need to be implemented with libraries such as jQuery, Prototype, and MooTools. While these tools are great, in some cases, using them seems a bit cumbersome. This article will introduce five practical functions that I think JavaScript should be built in.
Key points:
- JavaScript lacks some basic practical functions, which usually need to be implemented with libraries such as jQuery, Prototype, and MooTools. However, for some requirements, using these libraries is too cumbersome.
-
getElementsByClassName()
Functions are not supported by all versions of Internet Explorer, but wrapper functions can be used to be compatible with older versions. This function uses class names to retrieve elements, which is a feature that HTML5 did not have before. -
extend()
Functions are used to merge two or more objects, which is often required when writing plugins. This function is not a native JavaScript function, but can be easily built. -
inArray()
Functions are not native to JavaScript, but you can write this function to test whether a value is in an array. This function can be enhanced using theequals()
function to get a match when two objects have the same properties and values. -
toggleClass()
Function is used to add or delete an element's class name based on whether the class name exists.
getElementsByClassName()
JavaScript allows the use of getElementById()
functions to retrieve elements by their ID, but before HTML5, there was no native function that could use class names to get one or more elements. The new function is called getElementsByClassName()
, which is available in Firefox 3, Opera 9.5, Safari 3.1, and all versions of Google Chrome. Unfortunately, it is not supported by all versions of Internet Explorer, the number one enemy browser for web designers. Only Internet Explorer 9 supports getElementsByClassName()
, so for older versions, a wrapper function is required. The best function I found was written by Robert Nyman. His implementation was released under the MIT license and has been recommended by WHATWG. It uses the native getElementsByClassName()
method in browsers that support it, and then falls back to the lesser-known document.evaluate()
method, which is subject to older versions of Firefox (at least from version 1.5) and Opera (at least from version 9.27) Start) Support. If all methods fail, the script falls back to the recursive traversal of the DOM and collects elements that match the given class name. The code is as follows:
var getElementsByClassName = function (className, tag, elm){ // ... (代码与原文相同) ... };
extend()
If you've ever written a plugin, you've almost certainly been having problems merging two or more objects. This happens often when you have some default settings and want the user to be able to replace some default values. If you are using jQuery, you can use extend()
, but since we are talking about native JavaScript, the bad news is that there are no native functions. Fortunately, you can easily build it yourself. The following example will show you how to create code with the same functionality as the jQuery method. I added our extend()
method to the Object
prototype so that all objects can share the same method.
var getElementsByClassName = function (className, tag, elm){ // ... (代码与原文相同) ... };
equals()
Object comparison is a very common operation. While this test can be performed using the strict equality operator (===), sometimes you don't want to test whether two variables refer to the same object in memory. Instead, you want to know if two objects have the same properties and the same value. This is exactly what the following code does. Note that the following code was not written by me; it belongs to a user named crazyx. Again, equals()
has been added to Object.prototype
.
Object.prototype.extend = function() { // ... (代码与原文相同) ... };
inArray()
JavaScript There is no native method to test whether a value is in an array. We will write a function that returns true if the value exists, otherwise false. This function simply compares the given value to each element of the array. As with the previous two examples, inArray()
is added to the prototype properties of the Array
class.
Object.prototype.equals = function(x) { // ... (代码与原文相同) ... };
This function does not work as expected due to its simplicity. While it works for primitive types like strings and numbers, if you compare objects, it will only return true if the function finds the same object. To better understand how it works, let's look at the following example.
Array.prototype.inArray = function (value) { // ... (代码与原文相同) ... };
The improved inArray()
function is as follows:
// ... (代码与原文相同) ...
toggleClass()
Another function that is often used in jQuery is toggleClass()
. It adds or deletes the class name of the element based on whether the class name exists. A simple toggleClass()
version is shown below.
Array.prototype.inArray = function (value) { // ... (代码与原文相同) ... };
The improved toggleClass()
function is as follows:
function toggleClass(id, className) { // ... (代码与原文相同) ... }
Conclusion
This article focuses on some of the most important functions that I think are missing in JavaScript. Of course, JavaScript is missing something else, which we will see in the next few weeks. But, now, I want to point out the following points:
- Frameworks like jQuery have many useful functions, but they increase overhead. So if you only need a few functions, use native JavaScript and group the functions you need into an external file.
- If you use a function introduced in the new JavaScript version, don't delete it. Wrap it up with conditional statements to test if it is supported, and if it is not supported, use your old code as shown in
getElementsByClassName()
. This way, you will continue to support your old browser. - Add functions to the object's prototype as much as possible, as shown in
extend()
. All instances will share the same method and perform better. - Reuse your code as much as possible, as shown in the second version of
toggleClass()
.
The picture remains the original format and position unchanged.
The above is the detailed content of Five Useful Functions Missing in Javascript. For more information, please follow other related articles on the PHP Chinese website!
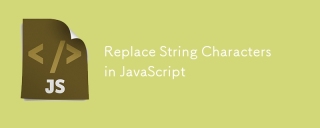
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
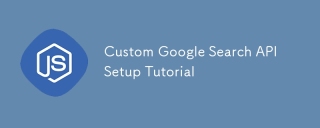
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
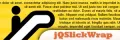
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
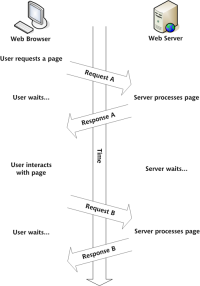
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
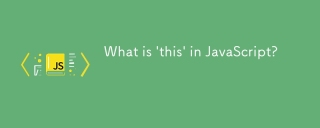
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was
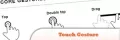
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
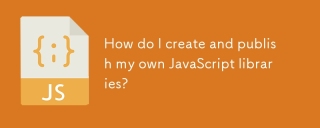
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
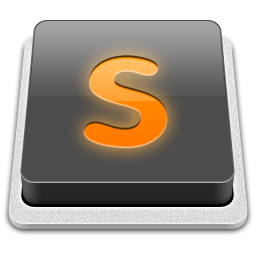
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
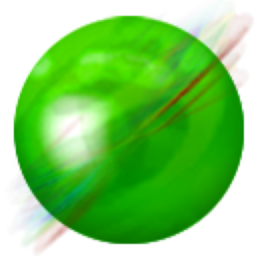
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 English version
Recommended: Win version, supports code prompts!
