Key Points
- Do not include manifest files in the application cache manifest, which can create a loop that will almost no longer inform your website that new cache files are available.
- Always set the application type manifest in the server's .htaccess file to ensure AppCache is running correctly. If the media type is not set, AppCache will not work.
- Note that if none of the individual files specified in the manifest file can be found or cannot be downloaded, the entire manifest file will be discarded. This is a special behavior of AppCache.
- After updating the website, always update the manifest file, otherwise the user will not see the changes and will only see the previously cached data. You can update the version number or date in the comments in the manifest file to force the user's web browser to download a new version of the manifest file.
HTML5 application caching (also known as AppCache) has become a hot topic for web developers recently. AppCache allows you to allow website visitors to browse your website while offline. You can even store parts of the website, such as images, style sheets, or web fonts, in a cache on the user's computer. This can help your website load faster, thereby reducing the load on the server.
To use AppCache, you can create a manifest file with the extension "appcache", for example: manifest.appcache. In this file, you can list all files to cache. To enable it on your site, you must include a reference to the manifest file on the webpage of the html element, as shown below:
<html lang="en" manifest="manifest.appcache">
This is a sample manifest file:
<code>CACHE MANIFEST # 2015-01-23 v0.1 /style.css /logo.gif /script.js NETWORK: * FALLBACK: /server/ /fallback.html</code>
In addition to the benefits of AppCache, there are some common pitfalls that should be avoided to prevent disrupting the user experience and destroying your app.
Do not list manifest files in manifest files
If you include the manifest file itself in the application cache manifest, it will get stuck in some sort of loop that will hardly inform your website that there is a new cache file available, and it should download and use the new manifest file instead of the old one manifest file. Therefore, be careful not to make the following mistakes:
<code>CACHE MANIFEST # 2015-01-23 v0.1 manifest.appcache page2.css</code>
Uncacheable resources on the cache page will not load
This is a very common mistake when using AppCache for the first time. This is where the NETWORK flag in the manifest file comes into play. The NETWORK section of the manifest file specifies the resources that the web application needs to access online.
The URL specified under the NETWORK flag is basically a "whitelist", that is, the file specified under this flag is always loaded from the server when there is an Internet connection. For example, the following code snippet ensures that requests to load resources contained in the /api/ subtree are always loaded from the network, not from the cache.
<html lang="en" manifest="manifest.appcache">
Always set the application type manifest in the server's .htaccess file
The manifest file should always be served under the correct media type text/cache-manifest. If the media type is not set, AppCache will not work.
It should always be configured in the .htaccess file of the production server. This is mentioned in most tutorials explaining AppCache, but many developers ignore this when migrating web applications from development servers to production servers.
Enter the following in Apache's .htaccess file:
<code>CACHE MANIFEST # 2015-01-23 v0.1 /style.css /logo.gif /script.js NETWORK: * FALLBACK: /server/ /fallback.html</code>
If you upload your app to Google App Engine, you can do the same task by adding the following snippet to the app.yaml file:
<code>CACHE MANIFEST # 2015-01-23 v0.1 manifest.appcache page2.css</code>
Avoid discarding the entire list because the file cannot be found
If none of the individual files specified in the manifest file can be found or cannot be downloaded, the entire manifest file will be discarded. This is a strange behavior of AppCache, which should be kept in mind when designing web applications that use AppCache.
Example:
<code>NETWORK: /api</code>
If you delete the logo.gif, AppCache will not be able to find the deleted image file, so nothing in the manifest file will be executed.
Data is loaded from AppCache even if online
Once your web browser saves the cache manifest file, the file will be loaded from the cache manifest itself even if the user is connected to the internet. This feature helps to increase the loading speed of your website and helps reduce server load.
The changes on the server do not occur until the manifest file is updated
Because you knew from the previous point that data would load from AppCache even if the user is online, changes to files in the website or server will not occur until the manifest file is updated.
After updating the website, you always have to update the manifest file, otherwise your users will never see the changes, they will only see the data that was previously cached. You can update the version number or date in the comments in the manifest file to force the user's web browser to download a new version of the manifest file. For example, if you are using a list file before making changes to your website:
<code>AddType text/cache-manifest .manifest</code>
It can be changed to something similar to the following code block so that the user's browser can download a new copy of the manifest file.
<code>- url: /public_html/(.*\.appcache) static_files: public_html/ mime_type: text/cache-manifest upload: public_html/(.*\.appcache)</code>
Please note that lines starting with # are comment lines that will not be executed.
The manifest file must be served from the same source as the host.
Although the manifest file can hold a reference to the resource to be cached from other domains, it should be provided to the web browser from the same source as the host page. If this is not the case, the manifest file will not be loaded. For example, the following manifest file is correct:
<code>CACHE MANIFEST # 2015-01-23 v0.1 /style.css /logo.gif /script.js</code>
Here we specify what we want to store in the user's browser cache, which is referenced from another domain, which is absolutely fine.
Relative URL Relative to list URL
One important thing to note is that the relative URL you mentioned in the manifest is relative to the manifest file, not relative to the document you reference the manifest file. If the manifest and reference are not in the same path, the resource will not be loaded and the manifest file will not be loaded.
