Key Points
- Understanding JavaScript objects are essential for successful development in the language, as many built-in data types are represented as objects. An object is a composite data type built from primitives and other objects, and its properties describe aspects of an object.
- Objects can be created and accessed in JavaScript in a variety of ways. Object literal notation (in braces) allows for quick creation of objects with key/value pairs. Object properties can be accessed through dot notation or square bracket notation, which provides greater flexibility for variable attribute names or attribute names containing special characters.
- Functions used as objects' properties are called methods and can be called using point notation and square bracket notation. Attributes and methods can be added to an existing object through assignment statements, and properties of nested objects can be accessed by linking dots and/or bracket references together.
JavaScript objects are the cornerstone of the language. Many built-in data types (such as errors, regular expressions, and functions) are represented as objects in JavaScript. To be a successful JavaScript developer, you must have a firm grasp of how objects work. This article will teach you the basics of JavaScript object creation and manipulation. Objects are composite data types, built from primitives and other objects. An object's building block is often referred to as its field or attribute . Attributes are used to describe certain aspects of an object. For example, attributes can describe the length of the list, the color of the dog, or the date of birth of a person.
Create an object
Creating objects in JavaScript is easy. The language provides a syntax called object literal notation for quickly creating objects. The object text is represented in braces. The following example creates an empty object without attributes.
var object = {};
In braces, the attribute and its values are specified as a list of key/value pairs. The key can be a string or an identifier, while the value can be any valid expression. The list of key/value pairs is separated by commas, and each key and value is separated by a colon. The following example uses literal notation to create an object with three attributes. The first attribute foo stores the number 1. The second attribute bar is specified using a string and also stores the string value. The third property baz stores an empty object.
var object = { foo: 1, "bar": "some string", baz: { } };
Please pay attention to the use of spaces in the previous example. Each attribute is written on a separate line and indented. The entire object can be written on a single line, but the code written in this format is easier to read. This is especially true for objects with many properties or nested objects.
Access attributes
JavaScript provides two notations of accessing object properties. The first and most common one is called point notation. In dot notation, the property is accessed by giving the name of the host object, followed by a period (or dot), and then the property name. The following example shows how to read and write properties using point notation. If the initial stored value of object.foo is 1, its value will become 2 after executing this statement. Note that if object.foo does not have a value yet, it will be undefined.
var object = {};
Another syntax for accessing object properties is called square bracket notation . In square bracket notation, the object name is followed by a set of square brackets. In square brackets, the property name is specified as a string. The example of the previous point notation has been rewritten below to use square bracket notation. While the code may look different, it is functionally equivalent to the previous example.
var object = { foo: 1, "bar": "some string", baz: { } };
Square bracket notation is more expressive than dot notation because it allows variables to specify all or part of the attribute name. This is possible because the JavaScript interpreter automatically converts expressions in square brackets into strings and then retrieves the corresponding properties. The following example shows how to dynamically create attribute names using square bracket notation. In this example, the attribute name foo is created by concatenating the contents of the variable f with the string "oo".
object.foo = object.foo + 1;
Square bracket notation also allows attribute names to contain prohibited characters in dot notation. For example, the following statement is completely legal in square bracket notation. However, if you try to create the same property name in the dot notation, you will encounter a syntax error.
object["foo"] = object["foo"] + 1;
Access nested properties
The properties of nested objects can be accessed by linking dots and/or square bracket references together. For example, the following object contains a nested object named baz, which contains another object named foo, which has a property named bar that stores the value 5.
var f = "f"; object[f + "oo"] = "bar";
The following expression accesses nested property bar. The first expression uses dot notation, while the second expression uses square bracket notation. The third expression combines two notations to achieve the same result.
object["!@#$%^&*()."] = true;
Expressions like those shown in the previous examples may cause performance degradation if used incorrectly. It takes time to evaluate each point or square bracket expression. If you use the same property multiple times, it is best to access the property only once and then store the value in a local variable for use in all future purposes. The following example uses bar multiple times in a loop. However, instead of wasting time calculating the same value over and over, store bar in a local variable.
var object = { baz: { foo: { bar: 5 } } };
Function as method
When a function is used as an object property, it is called the method. Like properties, methods can also be specified in object literal notation. The following example shows how to achieve this.
object.baz.foo.bar; object["baz"]["foo"]["bar"]; object["baz"].foo["bar"];
Methods can also be called using dot notation and square bracket notation. The following example uses these two notations to call the sum() method in the previous example.
var object = {};
Add attributes and methods
Object literal notation is useful for creating new objects, but it cannot add properties or methods to existing objects. Fortunately, adding new data to an object is as simple as creating an assignment statement. The following example creates an empty object. Then use the assignment statement to add two attributes foo and bar and a method baz. Note that this example uses dot notation, but square bracket notation is equally effective.
var object = { foo: 1, "bar": "some string", baz: { } };
Conclusion
This article introduces the basic knowledge of JavaScript object syntax. It is crucial to master these contents because it forms the basis for the rest of the language. They say you have to learn to walk before you can run. Then, in the world of JavaScript, you must first understand objects to understand object-oriented programming.
