WordPress 3.0 ushered in significant updates, including the integration of WordPress MU (enabling multi-site management) and the introduction of custom post types. A particularly useful feature enhanced by custom post types is the Custom Write Panel.
Custom Write Panels provide customizable form fields (text inputs, checkboxes, etc.) within the post editor, linked to custom fields. While the default Custom Field Panel is available, it can be cumbersome for extensive data entry. Custom Write Panels offer a streamlined, visually appealing alternative.
Let's illustrate with a "Books" custom post type. Beyond the standard title and content, we'll add "Author" and "ISBN" fields. In your theme's functions.php
, add this code to register the custom post type:
add_action( 'init', 'create_book_type' ); function create_book_type() { register_post_type( 'books', array( 'labels' => array( 'name' => __( 'Books' ), 'singular_name' => __( 'Book' ) ), 'public' => true, ) ); }
This registers the "Books" post type. Next, add the following to functions.php
to create the Custom Write Panel:
// Define paths (adjust as needed) define( 'MY_WORDPRESS_FOLDER', $_SERVER['DOCUMENT_ROOT'] ); define( 'MY_THEME_FOLDER', str_replace("\",'/',dirname(__FILE__)) ); define( 'MY_THEME_PATH', '/' . substr( MY_THEME_FOLDER, stripos(MY_THEME_FOLDER,'wp-content') ) ); add_action('admin_init','book_meta_init'); function book_meta_init() { wp_enqueue_style( 'my_meta_css', MY_THEME_PATH . '/custom/book_panel.css' ); add_meta_box( 'book_meta', 'Book Information', 'book_meta', 'books', 'advanced', 'high' ); } function book_meta() { global $post; $author = get_post_meta($post->ID,'author',TRUE); $isbn = get_post_meta($post->ID,'isbn',TRUE); include(MY_THEME_FOLDER . '/custom/book_information.php'); wp_nonce_field( __FILE__, 'my_meta_noncename' ); } function my_meta_save($post_id) { if (!wp_verify_nonce( $_POST['my_meta_noncename'], __FILE__ )) return $post_id; if (!current_user_can('edit_post', $post_id)) return $post_id; $accepted_fields['books'] = array( 'author', 'isbn' ); $post_type_id = $_POST['post_type']; foreach ($accepted_fields[$post_type_id] as $key) { $custom_field = $_POST[$key]; if (is_null($custom_field)) delete_post_meta($post_id, $key); elseif (isset($custom_field) && !is_null($custom_field)) update_post_meta($post_id,$key,$custom_field); } return $post_id; } add_action('save_post','my_meta_save');
Create a custom
directory in your theme and add book_panel.css
(for styling) and book_information.php
(for the panel HTML).
book_panel.css
:
.book_panel .description { display: none; } .book_panel label { display: block; font-weight: bold; margin: 6px; margin-bottom: 0; margin-top: 12px; } .book_panel label span { display: inline; font-weight: normal; } .book_panel span { color: #999; display: block; } .book_panel textarea, .book_panel input[type='text'] { margin-bottom: 3px; width: 100%; } .book_panel h4 { color: #999; font-size: 1em; margin: 15px 6px; text-transform:uppercase; }
book_information.php
:
<div class="book_panel"> <h4 id="Book-Details">Book Details</h4> <label for="author">Author <span>(Required)</span></label> <input type="text" name="author" id="author" value="<?php echo esc_attr( $author ); ?>" /><br/> <label for="isbn">ISBN <span>(Required)</span></label> <input type="text" name="isbn" id="isbn" value="<?php echo esc_attr( $isbn ); ?>" /> </div>
Finally, to display the custom fields in your theme, use get_post_meta()
within your loop:
$loop = new WP_Query( array( 'post_type' => 'books', 'posts_per_page' => 10 ) ); while ($loop->have_posts()) : $loop->the_post(); the_title(); the_content(); echo 'Author: ' . get_post_meta(get_the_ID(), "author", true); echo 'ISBN: ' . get_post_meta(get_the_ID(), "isbn", true); endwhile;
This completes the setup. Remember to adjust paths in the code to match your theme's structure. For more in-depth WordPress knowledge, consider our publication, "Build Your Own Wicked WordPress Themes."
The above is the detailed content of Guide to Wordpress's Custom Write Panels. For more information, please follow other related articles on the PHP Chinese website!