If your application structure looks like this:
<html lang="en" manifest="manifest.appcache">
Then your manifest file should look like this:
<code>CACHE MANIFEST # 2015-01-23 v0.1 /style.css /logo.gif /script.js NETWORK: * FALLBACK: /server/ /fallback.html</code>
Check the status of the checklist programmatically
You can programmatically check if your application is using an updated version of the cache manifest by testing window.applicationCache.status. Here are some sample code:
<code>CACHE MANIFEST # 2015-01-23 v0.1 manifest.appcache page2.css</code>
Running the above code on the website will let you know when new updates to the AppCache list are available. Note that UPDATEREADY is a defined state. You can even use the swapCache() method in the onUpdateReady() function to replace the old manifest file with the new manifest file:
<code>NETWORK: /api</code>
Conclusion
AppCache is a useful technique, but as we have seen, be cautious when implementing it in a project. Developers should selectively select what should be included in the manifest file. Ideally, the manifest file should contain static content such as stylesheets, scripts, web fonts, and images. However, you are always the best judge of what is included in the manifest file. Appcache is a double-edged sword, so be careful when using it!
Most of the above content has been introduced elsewhere, and there are some other key points. You can check out the following resources for more information:
- Application Cache Trap on MDN
- Jake Archibald's app cache is a jerk
- Jake Archibald's Offline Recipes
FAQs about HTML5 Application Caching (FAQ)
What is HTML5 application caching and why is it important?
HTML5 Application Cache (AppCache) is a feature that allows developers to specify which files should be cached by the browser and make them available for users to offline. It is important because it can improve the performance of your web application by reducing server load and saving bandwidth. It also allows applications to run even when users are offline, providing a better user experience.
How does HTML5 application caching work?
HTML5 Application Caching works by using manifest files. This file lists the resources that the browser should cache for offline use. When a user accesses a web page, the browser checks whether the manifest file is associated with it. If so, the browser will download and store the listed resources. The next time a user visits a web page, the browser will load the cached resources instead of downloading them from the server.
What are the common pitfalls when using HTML5 application caching?
When using HTML5 application caching, some common pitfalls include: not updating the manifest file correctly, causing old resources to be provided; not handling the cached manifest fallback part correctly, resulting in errors; and not considering the impact of cache on user device storage.
How to avoid these traps?
To avoid these pitfalls, always update the manifest file correctly when the resource changes. Use the NETWORK section of the manifest file to specify resources that should never be cached. Also, consider the user's device storage and cache only the necessary resources.
What is the future of HTML5 application caching?
HTML5 application cache is being deprecated, replaced by Service Workers. Service Workers provide more control over caches and can handle more complex scenarios. However, Service Workers are currently supported by not all browsers, so it is still important to understand and use HTML5 application caching.
How to create a manifest file?
The manifest file is a simple text file that lists the resources to be cached. It should be served as MIME type "text/cache-manifest". The first line of the file should be "CACHE MANIFEST", followed by the resource to be cached.
How to associate a web page with a manifest file?
To associate a web page with a manifest file, add the "manifest" attribute to the "html" tag of the web page. The value of the "manifest" attribute should be the URL of the manifest file.
How to update the cache?
To update the cache, make changes to the manifest file. Every time a user visits a web page, the browser checks for updates to the manifest file. If the manifest file has been changed, the browser will download and cache the new resource.
What happens if the resources listed in the manifest file cannot be downloaded?
If the resources listed in the manifest file cannot be downloaded, the entire cache update process will fail. The browser will continue to use the old cache.
Can I use HTML5 application cache for all resources?
While technically you can use HTML5 application cache for all resources, this is not recommended. Excessive cache of resources can fill up the user's device storage and negatively impact performance. It is best to cache only the necessary resources.
The above is the detailed content of Common Pitfalls to Avoid when using HTML5 Application Cache. For more information, please follow other related articles on the PHP Chinese website!
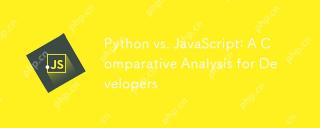
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
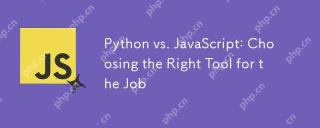
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
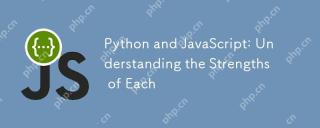
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
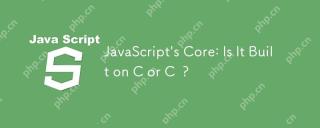
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
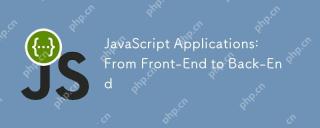
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
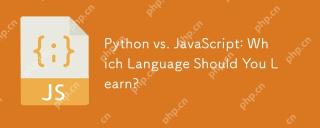
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
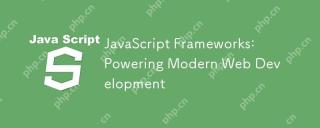
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
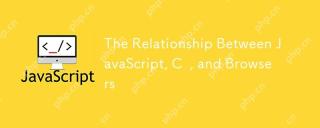
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
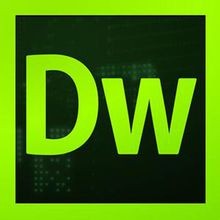
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
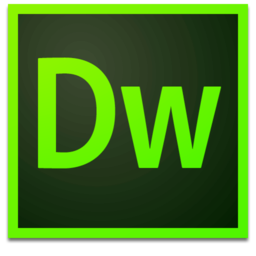
Dreamweaver Mac version
Visual web development tools