Frequently Asked Questions about JavaScript Object Syntax (FAQ)
What is the difference between midpoint notation and square bracket notation in JavaScript object syntax?
In JavaScript, objects are collections of key-value pairs. You can access these values using dot notation or square bracket notation. The dot representation is more direct and easier to read. Use it when you know the property name. For example, if you have an object named "person" that has a property named "name", you can access it like this: person.name.
On the other hand, square brackets are more flexible. It allows you to access properties using variables or attribute names that may not be valid identifiers. For example, if the property name contains spaces or special characters, or it is a number, you can access it like this: person['property name'].
How to add properties to existing JavaScript objects?
You can add properties to an existing JavaScript object using dot notation or square bracket notation. For point notation you just need to use the syntax object.property = value. For square bracket notation, the syntax is object['property'] = value. In both cases, if the property does not exist in the object, it is added.
How to delete attributes from JavaScript objects?
You can use the "delete" operator to delete properties from JavaScript objects. The syntax of the “delete” operator is delete object.property for point notation and delete object['property'] for square bracket notation. This will remove the attribute and its value from the object.
What are the methods in JavaScript objects?
Themethod is a function stored as an object property. They are used to perform operations that utilize object data. You can define methods in an object using function syntax as follows: object.methodName = function() { / code / }.
How to iterate over properties of JavaScript objects?
You can use the "for...in" loop to iterate over properties of JavaScript objects. This loop will iterate over the object's so enumerable properties, including properties inherited from the prototype chain. The syntax is as follows: for (var property in object) { / code / }.
What is the "this" keyword in a JavaScript object?
The "this" keyword in a JavaScript object refers to the object to which it belongs. Inside the method, "this" refers to the owner object. In the constructor, "this" refers to the newly created object.
What is the constructor in JavaScript?
Constructors in JavaScript are special functions used to create objects of the same type. They are named in capital letters to distinguish them from ordinary functions. The "new" keyword is used to call the constructor and create a new object.
What is the object prototype in JavaScript?
Each JavaScript object has a prototype. A prototype is also an object, and all objects inherit properties and methods from its prototype. This is a powerful feature of JavaScript because it allows you to add new properties or methods to instances of object types.
How to check if there are properties in JavaScript objects?
You can use the "in" operator or the "hasOwnProperty" method to check whether properties exist in a JavaScript object. The "in" operator returns true if the property exists in an object or its prototype chain. The "hasOwnProperty" method returns true only if the property exists in the object itself.
What is object destruction in JavaScript?
Object destruction in JavaScript is a function that allows you to extract properties from objects and bind them to variables. This can make your code more concise and easy to read. The syntax is as follows: var { property1, property2 } = object.
The above is the detailed content of Object Syntax in JavaScript. For more information, please follow other related articles on the PHP Chinese website!
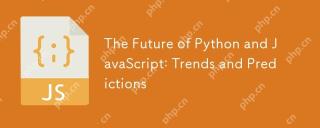
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
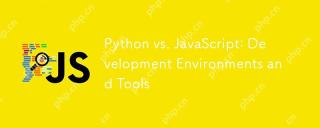
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
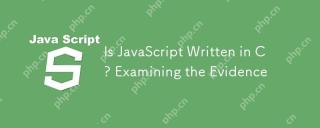
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
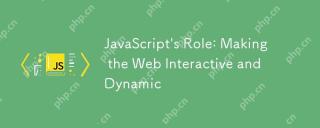
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
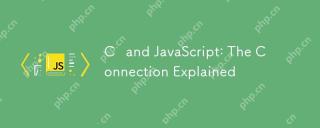
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
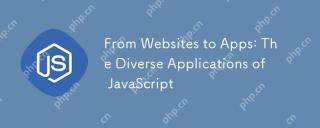
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
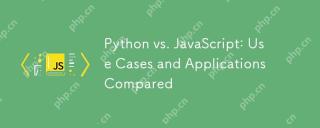
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
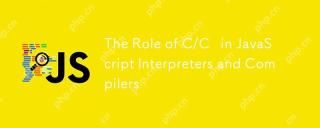
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
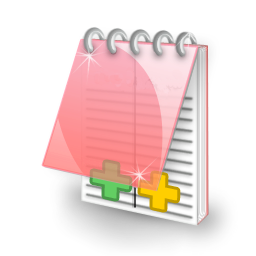
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
