This tutorial demonstrates how to create custom validation rules for your forms using the jQuery.validate.js plugin, expanding upon a previous guide on setting up form validation. We'll leverage the $.validator.addMethod()
function to define these rules. A live demo is provided, and the examples include handling date of birth validation. Note: A patched version addressing cross-browser compatibility and recursion issues is included.
Examples:
The following examples illustrate custom validation rules ensuring that both a name and email field are populated. If one is filled, the other must be as well.
-
Left Field Validation:
-
Right Field Validation:
-
Both Fields in Action (Multiple Pairs):
Live Demo:
(Edit on jsfiddle)
JQuery Code (Patched Version):
This improved snippet resolves cross-browser inconsistencies and recursion problems:
// Custom validation: each friend entered must have an email and a name $.validator.addMethod("fieldPresent", function(value, element, options) { var targetEl = $('input[name="' + options.data + '"]'), targetEmpty = (targetEl.val() == ''), elEmpty = (value == ''), bothEmpty = elEmpty && targetEmpty; if (!bothEmpty && targetEmpty) { setTimeout(function() { if (targetEl.closest('.control-group-inner').find('label.fieldPresentError').length == 0) { targetEl.addClass('error'); if (!elEmpty) $(element).closest('.control-group-inner').find('label.fieldPresentError').remove(); targetEl.closest('.control-group-inner').find('label.fieldPresentError').remove(); targetEl.after("<label class='fieldPresentError'>Friend's name and email required.</label>"); } }, 500); } return (bothEmpty || !elEmpty); }, "Friend's name and email required."); $('#myForm').validate({ onkeyup: true, rules: { "friend1-name": { "fieldPresent": { data: "friend1-email" } }, "friend1-email": { "fieldPresent": { data: "friend1-name" } } }, submitHandler: function(form) { console.log('passed validation.'); //submit form handler } });
HTML:
<form id="myForm"> <div class="control-group"> <div class="control-group-inner"> <label for="friend1-name">Friend's name</label> <input type="text" name="friend1-name" /> </div> <div class="control-group-inner"> <label for="friend1-email">Email Address</label> <input type="email" name="friend1-email" /> </div> </div> <button type="submit">Submit</button> </form>
CSS:
.control-group { width: 100%; } .control-group-inner { width: 50%; float: left; display: inline-block; } .error { border: 1px solid red; } label.fieldPresentError { color: red; display: block; margin-top: 5px; }
Date of Birth Validation (3 Inputs):
This section shows how to validate a date of birth using three separate fields (day, month, year):
// Custom validation for dob $.validator.addMethod("dobValid", function(value, element, options) { var day = $('input[name="dob-day"]'), month = $('input[name="dob-month"]'), year = $('input[name="dob-year"]'), anyEmpty = (day.val() == '' || month.val() == '' || year.val() == ''); if (anyEmpty) { day.add(month).add(year).addClass('error'); } else { day.add(month).add(year).removeClass('error'); } return !anyEmpty; }, "Please enter your date of birth."); // ... (rest of your validation code, including the rules for dob-day, dob-month, dob-year)
Remember to include the necessary <script></script>
tags for jQuery and the jQuery Validate plugin in your HTML file. This enhanced tutorial provides a more robust and reliable solution for custom form validation.
The above is the detailed content of jQuery Custom Validation Rule - fieldPresent. For more information, please follow other related articles on the PHP Chinese website!
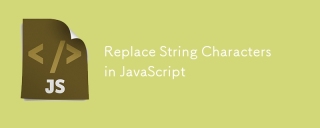
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
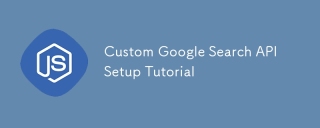
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
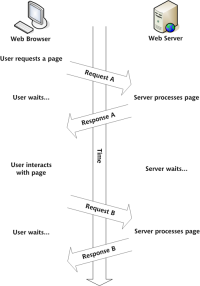
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
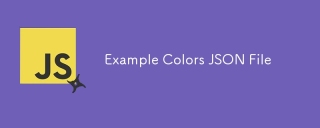
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
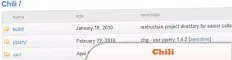
Enhance Your Code Presentation: 10 Syntax Highlighters for Developers Sharing code snippets on your website or blog is a common practice for developers. Choosing the right syntax highlighter can significantly improve readability and visual appeal. T
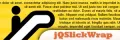
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
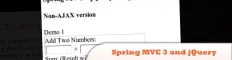
This article presents a curated selection of over 10 tutorials on JavaScript and jQuery Model-View-Controller (MVC) frameworks, perfect for boosting your web development skills in the new year. These tutorials cover a range of topics, from foundatio
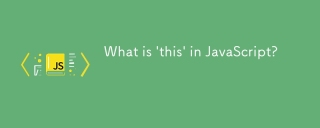
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
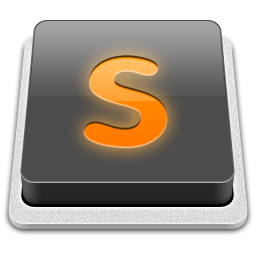
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
