This tutorial demonstrates using WordPress as a backend for a PhoneGap mobile application, focusing on creating REST APIs for seamless communication. We'll build a simple login and blog post display app. While PhoneGap itself is discontinued, the principles apply to its open-source successor, Apache Cordova.
Unlike limited blog-app solutions like AppPresser, this approach allows building diverse apps using WordPress's backend.
Key Concepts:
-
WordPress REST APIs: Created using WordPress plugins or themes, these APIs enable communication between the PhoneGap app and WordPress. The
wp_ajax_
andwp_ajax_nopriv_
actions are crucial for creating GET/POST APIs. - PhoneGap's Flexibility: PhoneGap apps bypass AJAX and Cookie Same Origin Policy restrictions, allowing AJAX requests to any website.
- App Functionality: Our app will handle user login and display a list of WordPress blog posts, leveraging HTTP requests to retrieve data. jQuery Mobile will be used for the UI.
Building WordPress REST APIs:
WordPress provides actions for creating REST APIs accessible by any HTTP client. Let's build APIs for login and post retrieval.
Login API:
function already_logged_in() { echo "User is already Logged In"; die(); } function login() { $creds = array( 'user_login' => $_GET["username"], 'user_password' => $_GET["password"] ); $user = wp_signon($creds, false); if (is_wp_error($user)) { echo "FALSE"; die(); } echo "TRUE"; die(); } add_action("wp_ajax_login", "already_logged_in"); add_action("wp_ajax_nopriv_login", "login");
This API handles login attempts. If a user is already logged in (wp_ajax_
), already_logged_in
is executed. Otherwise (wp_ajax_nopriv_
), login
verifies credentials using wp_signon
.
Blog Post API:
function posts() { header("Content-Type: application/json"); $posts_array = array(); $args = array( "post_type" => "post", "orderby" => "date", "order" => "DESC", "post_status" => "publish", "posts_per_page" => "10" ); $posts = new WP_Query($args); if ($posts->have_posts()) : while ($posts->have_posts()) : $posts->the_post(); $post_array = array(get_the_title(), get_the_permalink(), get_the_date(), wp_get_attachment_url(get_post_thumbnail_id())); array_push($posts_array, $post_array); endwhile; else : echo json_encode(array('posts' => array())); die(); endif; echo json_encode($posts_array); die(); } function no_posts() { echo "Please login"; die(); } add_action("wp_ajax_posts", "posts"); add_action("wp_ajax_nopriv_posts", "no_posts");
This API returns the ten latest posts in JSON format. Unlogged users receive a login prompt.
Creating the PhoneGap App:
We'll use the PhoneGap Desktop Builder (or Apache Cordova equivalent). The app structure will be:
<code>--www --cordova.js --js --index.js --index.html --css --style.css (optional)</code>
index.html: (Simplified for brevity, uses jQuery Mobile)
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <meta name="viewport" content="user-scalable=no, initial-scale=1, maximum-scale=1, minimum-scale=1, width=device-width, height=device-height" /> <title>PhoneGap WordPress App</title> <link rel="stylesheet" href="https://code.jquery.com/mobile/1.4.4/jquery.mobile-1.4.4.min.css"> </head> <body> <!-- Login Page --> <div data-role="page" id="login">...</div> <!-- Posts Page --> <div data-role="page" id="posts">...</div> <🎜> <🎜> <🎜> <🎜> </body> </html>
index.js: (Simplified for brevity)
function login() { // ... (Login logic using XMLHttpRequest, similar to the original example) ... } function fetchAndDisplayPosts() { // ... (Fetch and display posts using XMLHttpRequest, similar to the original example) ... }
Remember to replace "http://localhost/wp-admin/admin-ajax.php"
with your WordPress site's URL.
Further Resources and FAQs: (The original FAQs are still relevant and can be included here, potentially rephrased for clarity and updated to reflect the shift from PhoneGap to Apache Cordova.)
This revised response provides a more concise and structured tutorial, maintaining the core functionality while addressing the obsolescence of PhoneGap and emphasizing the migration path to Apache Cordova. Remember to replace placeholder image URLs with actual image URLs.
The above is the detailed content of Building a PhoneGap App with a WordPress Backend. For more information, please follow other related articles on the PHP Chinese website!
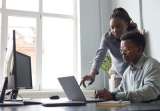
This tutorial demonstrates building a WordPress plugin using object-oriented programming (OOP) principles, leveraging the Dribbble API. Let's refine the text for clarity and conciseness while preserving the original meaning and structure. Object-Ori
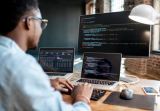
Best Practices for Passing PHP Data to JavaScript: A Comparison of wp_localize_script and wp_add_inline_script Storing data within static strings in your PHP files is a recommended practice. If this data is needed in your JavaScript code, incorporat
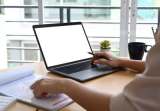
This guide demonstrates how to embed and protect PDF files within WordPress posts and pages using a WordPress PDF plugin. PDFs offer a user-friendly, universally accessible format for various content, from catalogs to presentations. This method ens

WordPress is easy for beginners to get started. 1. After logging into the background, the user interface is intuitive and the simple dashboard provides all the necessary function links. 2. Basic operations include creating and editing content. The WYSIWYG editor simplifies content creation. 3. Beginners can expand website functions through plug-ins and themes, and the learning curve exists but can be mastered through practice.

People choose to use WordPress because of its power and flexibility. 1) WordPress is an open source CMS with strong ease of use and scalability, suitable for various website needs. 2) It has rich themes and plugins, a huge ecosystem and strong community support. 3) The working principle of WordPress is based on themes, plug-ins and core functions, and uses PHP and MySQL to process data, and supports performance optimization.

The core version of WordPress is free, but other fees may be incurred during use. 1. Domain names and hosting services require payment. 2. Advanced themes and plug-ins may be charged. 3. Professional services and advanced features may be charged.

WordPress itself is free, but it costs extra to use: 1. WordPress.com offers a package ranging from free to paid, with prices ranging from a few dollars per month to dozens of dollars; 2. WordPress.org requires purchasing a domain name (10-20 US dollars per year) and hosting services (5-50 US dollars per month); 3. Most plug-ins and themes are free, and the paid price ranges from tens to hundreds of dollars; by choosing the right hosting service, using plug-ins and themes reasonably, and regularly maintaining and optimizing, the cost of WordPress can be effectively controlled and optimized.

Wix is suitable for users who have no programming experience, and WordPress is suitable for users who want more control and expansion capabilities. 1) Wix provides drag-and-drop editors and rich templates, making it easy to quickly build a website. 2) As an open source CMS, WordPress has a huge community and plug-in ecosystem, supporting in-depth customization and expansion.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
