ECMAScript 6 (ES6) Revolutionizes JavaScript Development: Simulating Classes and Inheritance
ES6 significantly enhances JavaScript, a prototype-based language, by providing class-like syntax and inheritance mechanisms. This empowers developers to build large-scale web applications more efficiently. Key improvements include stricter constructor invocation rules (requiring new
), non-enumerable methods, and streamlined inheritance.
The extends
keyword simplifies inheritance, allowing the creation of specialized child classes from parent classes. The super
keyword provides access to the parent class's methods and constructor, making inheritance straightforward.
TypeScript, a superset of JavaScript, offers a valuable pathway to understanding ES6. Its syntax closely mirrors ES6 (without the type annotations), making it an excellent tool for generating ES6-compliant JavaScript code.
Creating Classes in ES6
JavaScript's prototype-based nature allows for class simulation in ES5, but ES6 simplifies this considerably:
ES5 (Simulation):
var Animal = (function () { function Animal(name) { this.name = name; } Animal.prototype.doSomething = function () { console.log("I'm a " + this.name); }; return Animal; })();
ES6 (Native Classes):
class AnimalES6 { constructor(name) { this.name = name; } doSomething() { console.log("I'm a " + this.name); } }
ES6 classes offer improved readability and enforce stricter semantics. Methods are non-enumerable, and constructors must be called with new
.
Getters and Setters, and Enhanced Privacy with Symbols
ES6 supports getters and setters, enhancing code clarity and control over property access:
class AnimalES6 { constructor(name) { this.name = name; this[ageSymbol] = 0; // Using Symbol for (near) private member } get age() { return this[ageSymbol]; } set age(value) { if (value < 0) console.log("Invalid age"); this[ageSymbol] = value; } // ... }
The use of Symbol
creates a unique identifier, providing a degree of data hiding (though not absolute privacy).
Inheritance in ES6
ES6 elegantly handles inheritance using extends
and super
:
class InsectES6 extends AnimalES6 { constructor(name) { super(name); this[legsCountSymbol] = 6; //Using Symbol for (near) private member } // ... }
This approach is far more intuitive and readable than ES5's prototype-based inheritance simulation.
TypeScript's Role in ES6 Development
TypeScript's close resemblance to ES6 makes it an ideal tool for learning and developing ES6 code. It allows for type checking and improved code maintainability, ultimately generating clean ES6 (or ES5) JavaScript.
Conclusion
ES6 brings significant improvements to JavaScript, making it a more powerful and developer-friendly language for building complex web applications. The introduction of classes, enhanced inheritance, and features like Symbols and improved scoping contribute to cleaner, more maintainable code. TypeScript further enhances the development process by providing additional tooling and type safety.
The above is the detailed content of Understanding ECMAScript 6: Class and Inheritance. For more information, please follow other related articles on the PHP Chinese website!
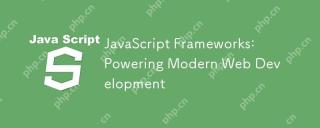
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
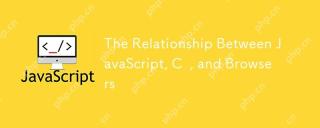
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
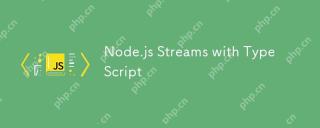
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
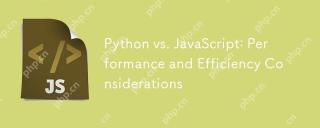
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
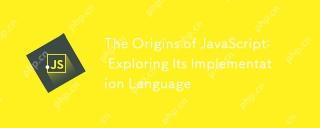
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
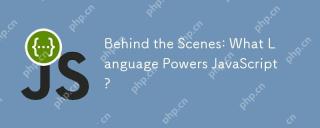
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
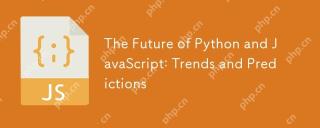
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
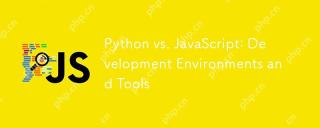
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
