Key Points
- jQuery.cookie, a jQuery plugin, simplifies the process of creating, reading and deleting cookies. It must be downloaded from the code base on GitHub and included in the page after the jQuery library. The
- cookie() method is used to create and read cookies. Creating a cookie requires two parameters: name and value. The optional third parameter can be an object literal containing additional options, such as path, domain, expires, and secure. To read the cookie, only the name parameter is required.
- Removing cookies is done using the removeCookie() method. If the cookie is found, it will return true, otherwise it will return false. The same options used when creating cookies (such as path and domain) must be passed in to successfully delete the cookies.
Cookies are common technologies for clients to store data. My previous article "How to Handle Cookies in JavaScript" explains how to perform CRUD operations on cookies using native JavaScript. This article turns to jQuery and will guide you through the jquery.cookie plugin, which makes cookie handling simple. This article assumes that readers are familiar with the contents of the aforementioned articles, or at least have a basic understanding of cookies. Without further ado, let's start.
Installing jquery.cookie
First, you need to download jquery.cookie from the code base on GitHub. Once you have obtained the jquery.cookie.js file, just add it to your page (s). Note that as a jQuery plugin you must include it after the jQuery library. Your page should contain a section similar to the following code:
<🎜> <🎜>
Method
jquery.cookie uses the same method cookie() to create and read cookies, but the number of parameters is different. To create a cookie, you need to pass in two required parameters, name and value of the cookie. You can pass a third optional parameter, which is an object literal with some additional options. These options are path, domain, expires, and secure. It is worth noting that these options can be set locally when you call the cookie() method, or globally through the $.cookie.defaults object. The options set with the former take precedence over the options set with the latter. To understand how cookies are created, let's look at a few examples. The following example tracks the number of times a user visits a website:
$.cookie("visits", 10);
This example stores the user's favorite cities and specifies the domain and path that the cookie can read and write to:
$.cookie("favourite-city", "London", {path: "/", domain: "jspro.com"});
This example stores the user's name. This cookie expired at 11:00 am on October 29, 2013 and can only be sent via a secure connection.
$.cookie("name", "Aurelio", {expires: new Date(2013, 10, 29, 11, 00, 00), secure: true});
Read Cookies
Reading cookies is very easy. You just need to pass in one parameter, namely the name of the cookie, and read it, as shown in the following example: Read the number of times a user visits the website:
console.debug($.cookie("visits")); // 打印 "10"
Read the user's favorite city:
console.debug($.cookie("favourite-city")); // 打印 "London"
Read user's name:
<🎜> <🎜>
Delete Cookies
Now you know how to create and read cookies. The last thing you need to know is how to delete a cookie using the removeCookie() method. Return true if the requested cookie is found, otherwise return false. Note that when you want to delete cookies, you need to pass in the same options, such as path and domain, otherwise the operation will fail. Now, let's look at several examples of the removeCookie() method. Delete cookies that store the number of visits to the site:
$.cookie("visits", 10);
Delete cookies that store users like cities:
$.cookie("favourite-city", "London", {path: "/", domain: "jspro.com"});
Next, we try to delete the cookie that stores the user's name. This example fails because the secure value is not specified.
$.cookie("name", "Aurelio", {expires: new Date(2013, 10, 29, 11, 00, 00), secure: true});
Conclusion
This article shows you how to manage cookies using jquery.cookie (a jQuery plugin). It solves many problems by abstracting cookie implementation details into several simple and flexible methods. If you need further instructions or other examples, please refer to the official documentation. If you like to read this article, you will love Learnable; there you can learn the latest skills and techniques from the masters. Members can instantly access all SitePoint's e-books and interactive online courses, such as jQuery: From Newbie to Ninja: New Tips and Tips. Comments in this article have been closed. Have questions about jQuery? Why not ask questions on our forum? *
FAQs about jQuery Cookies (FAQ)
How to set cookies using jQuery?
Setting cookies with jQuery is very simple. You can use the $.cookie function to set cookies. Here is an example:
$.cookie('cookie_name', 'cookie_value');
In this example, 'cookie_name' is the name of the cookie and 'cookie_value' is the value to be stored in the cookie. This will create a cookie that expires at the end of the browser session. If you want to set a specific expiration date, you can add the option object as the third parameter:
$.cookie('cookie_name', 'cookie_value', { expires: 7 });
This will create a cookie that expires after 7 days.
How to read cookies using jQuery?
It is also very easy to read cookies using jQuery. You can use the $.cookie function again, but this time without using the second parameter. Here is an example:
var cookie_value = $.cookie('cookie_name');
In this example, 'cookie_name' is the name of the cookie to be read. This function will return the value of the cookie.
How to delete cookies using jQuery?
To use jQuery to delete cookies, you can use the $.removeCookie function. Here is an example:
$.removeCookie('cookie_name');
In this example, 'cookie_name' is the name of the cookie to be deleted. This will delete cookies from the browser.
(The subsequent FAQ answer is similar to the previous output. The duplicate content is omitted here to keep the answer concise.)
The above is the detailed content of Working with Cookies in jQuery. For more information, please follow other related articles on the PHP Chinese website!
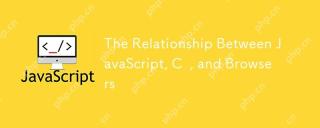
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
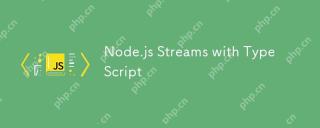
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
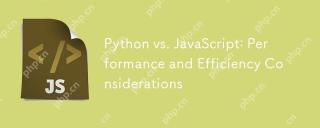
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
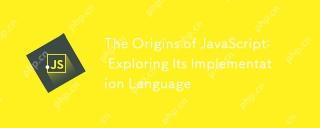
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
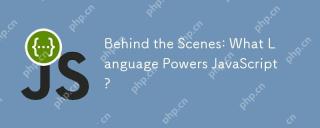
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
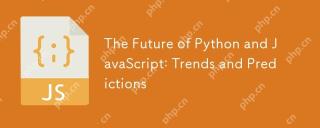
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
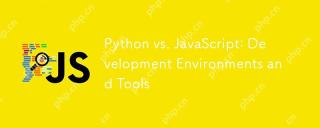
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
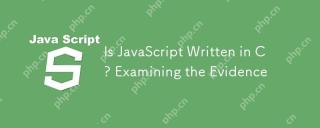
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor
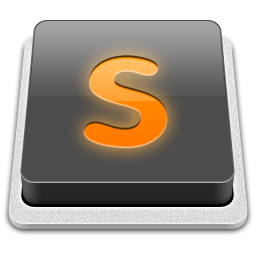
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
