Vanilla JavaScript loops vs. jQuery.each: A Performance Comparison
This article explores the performance differences between using vanilla JavaScript for
loops and jQuery's $.each
method for array iteration. We'll demonstrate that, for speed, vanilla for
loops, especially those with variable caching, significantly outperform $.each
. This can result in speed improvements of up to 84%, as shown in jsperf benchmarks (link omitted for brevity, but easily searchable).
jQuery.each Example:
$.each(myArray, function() { let currentElement = this; // ... your code using currentElement ... });
For Loop with Variable Caching (Fastest):
const len = myArray.length; for (let i = 0; i < len; i++) { let currentElement = myArray[i]; // ... your code using currentElement ... }
For Loop without Variable Caching:
for (let i = 0; i < myArray.length; i++) { let currentElement = myArray[i]; // ... your code using currentElement ... }
Pre-calculated Length Attempt: (Similar performance to the cached version)
let len = myArray.length; let i = 0; for (; i < len; i++) { let currentElement = myArray[i]; // ... your code using currentElement ... }
Frequently Asked Questions (FAQs):
While this article focuses on performance, here's a summary of key differences and considerations for choosing between $.each
and for
loops:
-
Functionality: jQuery's
$.each
iterates over arrays and objects, offering a concise syntax.for
loops provide more direct control over iteration. -
Performance:
for
loops (especially with variable caching) are generally faster due to reduced function call overhead. The difference becomes more pronounced with larger datasets. -
Readability:
$.each
can improve code readability for simple iterations.for
loops are more explicit. -
Breaking the Loop: Returning
false
from the$.each
callback breaks the loop.for
loops usebreak
. -
Index Access: Both provide access to the current index (though differently).
-
NodeList/HTMLCollection:
$.each
works with NodeLists and HTMLCollections, butthis
refers to a DOM element, not a jQuery object. Wrap with$(this)
to use jQuery methods. -
Native
forEach
: JavaScript's nativeforEach
offers similar syntax to$.each
but with potentially better performance than jQuery's implementation. -
Object Iteration: Both can iterate over objects.
-
Sparse Arrays:
$.each
skips undefined indices in sparse arrays, whilefor
loops include them. -
Chaining:
$.each
doesn't support chaining like other jQuery methods.
In summary, for optimal performance, especially when dealing with large datasets, prioritize vanilla JavaScript for
loops with variable caching. jQuery's $.each
is more convenient for smaller datasets or when readability is paramount. Consider JavaScript's native forEach
as a faster alternative to jQuery's $.each
.
The above is the detailed content of Speed Question jQuery.each vs. for loop. For more information, please follow other related articles on the PHP Chinese website!
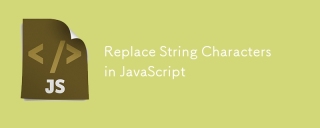
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
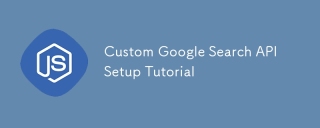
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
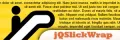
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
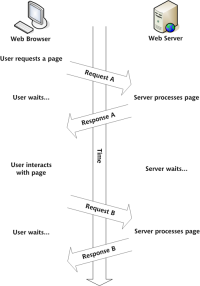
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
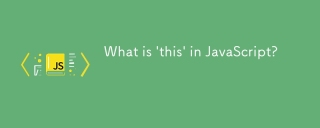
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
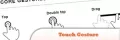
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig
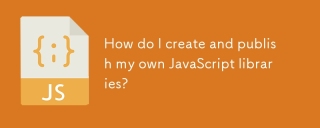
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
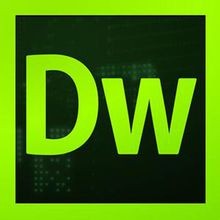
Dreamweaver CS6
Visual web development tools
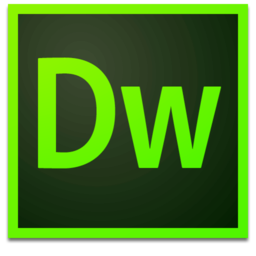
Dreamweaver Mac version
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment
