Core points
-
requestAnimationFrame
is a helper function that is used to write animations synchronized with the browser's drawing cycle, thereby achieving smoother and more CPU-saving animation effects. It is supported by all modern browsers and is compatible with older browsers. - The
requestAnimationFrame
functions created usinganimate
can be designed to accept a series of functions as parameters, which are called in sequence, thereby implementing an animation sequence. This function can track the progress of the animation and calculate the end time of the animation. -
requestAnimationFrame
creates animations more efficiently thansetTimeout
orsetInterval
because it works synchronously with the browser's refresh rate. It can be used with any JavaScript framework or library and can handle a variety of animations, including CSS properties, canvas animations, SVG animations, and scrolling or user interaction-based animations.
DOM element animation involves modifying the CSS style every few milliseconds to create the illusion of motion. This means passing the callback function to setTimeout
and modifying the style object of the node in that callback function. Then call setTimeout
again to queue up the next animation frame. requestAnimationFrame
This new helper function came into being for animation. It was initially seen in Firefox 4 and has gradually been adopted by all browsers, including IE 10. Fortunately, it's easy to make it compatible with older browsers.
window.requestAnimationFrame(callbackFunction);
Unlike setTimeout
(run after a specified time delay), requestAnimationFrame
runs the callback function the next time the browser draws the screen. This allows you to sync with the browser's drawing cycle so that you don't draw too often or not frequently enough, which means your animation will be very smooth and not overload the CPU.
Browser compatibility processing
Currently every browser has a prefixed version of requestAnimationFrame
, so let's detect which version is supported and reference it:
var _requestAnimationFrame = function(win, t) { return win["webkitR" + t] || win["r" + t] || win["mozR" + t] || win["msR" + t] || function(fn) { setTimeout(fn, 60) } }(window, "equestAnimationFrame");
Note how we use square bracket notation to access properties on window objects. We use square bracket notation because we are dynamically building property names using string concatenation. If the browser does not support it, we will fall back to a regular function that calls setTimeout
after 60 milliseconds to achieve a similar effect.
Animation function construction
Now let's build a simple function that will repeatedly call our _requestAnimationFrame
to simulate the animation. To implement animation, we need an external function as an entry point and an internal function (called a step function) that will be called repeatedly.
window.requestAnimationFrame(callbackFunction);
Every time we call the step function, we need to track the progress of the animation to know when it ends. We will calculate the time when the animation should end and calculate the progress based on the time remaining for each cycle.
var _requestAnimationFrame = function(win, t) { return win["webkitR" + t] || win["r" + t] || win["mozR" + t] || win["msR" + t] || function(fn) { setTimeout(fn, 60) } }(window, "equestAnimationFrame");
Note that we are using new Date()
to get the current time in milliseconds. The plus sign casts the date object to a numeric data type. rate
The variable is a number between 0 and 1, indicating the progress rate of the animation.
Anime function improvement
Now we need to consider the input and output of the function. Let's allow the function to accept the function and duration as parameters.
function animate() { var step = function() { _requestAnimationFrame(step); } step(); }
We can call this function like this:
function animate() { var duration = 1000 * 3, // 3 秒 end = +new Date() + duration; var step = function() { var current = +new Date(), remaining = end - current; if (remaining < 60) { // 如果剩余时间少于 60 毫秒,则在此结束动画 return; } else { var rate = 1 - remaining / duration; // 执行一些动画操作 } _requestAnimationFrame(step); } step(); }
In the run
function, I will place some code that animates the width of the node from "100px" to "300px".
function animate(item) { var duration = 1000 * item.time, end = +new Date() + duration; var step = function() { var current = +new Date(), remaining = end - current; if (remaining < 60) { item.run(1); // 1 = 进度为 100% return; } else { var rate = 1 - remaining / duration; item.run(rate); } _requestAnimationFrame(step); } step(); }
Improved animation function
It works fine, but what I really want is to be able to enter an array of functions that are called in sequence. This way, after the first animation is over, the second animation will begin. We will treat the array as a stack, popping items one by one at a time. Let's change the input:
animate({ time: 3, // 以秒为单位的时间 run: function(rate) { /* 使用 rate 执行某些操作 */ } });
When the animation is first run, item
is null, remaining
is less than 60 milliseconds, so we pop the first item from the array and start executing it. In the last frame of the animation, remaining
is also less than 60, so we complete the current animation, pop up the next item from the array and start animate the next item. Also note that I have passed the rate
value through the easing formula. The values from 0 to 1 now grow in a cubic proportion, making it look less stiff. To call the animation function, we do the following:
animate({ time: 3, run: function(rate) { document.getElementById("box").style .width = (rate * (300 - 100) + 100) + "px"; } });
Please note that the width of the box first expands, taking up 2 seconds, then height expands, and taking up 2 seconds.