TosecureaWordPresssite,followthesesteps:1)RegularlyupdateWordPresscore,themes,andpluginstopatchvulnerabilities.2)Usestrong,uniquepasswordsandenabletwo-factorauthentication.3)OptformanagedWordPresshostingorsecuresharedhostingwithawebapplicationfirewal

WordPressexcelsoverotherwebsitebuildersduetoitsflexibility,scalability,andopen-sourcenature.1)It'saversatileCMSwithextensivecustomizationoptionsviathemesandplugins.2)Itslearningcurveissteeperbutofferspowerfulcontroloncemastered.3)Performancecanbeopti
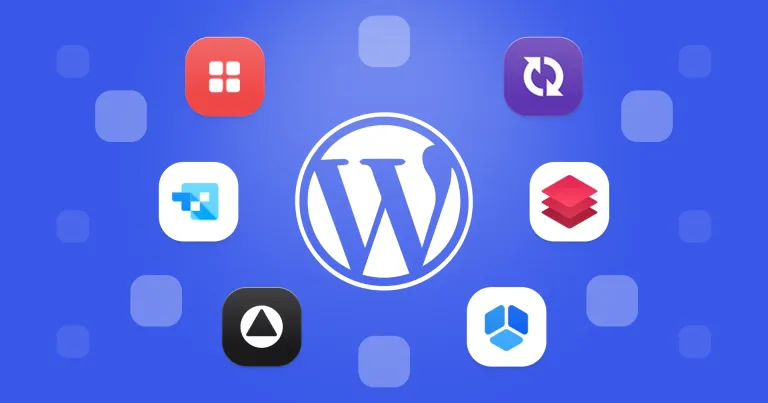
Seven Must-Have WordPress Plugins for 2025 Website Development Building a top-tier WordPress website in 2025 demands speed, responsiveness, and scalability. Achieving this efficiently often hinges on strategic plugin selection. This article highlig

WordPresscanbeusedforvariouspurposesbeyondblogging.1)E-commerce:WithWooCommerce,itcanbecomeafullonlinestore.2)Membershipsites:PluginslikeMemberPressenableexclusivecontentareas.3)Portfoliosites:ThemeslikeAstraallowstunninglayouts.Ensuretomanageplugins

Yes,WordPressisexcellentforcreatingaportfoliowebsite.1)Itoffersnumerousportfolio-specificthemeslike'Astra'foreasycustomization.2)Pluginssuchas'Elementor'enableintuitivedesign,thoughtoomanycanslowthesite.3)SEOisenhancedwithtoolslike'YoastSEO',boosting

WordPressisadvantageousovercodingawebsitefromscratchdueto:1)easeofuseandfasterdevelopment,2)flexibilityandscalability,3)strongcommunitysupport,4)built-inSEOandmarketingtools,5)cost-effectiveness,and6)regularsecurityupdates.Thesefeaturesallowforquicke

WordPressisaCMSduetoitseaseofuse,customization,usermanagement,SEO,andcommunitysupport.1)Itsimplifiescontentmanagementwithanintuitiveinterface.2)Offersextensivecustomizationthroughthemesandplugins.3)Providesrobustuserrolesandpermissions.4)EnhancesSEOa
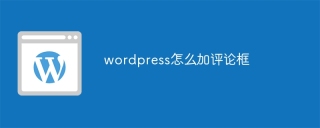
Enable comments on your WordPress website to provide visitors with a platform to participate in discussions and share feedback. To do this, follow these steps: Enable Comments: In the dashboard, navigate to Settings > Discussions, and select the Allow Comments check box. Create a comment form: In the editor, click Add Block and search for the Comments block to add it to the content. Custom Comment Form: Customize comment blocks by setting titles, labels, placeholders, and button text. Save changes: Click Update to save the comment box and add it to the page or article.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
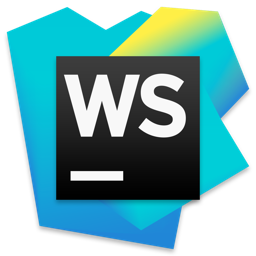
WebStorm Mac version
Useful JavaScript development tools
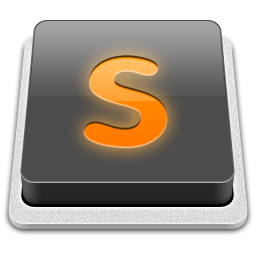
SublimeText3 Mac version
God-level code editing software (SublimeText3)
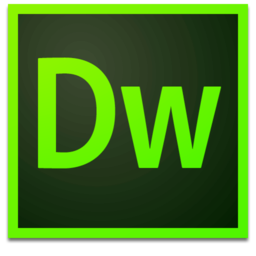
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor
