Key Advantages of Using Gulp.js with Ember.js
This article demonstrates how Gulp.js can significantly enhance your Ember.js development workflow by automating repetitive tasks. These include SCSS compilation, JavaScript and CSS minification and concatenation, file monitoring, Handlebars template compilation, and JavaScript code optimization. We'll explore the setup and configuration of essential Gulp.js plugins to achieve this.
This guide assumes familiarity with Gulp.js. If you need a refresher, refer to SitePoint's Introduction to Gulp.js.
Standard Ember.js Development Challenges
Ember.js projects often involve managing SCSS, CSS, JavaScript, and Handlebars files. Let's examine the common tasks:
- SCSS to CSS Conversion: Transforming SCSS (Sassy CSS) preprocessor code into standard CSS.
- Minification: Reducing file sizes by removing unnecessary whitespace and comments in JavaScript and CSS.
- Concatenation: Combining multiple files into a single file to minimize HTTP requests, improving load times, especially on mobile devices.
- File Monitoring: Automating the build process by automatically triggering tasks whenever files are modified.
- Handlebars Compilation: Converting Handlebars templates into JavaScript functions for use by the Ember.js runtime.
- JavaScript Optimization: Minimizing JavaScript code size and improving performance through techniques like minification and renaming variables.
Essential Gulp.js Plugins
We'll utilize the following plugins:
-
gulp
: The core Gulp.js package. -
gulp-compass
: For SCSS compilation (requires Ruby and the Compass gem). -
gulp-uglify
: For JavaScript minification and optimization. -
gulp-watch
: For file monitoring and triggering tasks on changes. -
gulp-concat
: For concatenating CSS and JavaScript files. -
gulp-ember-handlebars
: For compiling Handlebars templates.
Plugin Installation
- Create a
package.json
file (if one doesn't exist). - Install Gulp globally:
npm install gulp -g
- Install plugins locally:
npm install gulp gulp-compass gulp-uglify gulp-watch gulp-concat gulp-ember-handlebars --save-dev
Your package.json
should now list these plugins under devDependencies
.
Gulpfile.js Configuration
Create a gulpfile.js
file and add the following code to import the plugins:
var gulp = require('gulp'), compass = require('gulp-compass'), watch = require('gulp-watch'), handlebars = require('gulp-ember-handlebars'), uglify = require('gulp-uglify'), concat = require('gulp-concat');
Defining Gulp Tasks
Let's define tasks for common operations. Remember that file paths are relative to gulpfile.js
.
-
CSS Task: Compiles SCSS, concatenates, and outputs to
dist/css
.
gulp.task('css', function() { return gulp.src('scss/*.scss') .pipe(compass({ sass: 'scss' })) .pipe(concat('main.min.css')) .pipe(gulp.dest('dist/css')); });
-
Templates Task: Compiles Handlebars templates and outputs to
js/
.
gulp.task('templates', function() { gulp.src(['js/templates/**/*.hbs']) .pipe(handlebars({ outputType: 'browser', namespace: 'Ember.TEMPLATES' })) .pipe(concat('templates.js')) .pipe(gulp.dest('js/')); });
-
Scripts Task: Uglifies, concatenates, and outputs JavaScript to
dist/js
.
gulp.task('scripts', function() { // ... (Your JavaScript file list here) ... return gulp.src(scriptSrc) .pipe(uglify({ mangle: false })) .pipe(concat('main.min.js')) .pipe(gulp.dest('dist/js')); });
- Watch Task: Monitors files and triggers relevant tasks on changes.
gulp.task('watch', function() { gulp.watch('scss/*.scss', ['css']); gulp.watch('js/templates/**/*.hbs', ['templates']); gulp.watch('js/**/*.js', ['scripts']); });
Utilizing the Tasks
-
Development: Run
gulp
(orgulp default
) to start the watch task and automatically rebuild on file changes. You might want to adjust theuglify
settings for development to avoid unnecessary processing.
gulp.task('default', ['css', 'templates', 'scripts', 'watch']);
-
Production: Run
gulp production
for a single build without the watch task.
gulp.task('production', ['css', 'templates', 'scripts']);
Remember to replace the placeholder JavaScript file list in the scripts
task with your actual project's files. Consult the documentation for each plugin for advanced customization options. This setup provides a robust and efficient workflow for your Ember.js projects.
The above is the detailed content of Improving Your Ember.js Workflow Using Gulp.js. For more information, please follow other related articles on the PHP Chinese website!
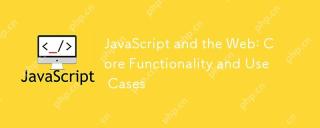
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
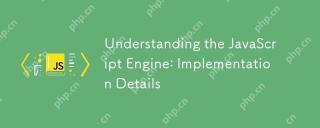
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
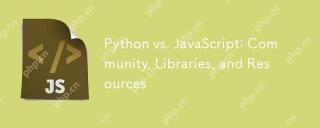
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
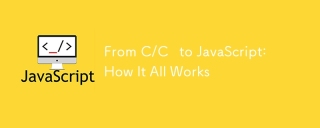
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
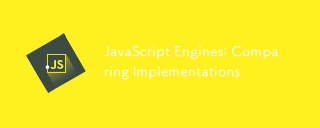
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
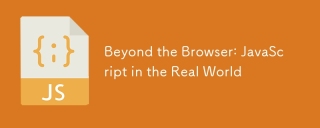
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
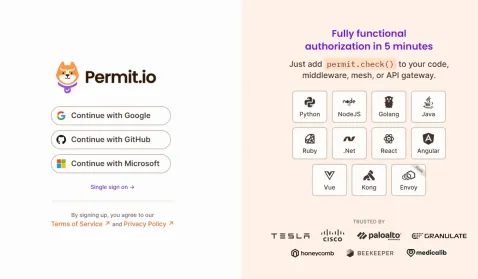
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
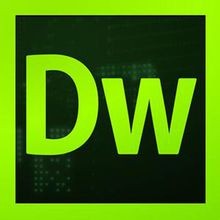
Dreamweaver CS6
Visual web development tools