Code optimization
Let's clean up our code a little. Please note that we call getElementById
several times, which is not very good. Let's cache it and cache it while cache the start and end values.
function animate(list) { var item, duration, end = 0; var step = function() { var current = +new Date(), remaining = end - current; if (remaining < 60) { if (item) item.run(1); // 1 = 进度为 100% item = list.shift(); // 获取下一个项目 if (item) { duration = item.time * 1000; end = current + duration; item.run(0); // 0 = 进度为 0% } else { return; } } else { var rate = remaining / duration; rate = 1 - Math.pow(rate, 3); // 缓动公式 item.run(rate); } _requestAnimationFrame(step); }; step(); }
Note that we do not need to modify the main function, because the run
function is always part of the self-contained object and can access all properties of the object through the this
variable. Now, whenever we run the step function, we cache all the variables. That's it. A simple animation helper function that takes advantage of requestAnimationFrame
and provides fallbacks for older browsers.
(The script demo part is omitted because JavaScript code cannot be run in this environment.)
(The FAQ section on using requestAnimationFrame for simple animation is also omitted, because the content is highly duplicated from the original text, and you only need to keep the core points to complete the pseudo-original.)
The above is the detailed content of Simple Animations Using requestAnimationFrame. For more information, please follow other related articles on the PHP Chinese website!
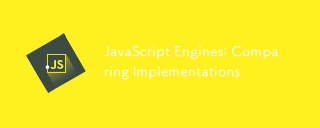
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
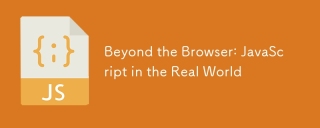
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
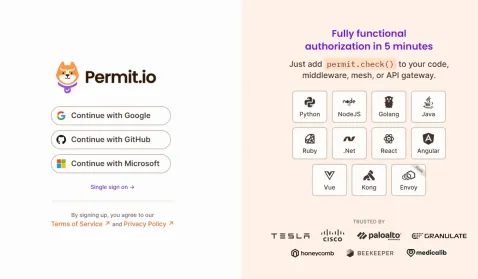
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
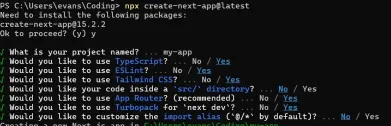
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
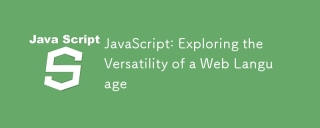
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
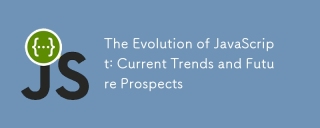
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
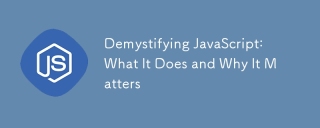
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
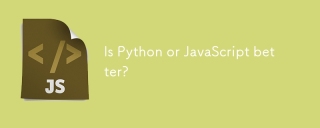
Python is more suitable for data science and machine learning, while JavaScript is more suitable for front-end and full-stack development. 1. Python is known for its concise syntax and rich library ecosystem, and is suitable for data analysis and web development. 2. JavaScript is the core of front-end development. Node.js supports server-side programming and is suitable for full-stack development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
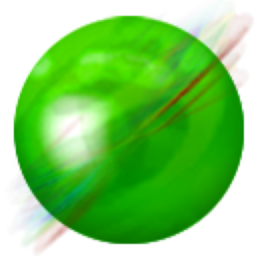
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
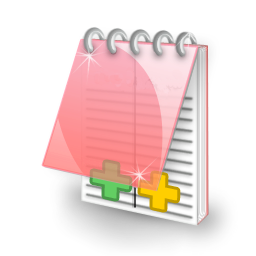
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment